I am developing a simple android application and I need to write a text file in internal storage device. I know there are a lot of questions (and answers) about this matter but I really cannot understand what I am doing in the wrong way.
This is the piece of code I use in my activity in order to write the file:
public void writeAFile(){
String fileName = "myFile.txt";
String textToWrite = "This is some text!";
FileOutputStream outputStream;
try {
outputStream = openFileOutput(fileName , Context.MODE_PRIVATE);
outputStream.write(textToWrite.getBytes());
outputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
I really cannot understand which mistake I am doing. In addition, I have tried this project on my emulator in Android Studio and my phone in order to understand where I am doing something wrong but even with that project no file is written neither on the phone or on the emulator.
EDIT:
I know that no file is written to my internal storage because I try to read the content of the file, after I have written to it, with this code:
public void ReadBtn(View v) {
//reading text from file
try {
FileInputStream fileIn=openFileInput("myFile.txt");
InputStreamReader InputRead= new InputStreamReader(fileIn);
char[] inputBuffer= new char[READ_BLOCK_SIZE];
String s="";
int charRead;
while ((charRead=InputRead.read(inputBuffer))>0) {
String readstring=String.copyValueOf(inputBuffer,0,charRead);
s +=readstring;
}
InputRead.close();
textmsg.setText(s);
} catch (Exception e) {
e.printStackTrace();
}
}
Nothing is shown at all.
Use below code to write a file to internal storage
EDIT: added e.printStackTrace();
to catch block as a good practice and permissions reminders
public void writeFileOnInternalStorage(Context mcoContext,String sFileName, String sBody){
File file = new File(mcoContext.getFilesDir(),"mydir");
if(!file.exists()){
file.mkdir();
}
try{
File gpxfile = new File(file, sFileName);
FileWriter writer = new FileWriter(gpxfile);
writer.append(sBody);
writer.flush();
writer.close();
}catch (Exception e){
e.printStackTrace();
}
}
And don't forget to add external storage writing AND reading permissions in the manifest:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
no file is written neither on the phone or on the emulator.
Yes, there is. It is written to what the Android SDK refers to as internal storage. This is not what you as a user consider to be "internal storage", and you as a user cannot see what is in internal storage on a device (unless it is rooted).
If you want to write a file to where users can see it, use external storage.
This sort of basic Android development topic is covered in any decent book on Android app development.
Save to Internal storage
data="my Info to save";
try {
FileOutputStream fOut = openFileOutput(file,MODE_WORLD_READABLE);
fOut.write(data.getBytes());
fOut.close();
Toast.makeText(getBaseContext(),"file saved",Toast.LENGTH_SHORT).show();
}
catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
Read from Internal storage
try {
FileInputStream fin = openFileInput(file);
int c;
String temp="";
while( (c = fin.read()) != -1){
temp = temp + Character.toString((char)c);
}
tv.setText(temp);
Toast.makeText(getBaseContext(),"file read",Toast.LENGTH_SHORT).show();
}
catch(Exception e){
}
Have you requested permission to write to external storage?
https://developer.android.com/training/basics/data-storage/files.html#GetWritePermission
Perhaps your try\catch block is swallowing an exception that could be telling you what the problem is.
Also, you do not appear to be setting the path to save to.
e.g: Android how to use Environment.getExternalStorageDirectory()
To view files on a device, you can log the file location >provided by methods such as File.getAbsolutePath(), and >then browse the device files with Android Studio's Device >File Explorer.
I had a similar problem, the file was written but I never saw it. I used the Device file explorer and it was there waiting for me.
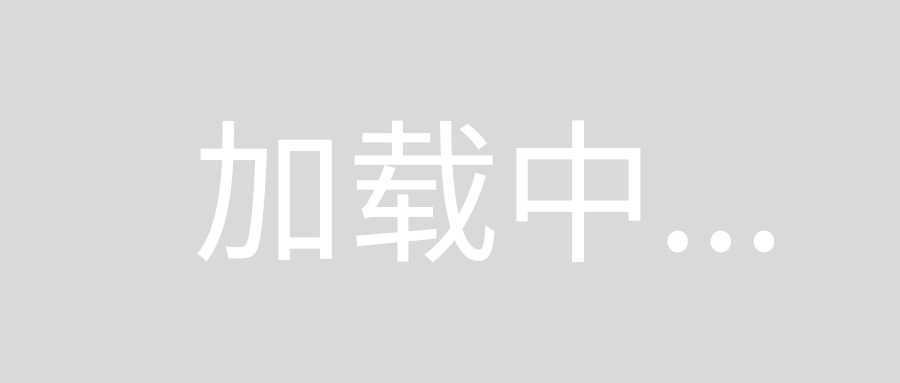
String filename = "filename.jpg";
File dir = context.getFilesDir();
File file = new File(dir, filename);
try {
Log.d(TAG, "The file path = "+file.getAbsolutePath());
file.createNewFile();
} catch (Exception e) {
e.printStackTrace();
}
return true;