I would like to display the default product attributes form value and it's regular price in my front end template file.
The var_dump
below shows options in an array. I Need to get the [default_attributes]
values.
<?php
global $product;
echo var_dump( $product );
// Need to get the [default_attributes] values
?>
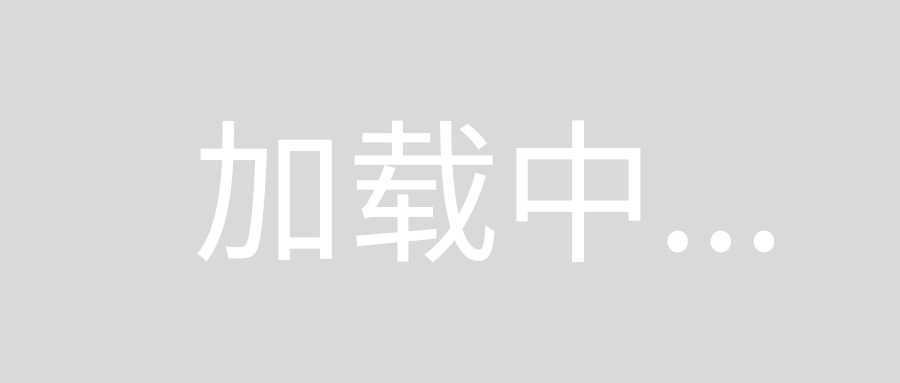
To get the default attributes for a variable product you can use WC_Product
method get_default_attributes()
this way:
<?php
global $product;
if( $product->is_type('variable') ){
$default_attributes = $product->get_default_attributes();
// Testing raw output
var_dump($default_attributes);
}
?>
Now to find out which is the corresponding product variation for this "defaults" attributes, is a little more complicated:
<?php
global $product;
if( $product->is_type('variable') ){
$default_attributes = $product->get_default_attributes();
foreach($product->get_available_variations() as $variation_values ){
foreach($variation_values['attributes'] as $key => $attribute_value ){
$attribute_name = str_replace( 'attribute_', '', $key );
$default_value = $product->get_variation_default_attribute($attribute_name);
if( $default_value == $attribute_value ){
$is_default_variation = true;
} else {
$is_default_variation = false;
break; // Stop this loop to start next main lopp
}
}
if( $is_default_variation ){
$variation_id = $variation_values['variation_id'];
break; // Stop the main loop
}
}
// Now we get the default variation data
if( $is_default_variation ){
// Raw output of available "default" variation details data
echo '<pre>'; print_r($variation_values); echo '</pre>';
// Get the "default" WC_Product_Variation object to use available methods
$default_variation = wc_get_product($variation_id);
// Get The active price
$price = $default_variation->get_price();
}
}
?>
This is tested and works.