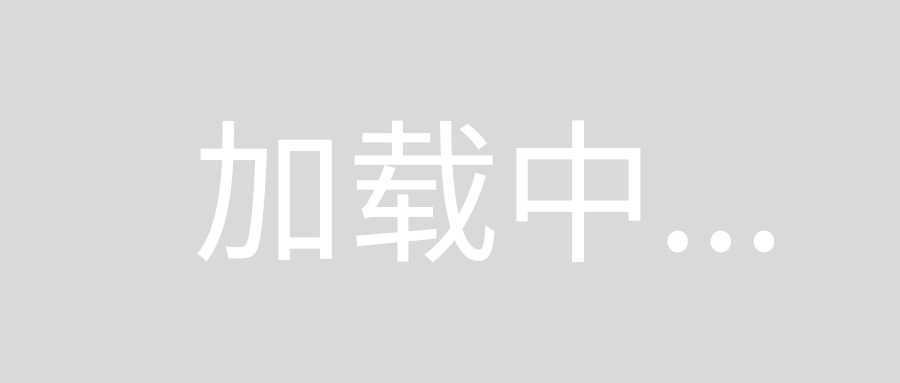
When click on add phone text I want to add view dynamically (view below add phone: where 'mobile' is textview
and on right side there is edit text)
and this need to be for N level ..when user click on add phone view will be added..
How to do this.?
Try this code.This might help you.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/btn_Click"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Click" />
<LinearLayout
android:id="@+id/linearView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical" />
</LinearLayout>
In your Activity class on button Click just use the below method.And make sure to declare an int variable (index) that will help you add new View to the end.
int index=0;
linearView = (LinearLayout) findViewById(R.id.linearView);
@OnClick(R.id.btn_Click)
public void click() {
LinearLayout mainLinearLayout = new LinearLayout(this);
mainLinearLayout.setOrientation(LinearLayout.VERTICAL);
LinearLayout.LayoutParams mainParams = new LinearLayout.LayoutParams(LinearLayout.LayoutParams.MATCH_PARENT, LinearLayout.LayoutParams.WRAP_CONTENT);
mainLinearLayout.setLayoutParams(mainParams);
mainLinearLayout.setGravity(Gravity.CENTER);
LinearLayout firstChildLinearLayout = new LinearLayout(this);
firstChildLinearLayout.setOrientation(LinearLayout.HORIZONTAL);
LinearLayout.LayoutParams firstChildParams = new LinearLayout.LayoutParams(LinearLayout.LayoutParams.MATCH_PARENT, LinearLayout.LayoutParams.MATCH_PARENT);
firstChildLinearLayout.setLayoutParams(firstChildParams);
TextView textView = new TextView(this);
LinearLayout.LayoutParams txtParams = new LinearLayout.LayoutParams(LinearLayout.LayoutParams.WRAP_CONTENT, LinearLayout.LayoutParams.MATCH_PARENT);
txtParams.setMarginStart(5);
txtParams.setMarginEnd(10);
textView.setGravity(Gravity.CENTER_VERTICAL);
textView.setLayoutParams(txtParams);
textView.setCompoundDrawablesWithIntrinsicBounds(getResources().getDrawable(R.drawable.ic_foreground), null, null, null);
textView.setText("mobile >");
EditText editText = new EditText(this);
LinearLayout.LayoutParams etParams = new LinearLayout.LayoutParams(LinearLayout.LayoutParams.WRAP_CONTENT, LinearLayout.LayoutParams.MATCH_PARENT);
etParams.weight = 1;
editText.setBackground(null);
editText.setLayoutParams(etParams);
firstChildLinearLayout.addView(textView, 0);
firstChildLinearLayout.addView(editText, 1);
LinearLayout secondChildLinearLayout = new LinearLayout(this);
secondChildLinearLayout.setOrientation(LinearLayout.HORIZONTAL);
secondChildLinearLayout.setBackgroundColor(getResources().getColor(R.color.color_grey));
LinearLayout.LayoutParams secondChildParams = new LinearLayout.LayoutParams(LinearLayout.LayoutParams.MATCH_PARENT, 1);
secondChildLinearLayout.setLayoutParams(secondChildParams);
mainLinearLayout.addView(firstChildLinearLayout, 0);
mainLinearLayout.addView(secondChildLinearLayout, 1);
linearView.addView(mainLinearLayout, index);
index++;
}
So to get values from EditText use following code. There I have displayed the value in Toast, you can use an array of String to store all dynamically created EditText values.
public void getAllEditTextValues(View view) {
View v = null;
for (int i = 0; i < linearView.getChildCount(); i++) {
v = linearView.getChildAt(i);
if (v instanceof LinearLayout) {
View tempView = ((LinearLayout) v).getChildAt(0);
View et = ((LinearLayout) tempView).getChildAt(1);
String etValue = null;
if (et instanceof EditText) {
etValue = ((EditText) et).getText().toString();
}
Toast.makeText(this, "" + etValue, Toast.LENGTH_SHORT).show();
// Use Array to Store all values of EditText
}
}
}
Create XML as "custom_row.xml" with code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:layout_width="0dp"
android:layout_weight="0.1"
android:id="@+id/crossImage"
android:layout_gravity="center_vertical"
android:layout_height="wrap_content"
android:src="@drawable/cross_image"/>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.45"
android:id="@+id/columnSpinner"
android:text="demo"
android:layout_gravity="center"/>
<EditText
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.45"
android:id="@+id/contentValueEditText"/>
</LinearLayout>
Now make you activity layout as:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:id="@+id/linearView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical" />
<Button
android:id="@+id/btn_Click"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Click" />
</LinearLayout>
Now do code as:
int index=0;
linearView = (LinearLayout) findViewById(R.id.linearView);
@OnClick(R.id.btn_Click)
public void click() {
final LinearLayout singleInsertView1 = (LinearLayout) LayoutInflater.from(QueryRunner.this).inflate(R.layout.insert_single_item, null);
EditText editText = (EditText)singleInsertView1.findViewById(R.id.contentValueEditText);
editText.setTag("EditText"+index);
singleInsertView1.setTag("LinearLayout"+index);
contentViewAdder.addView(singleInsertView1);
index++;
}
Now you can access the Edit Text by following code:
LinearLayout singleLayout = (LinearLayout)linearView.findViewWithTag("LinearLayout"+index);
EditText editText = singleLayout.findViewWithTag("EditText"+index);