I want to do an equation using the values I get from the input boxes called ampMin, voltMin and hastMin...
I'm not sure if it's a syntax problem or my method of approach is plain wrong.. Here's is an example of how the equation should look and work like with Excel.
What am I doing wrong? Thank you for your time!
EDIT: worth mentioning is that the whole block of code is within "strckenergi.php".
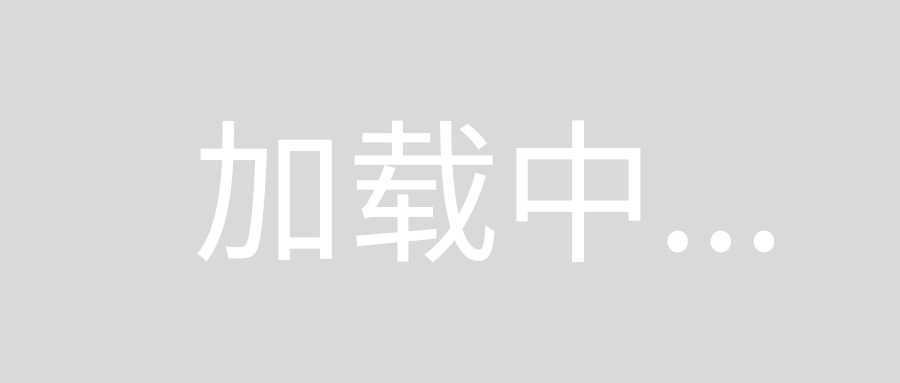
<html>
<title>Sträckenergi</title>
<body>
<h3>Svetsmetod: 111</h3>
<h4><i>Med K=0.8</i></h4>
<pre>
<form method="post" action="strckenergi.php">
Amp. Min <input type="text" name="ampMin"> Volt. Min <input type ="text" name="voltMin"> Hast. Min <input type="text" name="hastMin"> </pre>
<?php
echo "kJ/mm (minimum) = " . $qMin
$qMin = ( $ampMin * $voltMin ) / (( $hastMin * 1000 ) / $hastMin * 0.8));
?>
</body>
</html>
This works.
<html>
<title>Sträckenergi</title>
<body>
<h3>Svetsmetod: 111</h3>
<h4><i>Med K=0.8</i></h4>
<pre>
<form method="post" action="form.php">
Amp. Min <input type="text" name="ampMin"> Volt. Min <input type ="text" name="voltMin"> Hast. Min <input type="text" name="hastMin">
<input type="submit" value="submit"> </pre>
</form>
<?php
if($_POST){
$voltMin = $_POST['voltMin'];
$ampMin = $_POST['ampMin'];
$hastMin = $_POST['hastMin'];
$qMin = ( $ampMin * $voltMin ) / ( $hastMin * 1000 ) / $hastMin * 0.8;
echo "kJ/mm (minimum) = " . $qMin;
}
?>
You don't appear to be using forms correctly. If you want the browser do be able to display it right away, you should use JavaScript instead. PHP will require an additional pageload, or an AJAX request.
And in order to post the form, you need a submit button. Otherwise, the browser won't know what to do with it.
Further, your first PHP line needs a semi-colon, and your second one needs to be above the first - otherwise, the interpreter won't know what your value is when printing it, because it hasn't been calculated yet.
To be honest, I think you need to start by googling for how to construct an HTML form, then you can look up simple JavaScripts. Lycka till!
First off, as Joel Hinz says above, you need a submit button so the page knows when the user is done inputting and wants to send the form to the server for processing.
Second, you need to close the form with a tag.
Third, you're probably better off sticking with php at this stage; JavaScript can be a bit tricky and mighty frustrating for beginners.
This is a rough approximation of how your form should look.
<form method="post" action="strckenergi.php">
Amp. Min <input type="text" name="ampMin">
Volt. Min <input type ="text" name="voltMin">
Hast. Min <input type="text" name="hastMin">
<input type="submit" value="submit">
</form>
See http://www.tizag.com/phpT/forms.php for a clear explanation of forms.
OK first thing first. When the form is submitted, the values contained in the $_POST array aren't immediately available to the script on the server which processes the input.
For that you will need something like the following:
<?php
if($_POST){// this checks for the existence of the $_POST array, i.e. was something submitted
//now we're assuming a form was submitted
$voltMin = $_POST['voltMin'];
$ampMin = $_POST['ampMin'];
$hastMin = $_POST['hastMin'];
$qMin = ( ($ampMin*$voltMin)/($hastMin*1000)/($hastMin*0.8) );
echo "kJ/mm (minimum) = " . $qMin;
}// if($_POST)...
?>
Then you can process the elements, and print out the results of your calculations.
Oh, and drop the pre tags unless you really need them.