Hey I have a windows phone 8.1 app using the silverlight API.
I am downloading this image from my blob storage.
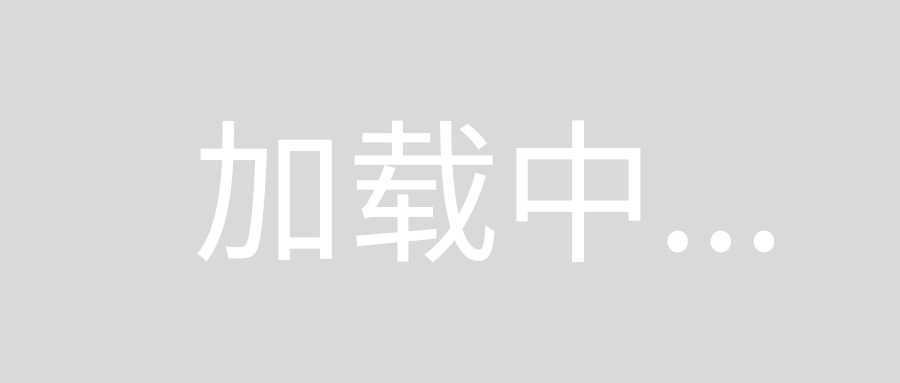
The image is coming from a link like this: https://[service].blob.core.windows.net/[imagename].png and the image can be showned and downloaded in multiple browsers, just using the URI.
I now want to use this as a imagebrush based on the imageuri from the blobstorage:
// If we have a returned SAS.
BitmapImage myOnlineImage = new BitmapImage();
myOnlineImage.UriSource = new Uri(uploadImage.ImageUri, UriKind.RelativeOrAbsolute);
//ImageOnlineTest.Source = myOnlineImage;
var imageBrush = new ImageBrush
{
ImageSource = myOnlineImage,
Stretch = Stretch.None
};
var source = FindChildShieldCanvas(CanvasImage, imageBrush);
WriteableBitmap wbm = new WriteableBitmap((BitmapImage)myOnlineImage);
ImageOnlineTest.Source = wbm;
The myOnlineImage
is not created correctly, at least I cannot convert the image to a writeablebitmapimage (getting a null exception from the conversion), and in addition the imagebrush is empty, i.e. null. But as far as I know this is the way to do it?
So basicly
How do I create an imagebrush
based on an url to a https site?
I ended up solving the issue myself:
Remember to add using System.Runtime.InteropServices.WindowsRuntime;
// If we have a returned SAS.
BitmapImage myOnlineImage = new BitmapImage();
//myOnlineImage.UriSource = new Uri(uploadImage.ImageUri, UriKind.RelativeOrAbsolute);
using (var webCLient = new Windows.Web.Http.HttpClient())
{
webCLient.DefaultRequestHeaders.Add("User-Agent", "bot");
var responseStream = await webCLient.GetBufferAsync(new Uri(uploadImage.ImageUri, UriKind.RelativeOrAbsolute));
var memoryStream = new MemoryStream();//responseStream.ToArrayAsStream().ReadAsync());
memoryStream.Write(responseStream.ToArray(), 0, responseStream.ToArray().Length);
memoryStream.Position = 0;
myOnlineImage.SetSource(memoryStream);
}
//ImageOnlineTest.Source = myOnlineImage;
var imageBrush = new ImageBrush
{
ImageSource = myOnlineImage,
Stretch = Stretch.None
};
var source = FindChildShieldCanvas(CanvasImage, imageBrush);
WriteableBitmap wbm = new WriteableBitmap((BitmapImage)myOnlineImage);
This code works, both for the imagebrush and writeablebitmap
To create a bitmap from an image you have to start the initialization of the bitmap object prior to setting the URL.
BitmapImage myOnlineImage = new BitmapImage();
myOnlineImage.BeginInit();
myOnlineImage.UriSource = new Uri(uploadImage.ImageUri, UriKind.RelativeOrAbsolute);
myOnlineImage.EndInit();
var imageBrush = new ImageBrush
{
ImageSource = myOnlineImage,
Stretch = Stretch.None
};
var source = FindChildShieldCanvas(CanvasImage, imageBrush);
WriteableBitmap wbm = new WriteableBitmap((BitmapImage)myOnlineImage);
ImageOnlineTest.Source = wbm;
You can do it with C# in the following way, replace the appropriate code with your fields.
Rectangle rectangle = new Rectangle();
rectangle.StrokeThickness = 10;
rectangle.Height = 200;
rectangle.Width = 100;
rectangle.SetValue(Canvas.LeftProperty, 100d);
rectangle.SetValue(Canvas.TopProperty, 100d);
rectangle.Fill = new ImageBrush(new BitmapImage(new Uri(@"C:\User\xiaorui.dong\Pictures\profile.jpeg")));