Is there a way to fix the drawing order of PointPlacemarks in World Wind?
Even in the Placemarks example the ordering of Placemark B and the Audio Placemark can change just by moving the mouse at certain zoom levels:
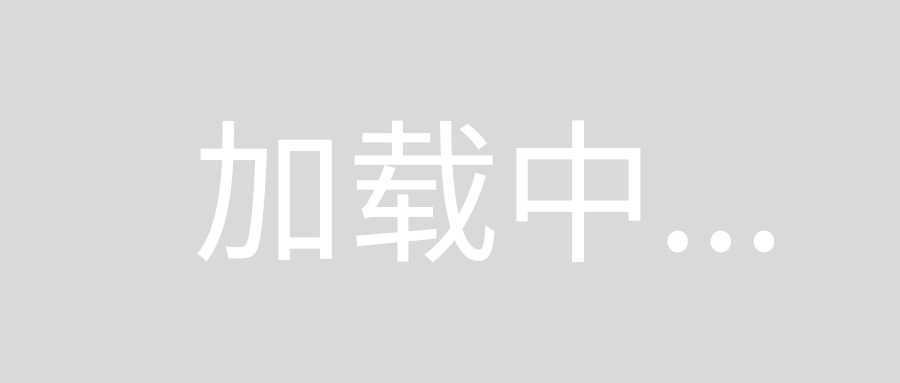
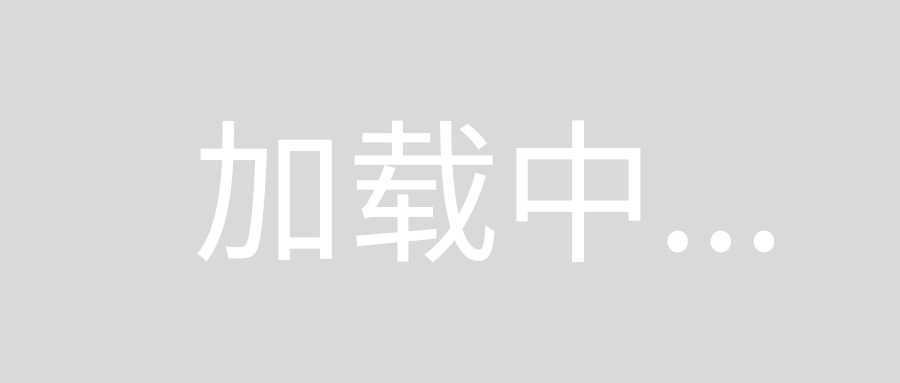
So far I have tried:
- Adding PointPlacemarks to separate layers
- Using
RenderableLayer.setRenderables
rather than RenderableLayer.addRenderable
- Related question on World Wind forums
Hacky solution (requires modifying World Wind source code)
In gov.nasa.worldwind.render.DrawContextImpl
change orderedRenderables
from
protected PriorityQueue<OrderedRenderableEntry> orderedRenderables =
new PriorityQueue<OrderedRenderableEntry>(100, new Comparator<OrderedRenderableEntry>()
{
public int compare(OrderedRenderableEntry orA, OrderedRenderableEntry orB)
{
double eA = orA.distanceFromEye;
double eB = orB.distanceFromEye;
return eA > eB ? -1 : eA == eB ? (orA.time < orB.time ? -1 : orA.time == orB.time ? 0 : 1) : 1;
}
});
to
protected Queue<OrderedRenderableEntry> orderedRenderables = new ArrayDeque<>();
(or modify the comparator to do what you want). This will fix the drawing order.
Alternate solution (does not require modifying World Wind source code)
Extend PointPlacemark
and override makeOrderedRenderable()
:
public class OrderedPointPlacemark extends PointPlacemark {
public OrderedPointPlacemark(Position position) {
super(position);
}
@Override
protected void makeOrderedRenderable(DrawContext dc) {
super.makeOrderedRenderable(dc);
if (this.intersectsFrustum(dc) || this.isDrawLine(dc)) {
dc.pollOrderedRenderables();
dc.addOrderedSurfaceRenderable(this);
}
}
}