I usually use custom UIColors on iOS using extensions with Swift but now with iOS11/XCode9 we can create Colors Sets. How can we use them?
Update - Tip
As @Cœur says we can drag&drop de color, and use it like a UIColor object and a possible solution could be use it as a extension:
Or as a constant:
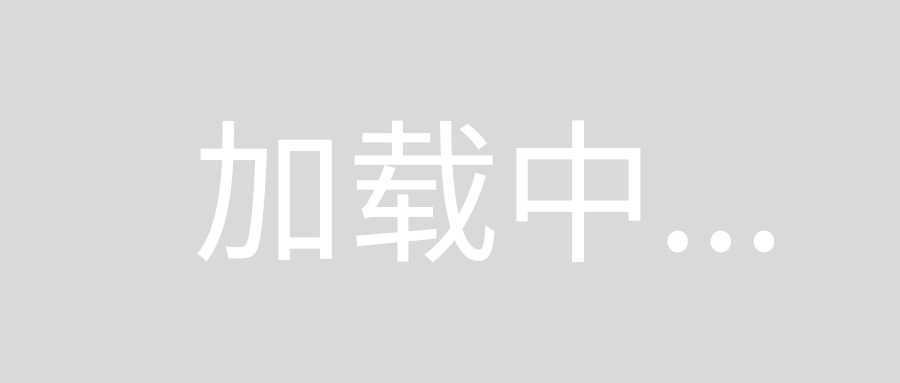
Now I wanna know if we can access them like an UIImage access to an Asset Image or not, like:
UIImage(named: "image-name") -> UIColor(named: "color-name")
UIColor(named: "myColor")
Source: WWDC 2017 Session 237 —— What's New in MapKit
Caveat: Your project's Deployment Target needs to be set to iOS 11.0.
(short answer to the question update: there is UIColor(named: "MyColor")
in Xcode 9.0)
Answering the original question:
- you create your color set
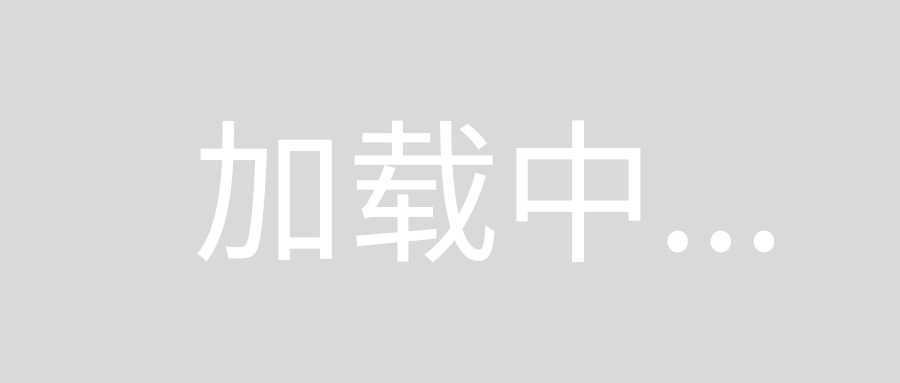
- you find your color among your snippets and you drag-n-drop it
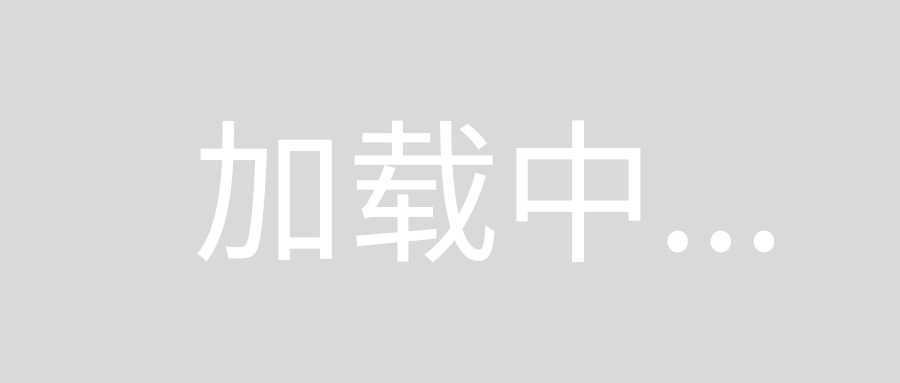
it will translate to a color literal when looking at the source code:
#colorLiteral(red: 0, green: 0.6378085017, blue: 0.8846047521, alpha: 1)
You notice how the values of red, green and blue are different? It's because I defined them using Color Space Display P3
, but the colorLiteral is using Color Space sRGB
.
