bool stringMatch(const char *expr, const char *str) {
// do something to compare *(expr+i) == '\\'
// In this case it is comparing against a backslash
// i is some integer
}
int main() {
string a = "a\sb";
string b = "a b";
cout << stringMatch(a.c_str(), b.c_str()) << endl;
return 1;
}
所以,现在的问题是:Xcode不是在“\”,当我在stringMatch函数调试读书,EXPR只似乎是“ASB”,而不是字面\某人。
和Xcode是吐出在该行的警告:字符串=“A \ SB”:未知转义序列
编辑:使用“A \\ SB”我已经尝试,它为“\\ SB”的字面读英寸
bool stringMatch(const char *expr, const char *str) {
// do something to compare *(expr+i) == '\\'
// In this case it is comparing against a backslash
// i is some integer
}
int main() {
string a = "a\\sb";
string b = "a b";
cout << stringMatch(a.c_str(), b.c_str()) << endl;
return 1;
}
C和C ++处理反斜杠因为默认情况下转义序列。 你得告诉C规定不通过添加额外的反斜线你的字符串使用反斜杠作为转义序列。
这些都是常见的转义序列:
- \一个 - 贝尔(嘟嘟)
- \ b - 退格
- \的F - 换页
- \ N - 新线
- \ r - 回车
- \ t - 水平制表
- \\ - 反斜杠
- \” - 单引号
- \” - 双Quatation马克
- \ OOO - 八进制表示
- \ XDD - 十六进制表达中
编辑:Xcode是你的机器上表现异常。 因此,我可以建议你这一点。
bool stringMatch(const char *expr, const char *str) {
// do something to compare *(expr+i) == '\\'
// In this case it is comparing against a backslash
// i is some integer
}
int main() {
string a = "a" "\x5C" "sb";
string b = "a b";
cout << stringMatch(a.c_str(), b.c_str()) << endl;
return 1;
}
不要担心在空间string a
声明,Xcode中串接一个空格隔开的字符串。
编辑2:其实Xcode是读你的"a\\b"
从字面上看,这就是它与逃跑反斜杠交易。 当你输出string a = "a\\sb"
安慰,你会看到, a\sb
。 但是,当你通过string a
的方法作为参数之间或私有成员,那么它会采取额外的反斜线字面。 你有那么它忽略额外的反斜杠来设计你的代码考虑到这一事实。 这是你高达你如何处理字符串。
编辑3: Edit 1
在这里你的最佳答案,但这里的另一个。
添加代码在你stringMatch()
方法来代替单反斜线双反斜杠。
你只需要在函数的一开始添加此额外的行:
expr=[expr stringByReplacingOccurrencesOfString:@"\\\\" withString:@"\\"];
这应该解决的双反斜线问题。
编辑4:有人认为编辑3的ObjectiveC,因此不是最佳的,所以在的ObjectiveC另一种选择++。
void searchAndReplace(std::string& value, std::string const& search,std::string const& replace)
{
std::string::size_type next;
for(next = value.find(search); // Try and find the first match
next != std::string::npos; // next is npos if nothing was found
next = value.find(search,next) // search for the next match starting after
// the last match that was found.
)
{
// Inside the loop. So we found a match.
value.replace(next,search.length(),replace); // Do the replacement.
next += replace.length(); // Move to just after the replace
// This is the point were we start
// the next search from.
}
}
编辑5:如果您更改const char *
在stringMatch()
为“string`它会为你那么复杂。
expr.replace(/*size_t*/ pos1, /*size_t*/ n1, /*const string&*/ str );
PS你现在有所有的答案,所以你高达你实现这一个,给你最好的结果是。
Xcode是吐出的警告,因为它是解释\s
在“A \ SB”作为转义序列,但\s
不是一个有效的转义序列。 它得到与刚刚更换s
因此字符串变成“ASB”。
逃离像反斜杠"a\\sb"
是正确的解决方案。 如果这有点不为你工作,请发表一下细节。
下面是一个例子。
#include <iostream>
#include <string>
int main() {
std::string a = "a\\sb";
std::cout << a.size() << ' ' << a << '\n';
}
这个程序的输出如下:
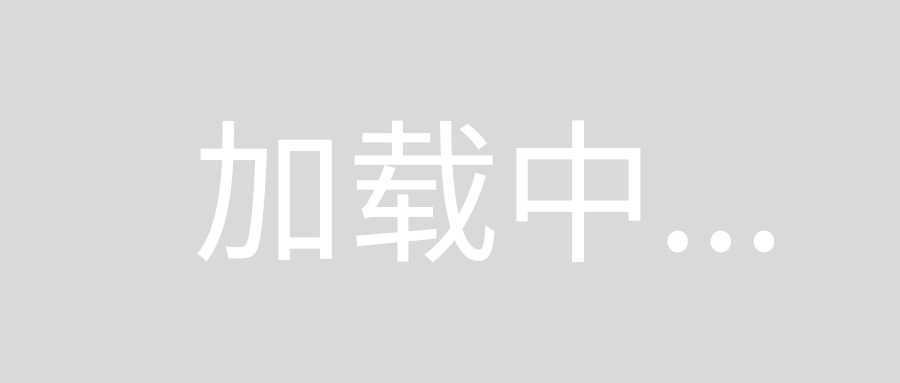
如果你得到不同的输出请张贴。 还请张贴正是你观察到的问题,当你试图“一\\ SB”更早。
Regexs可以在C ++的痛苦,因为反斜杠必须进行转义这种方式。 C ++ 11具有不允许任何种类的逃逸,使得逸出反斜杠是不必要的原始字符串: R"(a\sb)"
。