I am implementing a context menu for my kendo grid on MVC page. I am trying to call the edit button on my kendo grid using on click of the context menu. I have implemented event on my context menu and on the event written jquery code to call fire the click event of the edit button. I do see the window popping up for a split second and closing. How do I get this working
@(Html.Kendo().ContextMenu()
.Name("menu")
.Target("#GridTeam")
.Filter("tr")
.Orientation(ContextMenuOrientation.Vertical)
.Animation(animation =>
{
animation.Open(open =>
{
open.Fade(FadeDirection.In);
open.Duration(500);
});
})
.Items(items =>
{
items.Add()
.Text("Edit");
items.Add()
.Text("Delete");
})
.Events(e =>
{
e.Select("onEdit");
})
)
function onEdit(e) {
//Logic to be executed on Edit event
$('a.k-grid-edit').click();
You can use addRow, editRow and removeRow.
Model
public class ViewModel
{
public int Id { get; set; }
public string Name { get; set; }
public string Description { get; set; }
}
View
<script type="text/javascript">
function onSelect(e) {
var grid = $("#GridTeam").data("kendoGrid");
switch ($(e.item).children(".k-link").text()) {
case "Add":
grid.addRow(e.target);
break;
case "Edit":
grid.editRow(e.target);
break;
case "Delete":
grid.removeRow(e.target);
break;
}
}
</script>
@(Html.Kendo().Grid<ViewModel>()
.Name("GridTeam")
.Columns(columns =>
{
columns.Command(command => { command.Edit(); command.Destroy(); });
columns.Bound(d => d.Name).Title("Name");
columns.Bound(d => d.Description).Title("Description");
})
.Editable(editable => editable.Mode(GridEditMode.PopUp))
.DataSource(dataSource => dataSource
.Ajax()
.Model(model => model.Id(d => d.Id))
.Read(read => read.Action("Data_Read", "Home"))
.Update(update => update.Action("Data_Update", "Home"))
.Destroy(update => update.Action("Data_Destroy", "Home")))
)
@(Html.Kendo().ContextMenu()
.Name("menu")
.Target("#GridTeam")
.Filter("tr")
.Orientation(ContextMenuOrientation.Vertical)
.Animation(animation =>
{
animation.Open(open =>
{
open.Fade(FadeDirection.In);
open.Duration(500);
});
})
.Items(items =>
{
items.Add().Text("Add");
items.Add().Text("Edit");
items.Add().Text("Delete");
})
.Events(e => e.Select("onSelect"))
)
Controller
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult Data_Read([DataSourceRequest] DataSourceRequest request)
{
return Json(_models.ToDataSourceResult(request));
}
[HttpPost]
public ActionResult Data_Update([DataSourceRequest] DataSourceRequest request, ViewModel viewModel)
{
var model = _models.FirstOrDefault(x => x.Id == viewModel.Id);
if (model != null)
{
model.Name = viewModel.Name;
model.Description = viewModel.Description;
}
return Json(ModelState.IsValid ? new object() : ModelState.ToDataSourceResult());
}
[HttpPost]
public ActionResult Data_Destroy([DataSourceRequest] DataSourceRequest request, ViewModel viewModel)
{
_models.Remove(_models.First(x => x.Id == viewModel.Id));
return Json(new[] {_models}.ToDataSourceResult(request, ModelState));
}
// Created as static to simulate data from database
private static List<ViewModel> _models = new List<ViewModel>
{
new ViewModel {Id = 1, Name = "One", Description = "One Hundred"},
new ViewModel {Id = 2, Name = "Two", Description = "Two Hundreds"},
new ViewModel {Id = 3, Name = "Three", Description = "Three Hundreds"},
};
}
Screen Shot
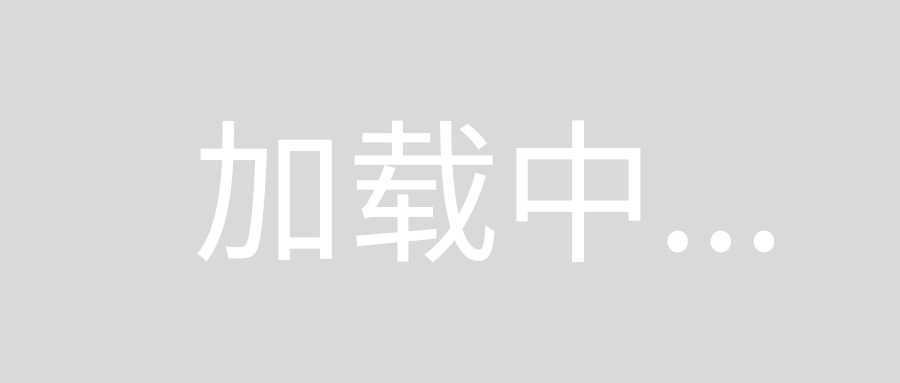
This should work.
First you get your grid instance. Then from context menu event find which row was clicked on. And then just put that row into edit mode.
function onEdit(e) {
//Logic to be executed on Edit event
var grid = $("#GridTeam").data("kendoGrid");
var model = e.target;
grid.editRow(model)
}