可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
Here's my code
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return 10
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
var cell :UITableViewCell = tableView.dequeueReusableCellWithIdentifier("discovered") as UITableViewCell!
bluetoothlog.registerNib(UINib(nibName: "devicedet", bundle: nil), forCellReuseIdentifier: "discovered")
//NSBundle.mainBundle().loadNibNamed("devicedet", owner: nil, options: nil)[0] as UITableViewCell
cell = blucell
devname.text = peri[indexPath.row]
devstrength.text = signalstrength[indexPath.row]
bluetoothlog.backgroundColor = UIColor.clearColor()
return cell
}
I have tried with the above code and nothing is displayed in Tableview, please help me to change this code working
Thanks
回答1:
import UIKit
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
//MARK:- Sample Data Array to load in TableView
let sampleArrray: \[String\] = \["val1", "val2", "val3", "val4", "val5"]
//MARK:- Cell reuse identifier
let cellReuseIdentifier = "cell"
//MARK:- UITableView outlet
@IBOutlet var tableView: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
// Registering the custom cell
self.tableView.register(UITableViewCell.self, forCellReuseIdentifier: cellReuseIdentifier)
// Setting delegate and Datasourse as same viewcontroller (this code is not neccessory if we are setting it from storyboard)
tableView.delegate = self
tableView.dataSource = self
}
//UITableview required methods
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return sampleArrray.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell:UITableViewCell = tableView.dequeueReusableCellWithIdentifier(cellReuseIdentifier) as UITableViewCell!
cell.textLabel?.text = sampleArrray\[indexPath.row\]
return cell
}
func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
print("clicked at: \(indexPath.row)")
//Here u can get the selected cell and add action related to this cell seelction
}
Added Screenshot for setting Datasource and delegate from storyboard
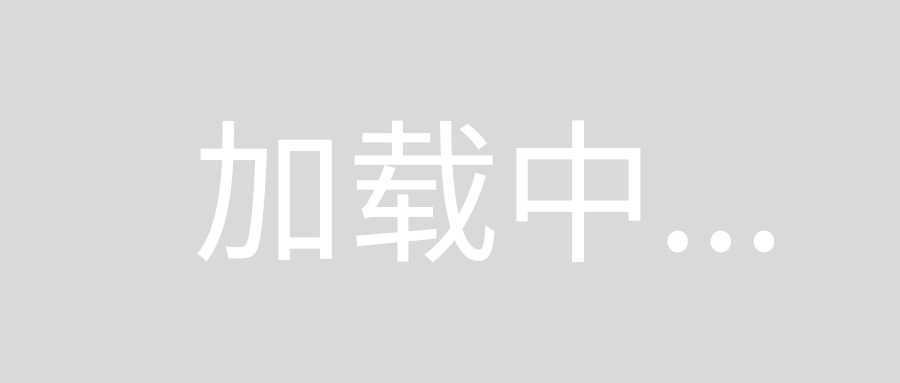
回答2:
Register your NIB with the reuse identifier:
override func viewDidLoad() {
super.viewDidLoad()
tableView.registerNib(UINib(nibName: "devicedet", bundle: nil), forCellReuseIdentifier: "Cell")
}
Your cellForRowAtIndexPath would then instantiate the cell.
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Cell", forIndexPath: indexPath) as! devicedet
return cell
}
回答3:
- the command registerNib should be called in ViewDidLoad, but not in each call of cell for row
- what is 'bluecell'? In your call-back cellforTable.., you assign cell = blucell -> this can cause your problems.
Try this:
import UIKit
class YourViewController: UITableViewController, UITableViewDataSource, UITableViewDelegate {
override func viewDidLoad() {
super.viewDidLoad()
//call register nib here!!
bluetoothlog.registerNib(UINib(nibName: "devicedet", bundle: nil), forCellReuseIdentifier: "discovered")
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return peri.count
}
//it seem that you have to add a è
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell!
{
let cell :UITableViewCell = tableView.dequeueReusableCellWithIdentifier("discovered") as UITableViewCell!
/* REMOVE THIS
bluetoothlog.registerNib(UINib(nibName: "devicedet", bundle: nil), forCellReuseIdentifier: "discovered")
//NSBundle.mainBundle().loadNibNamed("devicedet", owner: nil, options: nil)[0] as UITableViewCell
*/
//// THEN, UPDATE YOUR CELL with YOUR DATAs
/* I don't understand what are you doing with this line of code:
cell = blucell
*/
devname.text = peri[indexPath.row]
devstrength.text = signalstrength[indexPath.row]
//Seem that bluetoothlog is the tableview, you should call it in viewdidload or viewwillappear()
bluetoothlog.backgroundColor = UIColor.clearColor()
return cell
}
}
回答4:
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
var cell :devicedet = tableView.dequeueReusableCellWithIdentifier("discovered") as UITableViewCell!
bluetoothlog.registerNib(UINib(nibName: "devicedet", bundle: nil), forCellReuseIdentifier: "discovered")
//NSBundle.mainBundle().loadNibNamed("devicedet", owner: nil, options: nil)[0] as UITableViewCell
cell = blucell
devname.text = peri[indexPath.row]
devstrength.text = signalstrength[indexPath.row]
bluetoothlog.backgroundColor = UIColor.clearColor()
return cell
}
回答5:
In viewDidLoad register XIB as shown below
var userDetailsNIB = UINib(nibName: "UserDetailsCell", bundle: nil)
viewListingTable.registerNib(userDetailsNIB, forCellReuseIdentifier: "UserDetailsViewCellID")
And in func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell{
function use it by access it with Identifier which created in viewDidLoad method
let cell = tableView.dequeueReusableCellWithIdentifier("UserDetailsViewCellID") as UserDetailsViewCell
return cell
回答6:
if you would like to Load custom cell(Xib) in UITableView in swift ,you may use the following code.
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return array.count; //This function returns number of rows in table view
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
var cell:YourTableViewCell! = tableView.dequeueReusableCellWithIdentifier("YourTableViewCell", forIndexPath:indexPath)as! YourTableViewCell
// Do additional code here
}
Don’t forget to register nib in viewDidLoad
override func viewDidLoad() {
super.viewDidLoad()
// registering your nib
let yourNibName = UINib(nibName: "YourTableViewCell", bundle: nil)
tableView.registerNib(yourNibName, forCellReuseIdentifier: "YourTableViewCell")
// Do any additional setup after loading the view.
}
回答7:
Register xib
Cell in Swift
override func viewDidLoad() {
super.viewDidLoad()
tableView.register(UINib(nibName: "XibCell", bundle: nil), forCellReuseIdentifier: "Cell")
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell") as! UITableViewCell
return cell
}
回答8:
My code:
override func viewDidLoad() {
super.viewDidLoad()
tableView.register(
UINib(nibName: "XibCell", bundle: nil),
forCellReuseIdentifier: "Cell"
)
}
func tableView(_ tableView: UITableView,
cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell")
return cell as! UITableViewCell
}
回答9:
Your fuction registerNib , you try to write in viewDidLoad() method and remove from cellForRowAtIndexPah and then test your project
Your Cell Instance should be of CustomCell instance and not of UITableView Cell.
var cell :UITableViewCell should be replaced by
var cell :devicedet