可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I've seen there are actually two (maybe more) ways to concatenate lists in Python:
One way is to use the extend() method:
a = [1, 2]
b = [2, 3]
b.extend(a)
the other to use the plus(+) operator:
b += a
Now I wonder: Which of those two options is the 'pythonic' way to do list concatenation and is there a difference between the two (I've looked up the official Python tutorial but couldn't find anything anything about this topic).
回答1:
The only difference on a bytecode level is that the .extend
way involves a function call, which is slightly more expensive in Python than the INPLACE_ADD
.
It's really nothing you should be worrying about, unless you're performing this operation billions of times. It is likely, however, that the bottleneck would lie some place else.
回答2:
You can't use += for non-local variable (variable which is not local for function and also not global)
def main():
l = [1, 2, 3]
def foo():
l.extend([4])
def boo():
l += [5]
foo()
print l
boo() # this will fail
main()
It's because for extend case compiler will load the variable l
using LOAD_DEREF
instruction, but for += it will use LOAD_FAST
- and you get *UnboundLocalError: local variable 'l' referenced before assignment*
回答3:
You can chain function calls, but you can't += a function call directly:
class A:
def __init__(self):
self.listFoo = [1, 2]
self.listBar = [3, 4]
def get_list(self, which):
if which == "Foo":
return self.listFoo
return self.listBar
a = A()
other_list = [5, 6]
a.get_list("Foo").extend(other_list)
a.get_list("Foo") += other_list #SyntaxError: can't assign to function call
回答4:
According to the Zen of Python:
Simple is better than complex.
b += a
is more simple than b.extend(a)
.
The builtins are so highly optimized that there's no real performance difference.
回答5:
I would say that there is some difference when it comes with numpy (I just saw that the question ask about concatenating two lists, not numpy array, but since it might be a issue for beginner, such as me, I hope this can help someone who seek the solution to this post), for ex.
import numpy as np
a = np.zeros((4,4,4))
b = []
b += a
it will return with error
ValueError: operands could not be broadcast together with shapes (0,) (4,4,4)
b.extend(a)
works perfectly
回答6:
From python 3.5.2 source code:
No big difference.
static PyObject *
list_inplace_concat(PyListObject *self, PyObject *other)
{
PyObject *result;
result = listextend(self, other);
if (result == NULL)
return result;
Py_DECREF(result);
Py_INCREF(self);
return (PyObject *)self;
}
回答7:
According to the Python for Data Analysis.
“Note that list concatenation by addition is a comparatively expensive operation since a new list must be created and the objects copied over. Using extend to append elements to an existing list, especially if you are building up a large list, is usually preferable. ”
Thus,
everything = []
for chunk in list_of_lists:
everything.extend(chunk)
is faster than the concatenative alternative:
everything = []
for chunk in list_of_lists:
everything = everything + chunk
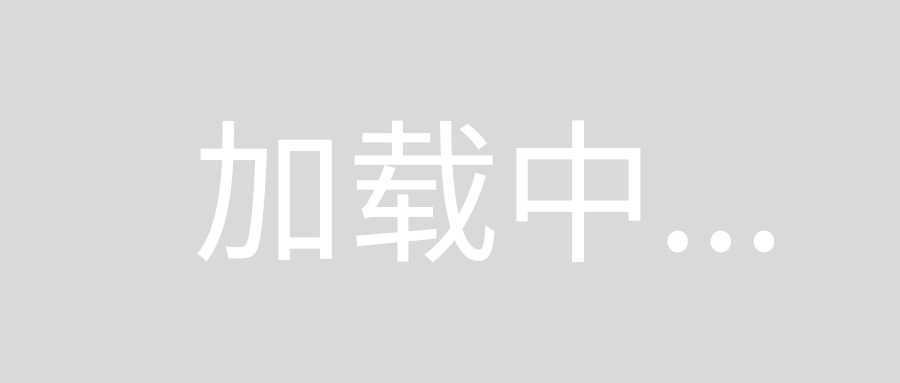