EDIT: Okay, so I've managed to get it working now with the help of a friend. =)
I am currently creating a Java applet. The applet works by taking text input from the user and displaying it to the screen when they press return. This is the point up to which my program is working at the moment.
I was wondering whether there was a way in which I could make it so the text input/String is drawn when the mouse is clicked, at the point of mouse click.
Many thanks in advance to anyone who can help me out with this. :)
I was wondering whether there was a way in which I could make it so
the text input/String is drawn when the mouse is clicked, at the point
of mouse click.
Answer: Yes...
Would it be rude of me to leave the answer as that...?
This is a relatively simple process, depending on what you want to achieve...
This example just uses Graphics#drawString
to render text to a custom component. You could, equally, just at a label to the component at the specified point, but that's another can of worms.
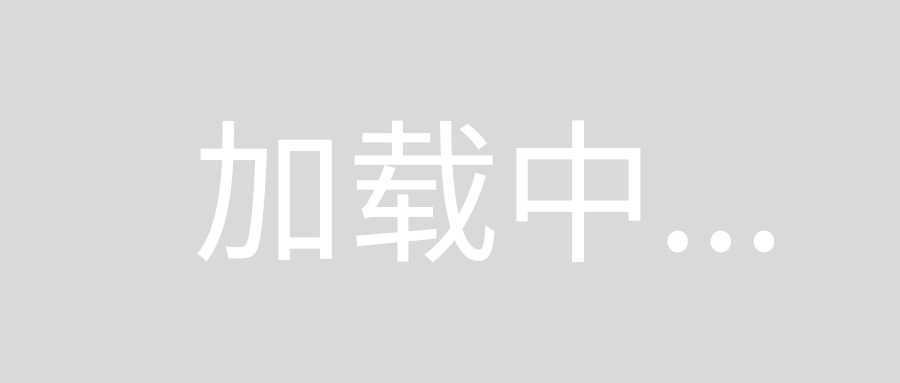
public class TestDrawText {
public static void main(String[] args) {
new TestDrawText();
}
public TestDrawText() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (Exception ex) {
}
JFrame frame = new JFrame("Test");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
private Point textPoint;
public TestPane() {
addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
textPoint = e.getPoint();
repaint();
}
});
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
if (textPoint != null) {
FontMetrics fm = g.getFontMetrics();
g.drawString("You clicked at " + textPoint.x + "x" + textPoint.y, textPoint.x, textPoint.y + fm.getAscent());
}
}
}
}
Check out
- How to Write a Mouse Listener
- Performing Custom Painting
For more info.