Short Version
Add a colour set to an asset catalog, name it and set your colour in the attributes inspector, then call it in your code with UIColor(named: "MyColor")
.
Full Instructions
In the asset catalog viewer, click the plus button at the bottom right of the main panel and choose New Color Set
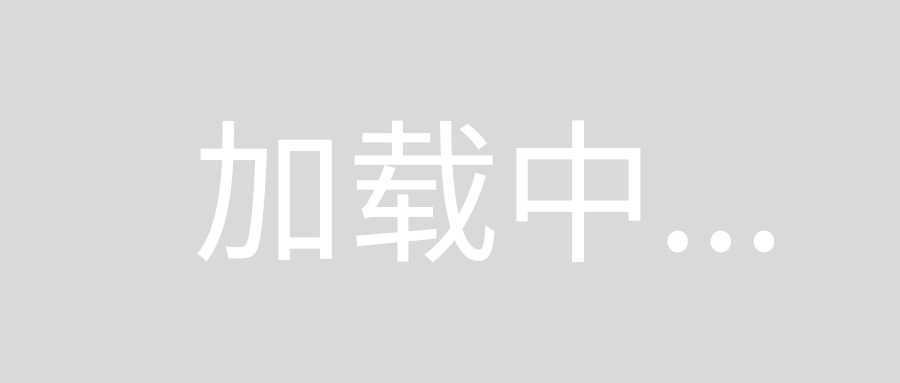
Click on the white square, and select the Attributes Inspector (right-most icon in the right pane)
From there you can name and choose your colour.
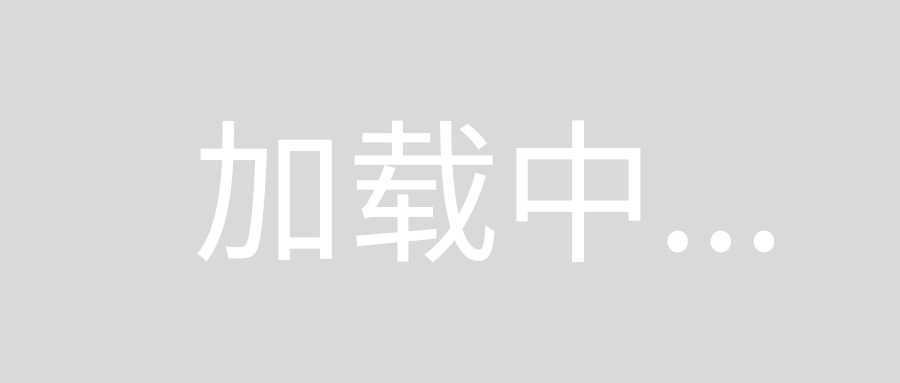
- To use it in your code, call it with
UIColor(named: "MyColor")
. This returns an optional, so you'll need to unwrap it in most cases (this is probably one of the few cases where a force unwrap is acceptable, given you know the colour exists in your asset catalog).
// iOS
let color = UIColor(named: "SillyBlue")
// macOS
let color = NSColor(named: "SillyBlue")
You need to use UIColor(named: "appBlue")
.
And you can create a function in UIColor extension for simple access.
enum AssetsColor {
case yellow
case black
case blue
case gray
case green
case lightGray
case seperatorColor
case rad
}
extension UIColor {
static func appColor(_ name: AssetsColor) -> UIColor? {
switch name {
case .yellow:
return UIColor(named: "appYellow")
case .black:
return UIColor(named: "appBlack")
case .blue:
return UIColor(named: "appBlue")
case .gray:
return UIColor(named: "appGray")
case .lightGray:
return UIColor(named: "appLightGray")
case .rad:
return UIColor(named: "appRad")
case .seperatorColor:
return UIColor(named: "appSeperatorColor")
case .green:
return UIColor(named: "appGreen")
}
}
You can use it like this
userNameTextField.textColor = UIColor.appColor(.gray)