Im struggling with some iOS development and I hope someone will be able to help me with an advice. I want to implement a stack of UIViews that will look like a deck of cards. User should be able to swipe out 'cards' with touch. I thought of UIScrollViews with pagingEnabled to give an impression that cards are separate. However, UIScrollView can swipe from horizontally where something appears from left or right side. I want my deck of cards to be in the middle of the screen so the user can swipe cards from the deck without seeing the card appearing on the left or right side.
Also, I was thinking of using Touch Methods ( touchesBegan, touchesMoved, etc.) to move views. It could work... but Im worried that I wont be able to reproduce proper mechanics of the card ( friction, bouncing effect when the swipe is to short and so on..).
Can someone please point me to a proper technique or advice some tutorials? I did some research already but I couldn't find anything that would be helpful.
Here is an image of the effect that I would like to achieve.
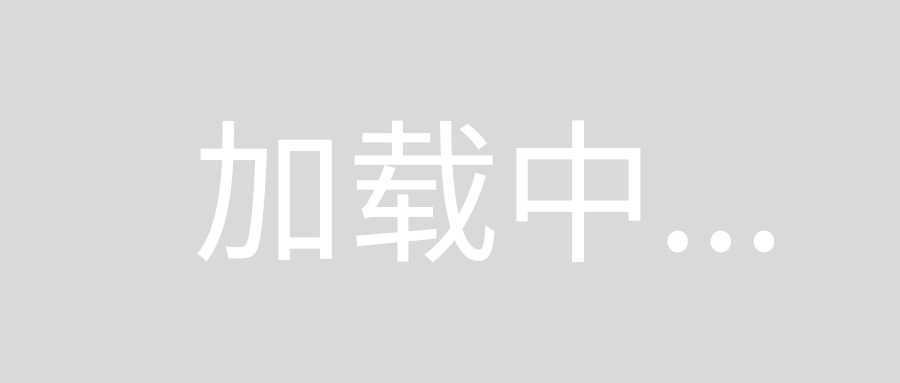
And what I want to achieve is to swipe cards out of the deck.
Thanks a lot!
I guess you want a swipe to take the top card of the stack and “shuffle” it to the bottom of the stack, revealing the second card on the stack, like this:
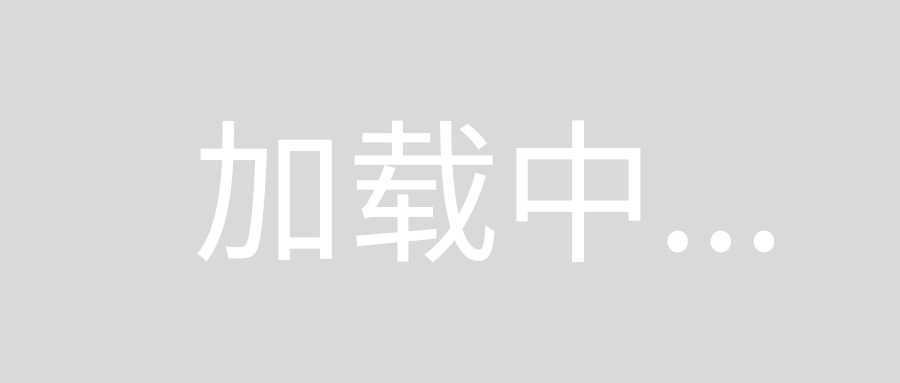
The animation is much smoother in real life. I had to use a low frame rate to make the animated GIF small.
One way to do this is to create a subclass of UIView
that manages the stack of card views. Let's call the manager view a BoardView
. We'll give it two public methods: one for shuffling the top card to the bottom, and one for shuffling the bottom card to the top.
@interface BoardView : UIView
- (IBAction)goToNextCard;
- (IBAction)goToPriorCard;
@end
@implementation BoardView {
BOOL _isRestacking;
}
We'll use the _isRestacking
variable to track whether the board view is animating the movement of a card off to the side.
