I am new to C and this is my code.
#include<stdio.h>
void printCard(char name, char symbol);
int main()
{
printCard('J','*');
printCard('K','&');
}
void printCard(char name, char symbol)
{
printf("-------------\n");
printf("| %c |\n",name);
printf("| %c |\n",symbol);
printf("| |\n");
printf("| |\n");
printf("| |\n");
printf("| %c |\n",symbol);
printf("| %c |\n",name);
printf("-------------\n");
}
This is the output that I am getting.
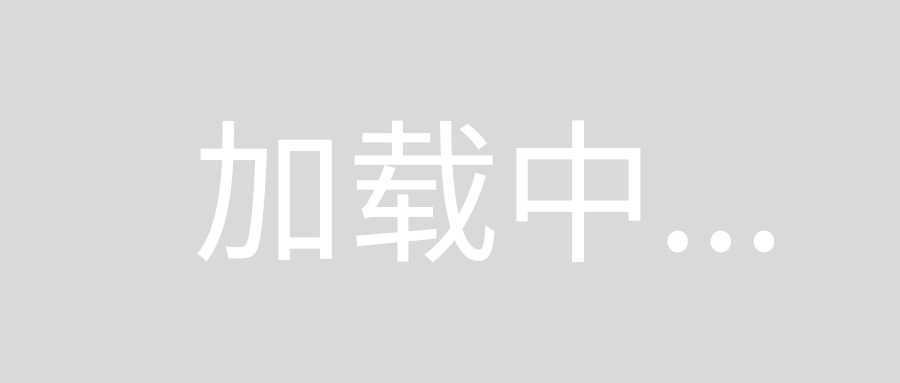
Is there a way to get that second card to appear beside the first?
Thanks
If your terminal supports them, these terminal escape codes could be use to position the cursor.
#include<stdio.h>
void printCard( int col, char name, char symbol);
int main()
{
printf ( "\033[2J");//clear screen
printCard( 0, 'J','*');
printCard( 1, 'K','&');
return 0;
}
void printCard(int col, char name, char symbol)
{
printf ( "\033[1;%dH", col * 14);//move cursor line 1
printf("-------------\n");
printf ( "\033[2;%dH", col * 14);//move cursor line 2
printf("| %c |\n",name);
printf ( "\033[3;%dH", col * 14);//move cursor line 3
printf("| %c |\n",symbol);
printf ( "\033[4;%dH", col * 14);//move cursor line 4
printf("| |\n");
printf ( "\033[5;%dH", col * 14);//move cursor line 5
printf("| |\n");
printf ( "\033[6;%dH", col * 14);//move cursor line 6
printf("| |\n");
printf ( "\033[7;%dH", col * 14);//move cursor line 7
printf("| %c |\n",symbol);
printf ( "\033[8;%dH", col * 14);//move cursor line 8
printf("| %c |\n",name);
printf ( "\033[9;%dH", col * 14);//move cursor line 9
printf("-------------\n");
}
This was the function that worked for me.
void gotoXY(int x, int y)
{
COORD coord;
coord.X = x;
coord.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
}
Thanks @PCLuddite for pointing me to check setConsoleCursorPosition which helped me find this above function online. Hope this helps others.