I am trying to write a C program that will use fork() and wait() in Visual Studio 2013. I have downloaded and installed Cygwin, but I am unable to configure Visual Studio to use the appropriate header files. I'm fairly new to the IDE and I was wondering if this community could help me figure out where I am making a mistake.
When starting a new project, this is what I do:
- Create new Visual C++ Win32 Console Application
- Add new main.c source file to the project
- Right-click project properties and configure Include Directories to
include the Cygwin directory: C:\cygwin64
I've taken a screenshot of where I am trying to configure the properties in case this is where I am making my mistake: 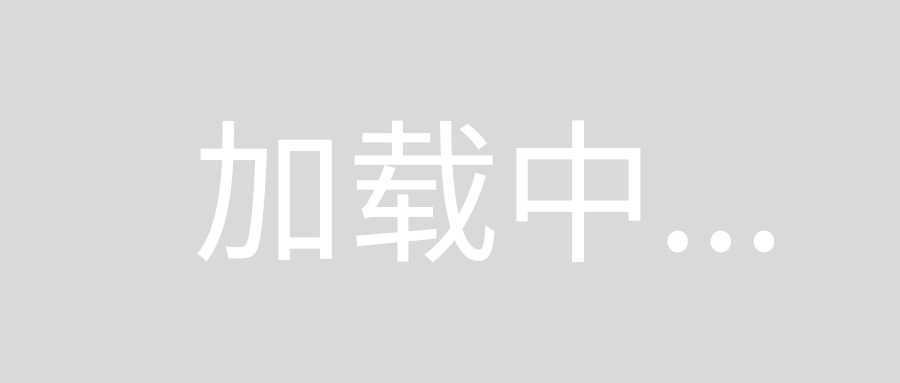
My code is rather simple as I am just trying to get it to run at this point:
#include <unistd.h>
int main() {
int i;
i = fork();
if (i == 0) {
printf("I am the child.");
}
else {
wait(&i);
}
}
Here's a screenshot of the error message I receive when I try to build my project: 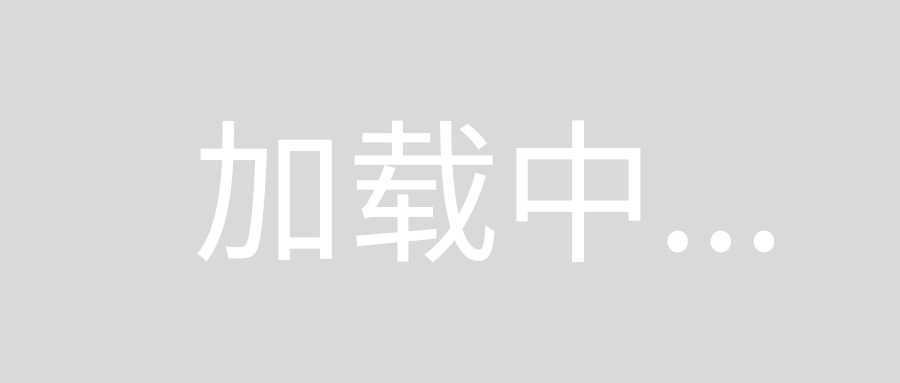
I apologize in advance if this is a silly question, but I do appreciate any help that you can offer. If there is anything that I can do on my end to help troubleshoot, please let me know.
The problem is that your current include path C:\cygwin64\bin
is equivalent to unix /bin
, which is for binaries (aka executables). To use unix headers, you need to use the equivalent of /usr/include
, which, for your system, should be C:\cygwin64\usr\include
.
Using fork() within a Win32 Console application is not going to work. It's a Unix system call. Cygwin is an emulation of the Linux environment that runs on top of Win32.
As others have said you need to be using the Cygwin toolchain to do your compile and builds.
There may be third party libraries to allow Visual Studio to cross-compile for Cygwin, but a Win32 console application is definitely not what you want if you re making Unix system calls.
Ok, there are lots of pieces here to do this, and there are also a lot of answers here with incorrect information.
First, as some have said, you need to modify your include path to contain your include folder from Cygwin, from your install that appears to be C:\Cygwin64\usr\include
.
Next, there is an FAQ on this very thing on the Cygwin site. How do I use cygwin1.dll with Visual Studio or MinGW?
From the page:
Use the impdef program to generate a .def file for the cygwin1.dll (if
you build the cygwin dll from source, you will already have a def
file)
impdef cygwin1.dll > cygwin1.def
Use the MS VS linker (lib) to generate an import library
lib /def=cygwin1.def /out=cygwin1.lib
Create a file "my_crt0.c" with the following contents
#include <sys/cygwin.h>
#include <stdlib.h>
typedef int (*MainFunc) (int argc, char *argv[], char **env);
void my_crt0 (MainFunc f) {
cygwin_crt0(f);
}
Use gcc in a Cygwin prompt to build my_crt0.c into a DLL (e.g. my_crt0.dll).
Follow steps 1 and 2 to generate .def and .lib files for the DLL.
Download crt0.c from the cygwin website and include it in your
sources. Modify it to call my_crt0() instead of cygwin_crt0().
Build your object files using the MS VC compiler cl.
Link your object files, cygwin1.lib, and my_crt0.lib (or whatever you
called it) into the executable.
Note that if you are using any other Cygwin based libraries that you
will probably need to build them as DLLs using gcc and then generate
import libraries for the MS VC linker.