A BoardView
treats all of its subviews as card views. It takes care of stacking them up, with the top card centered. In your screen shot, you offset the lower cards by slightly randomized amounts. We can make it look a little sexier by rotating the lower cards randomly. This method applies a small random rotation to a view:
- (void)jostleSubview:(UIView *)subview {
subview.transform = CGAffineTransformMakeRotation((((double)arc4random() / UINT32_MAX) - .5) * .2);
}
We'll want to apply that to each subview as it's added:
- (void)didAddSubview:(UIView *)subview {
[self jostleSubview:subview];
}
The system sends layoutSubviews
whenever a view's size changes, or when a view has been given new subviews. We'll take advantage of that to lay out all of the cards in a stack in the middle of the board view's bounds. But if the view is currently animating a card out of the way, we don't want to do the layout because it would kill the animation.
- (void)layoutSubviews {
if (_isRestacking)
return;
CGRect bounds = self.bounds;
CGPoint center = CGPointMake(CGRectGetMidX(bounds), CGRectGetMidY(bounds));
UIView *topView = self.subviews.lastObject;
CGFloat offset = 10.0f / self.subviews.count;
for (UIView *view in self.subviews.reverseObjectEnumerator) {
view.center = center;
if (view == topView) {
// Make the top card be square to the edges of the screen.
view.transform = CGAffineTransformIdentity;
}
center.x -= offset;
center.y -= offset;
}
}
Now we're ready to handle the shuffling. To “go to the next card”, we need to animate moving the top card off to the side, then move it to the bottom of the subview stacking order, and then animate it back to the middle. We also need to nudge the positions of all of the other cards because they've all moved closer to the top of the stack.
- (void)goToNextCard {
if (self.subviews.count < 2)
return;
First, we animate the movement of the top card off to the side. To make it look sexy, we rotate the card as we move it.
UIView *movingView = self.subviews.lastObject;
[UIView animateWithDuration:1 delay:0 options:UIViewAnimationCurveEaseInOut animations:^{
_isRestacking = YES;
CGPoint center = movingView.center;
center.x = -hypotf(movingView.frame.size.width / 2, movingView.frame.size.height / 2);
movingView.center = center;
movingView.transform = CGAffineTransformMakeRotation(-M_PI_4);
}
In the completion block, we move the card to the bottom of the stack.
completion:^(BOOL finished) {
_isRestacking = NO;
[self sendSubviewToBack:movingView];
And to move the now-bottom card back into the stack, and nudge all of the other cards, we'll just call layoutSubviews
. But we're not supposed to call layoutSubviews
directly, so instead we use the proper APIs: setNeedsLayout
followed by layoutIfNeeded
. We call layoutIfNeeded
inside an animation block so the cards will be animated into their new positions.
[self setNeedsLayout];
[UIView animateWithDuration:1 delay:0 options:UIViewAnimationCurveEaseInOut | UIViewAnimationOptionAllowUserInteraction animations:^{
[self jostleSubview:movingView];
[self layoutIfNeeded];
} completion:nil];
}];
}
That's the end of goToNextCard
. We can do goToPriorCard
similarly:
- (void)goToPriorCard {
if (self.subviews.count < 2)
return;
UIView *movingView = [self.subviews objectAtIndex:0];
[UIView animateWithDuration:1 delay:0 options:UIViewAnimationOptionCurveEaseInOut animations:^{
_isRestacking = YES;
CGPoint center = movingView.center;
center.x = -movingView.frame.size.height / 2;
movingView.center = center;
movingView.transform = CGAffineTransformMakeRotation(-M_PI_4);
} completion:^(BOOL finished) {
_isRestacking = NO;
UIView *priorTopView = self.subviews.lastObject;
[self bringSubviewToFront:movingView];
[self setNeedsLayout];
[UIView animateWithDuration:1 delay:0 options:UIViewAnimationOptionCurveEaseInOut | UIViewAnimationOptionAllowUserInteraction animations:^{
[self jostleSubview:priorTopView];
[self layoutIfNeeded];
} completion:nil];
}];
}
Once you have BoardView
, you just need to attach a swipe gesture recognizer that sends it the goToNextCard
message, and another swipe gesture recognizer that sends it the goToPriorCard
message. And you need to add some subviews to act as cards.
Another detail: to get the edges of the cards to look smooth when they're jostled, you need to set UIViewEdgeAntialiasing
to YES
in your Info.plist
.
You can find my test project here: http://dl.dropbox.com/u/26919672/cardstack.zip
Icarousel has that exact built in scrolling using UIImageViews.
https://github.com/nicklockwood/iCarousel. There's several different effects in there, I forget the name of the one you're describing.