可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
When you tap on a UIBarButtonItem
in a UIToolbar
, there is a white glow effect.
Is there a possibility to fire an event to show this effect?
I don't want to press the button. Only the effect should be displayed. I want to visualize to the user, that there is new content behind this button.
Thanks for your help!
回答1:
The following method assumes you are using the toolbarItems property of a navigation controller and that the button you want to highlight is the last item in that array. You can apply it to any toolbar, of course, and pick a different array index if necessary.
Right now this is not exactly perfect, because the animation moves the new button from the lower left of the screen. However, I think that can be turned off with a little effort.
Note that I used PeakJi's image.
I hope this works for you.
Enjoy,
Damien
- (void)highlightButton {
NSArray *originalFooterItems = self.toolbarItems;
NSMutableArray *footerItems = [originalFooterItems mutableCopy];
[footerItems removeLastObject];
NSString* pathToImageFile = [[NSBundle mainBundle] pathForResource:@"white_glow" ofType:@"png"];
UIImage* anImage = [UIImage imageWithContentsOfFile:pathToImageFile];
UIImageView *imageView = [[UIImageView alloc] initWithImage:anImage];
imageView.frame = CGRectMake(0, 0, 40, 40);
UIBarButtonItem *flashImage = [[UIBarButtonItem alloc] initWithCustomView:imageView];
[footerItems addObject:flashImage];
[UIView animateWithDuration:0.3
delay: 0.0
options: UIViewAnimationOptionCurveEaseOut
animations:^{
self.toolbarItems = footerItems; }
completion:^(BOOL finished){
[UIView animateWithDuration:.3
delay: 0.0
options:UIViewAnimationOptionCurveEaseIn
animations:^{
self.toolbarItems = originalFooterItems;
}
completion:nil];
}];
}
回答2:
Heres the "highlight.png", I'm not kidding! (Though you may not seeing it on a white background.)
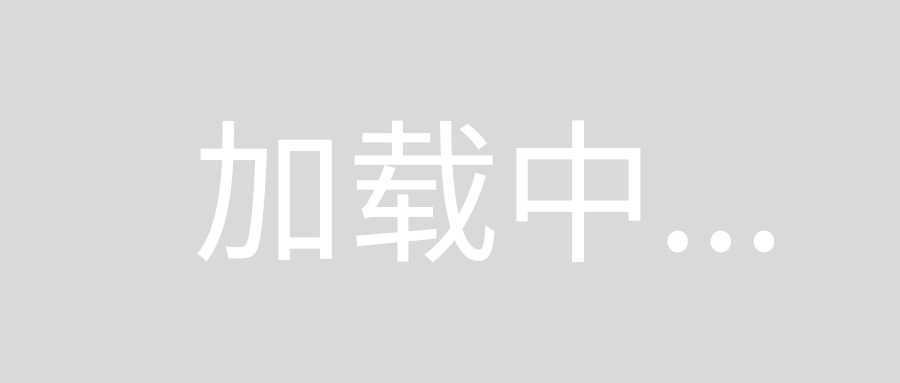
回答3:
For Bar items
[(UIButton *)[[toolbarItems objectAtIndex:1] customView] setImage:[UIImage imageNamed:@"highlight.png"] forState:UIControlStateNormal];
In General - Assuming you have a button
UIButton *button = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button addTarget:self
action:@selector(someFunction:)
forControlEvents:UIControlEventTouchDown];
[button setTitle:@"Click here" forState:UIControlStateNormal];
button.frame = CGRectMake(0.0, 0.0, 100.0, 40.0);
[self.view addSubview:button];
you can at any given point, programmatically call this function:
[button setTitle:@"Look Here" forState:UIControlStateNormal];
or if you like to have an highlight image
btnImage = [UIImage imageNamed:@"highlight.png"];
[button setImage:btnImage forState:UIControlStateNormal];
A very simple alternative:
That said , you can also set the button like this:
- (void)highlightButton:(UIButton *)button {
[button setHighlighted:YES];
}
回答4:
You can try to recreate a new bar button item with different style, and then reassign it back. My experiment was:
In viewDidLoad:
- (void)viewDidLoad
{
[super viewDidLoad];
UIBarButtonItem *rightBtn = [[UIBarButtonItem alloc] initWithTitle:@"Custom"
style:UIBarButtonItemStylePlain
target:self
action:nil];
self.navigationItem.rightBarButtonItem = rightBtn;
[rightBtn release];
[self performSelector:@selector(highlight)
withObject:nil
afterDelay:2.0];
}
And in method highlight:
- (void) highlight{
UIBarButtonItem *rightBtn = [[UIBarButtonItem alloc] initWithTitle:@"Custom"
style:UIBarButtonItemStyleDone
target:self
action:nil];
self.navigationItem.rightBarButtonItem = rightBtn;
[rightBtn release];
}
Your implementation might be different than mine, but as long as you are fine with reassigning the bar button, I think it'll work as expected. Good luck :)
回答5:
If you have a category:
#define HIGHLIGHT_TIME 0.5f
@interface UIButton (Highlight)
- (void)highlight;
@end
@implementation UIButton (Highlight)
- (void)highlight {
UIButton *overlayButton = [UIButton buttonWithType:UIButtonTypeCustom];
overlayButton.frame = self.frame;
overlayButton.showsTouchWhenHighlighted = YES;
[self.superview addSubview:overlayButton];
overlayButton.highlighted = YES;
[self performSelector:@selector(hideOverlayButton:) withObject:overlayButton afterDelay:HIGHLIGHT_TIME];
}
- (void)hideOverlayButton:(UIButton *)overlayButton {
overlayButton.highlighted = NO;
[overlayButton removeFromSuperview];
}
@end
Then you need code like this…
UIBarButtonItem *addButton = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemAdd target:self action:@selector(insertNewObject:)] autorelease];
self.navigationItem.rightBarButtonItem = addButton;
[self.navigationController.navigationBar.subviews.lastObject performSelector:@selector(highlight) withObject:nil afterDelay:2];
…to highlight the button in the navigation bar after 2 seconds.
回答6:
A simple ay is to init your UIBarButtonItem with a CustomView (UIBUtton)
UIButton *favButton = [UIButton buttonWithType:UIButtonTypeCustom];
[favButton setFrame:CGRectMake(0.0, 100.0, 50.0, 30.0)];
[favButton setBackgroundImage:[UIImage imageNamed:@"bouton_black_normal.png"]
forState:UIControlStateNormal];
[favButton setBackgroundImage:[UIImage imageNamed:@"bouton_black_hightlight.png"]
forState:UIControlStateHighlighted];
[favButton setTitle:@"Add" forState:UIControlStateNormal];
[favButton setTitle:@"Add" forState:UIControlStateHighlighted];
[favButton setTitleColor:[UIColor whiteColor] forState:UIControlStateNormal];
[favButton setTitleColor:[UIColor whiteColor] forState:UIControlStateHighlighted];
[[favButton titleLabel] setFont:[UIFont boldSystemFontOfSize:13]];
[favButton addTarget:self action:@selector(doSomething)
forControlEvents:UIControlEventTouchUpInside];
UIBarButtonItem *button = [[UIBarButtonItem alloc] initWithCustomView:favButton];