This is a small C code
#include<stdio.h>
int main()
{
char ch='Y';
while(ch=='Y')
{
printf("\nEnter new value for Y ");
scanf("%c",&ch);
if(ch=='Y' || ch=='y')
// do some work
printf("Y was selected\n");
}
printf("exiting main");
return 0;
}
I entered 'Y' as input (without qoutes) for the first time, the loop returned true and came inside for the second time, but this time it didn't stop on scanf() for user input.(Please execute with input as 'Y' for clarity). The output is shown below :
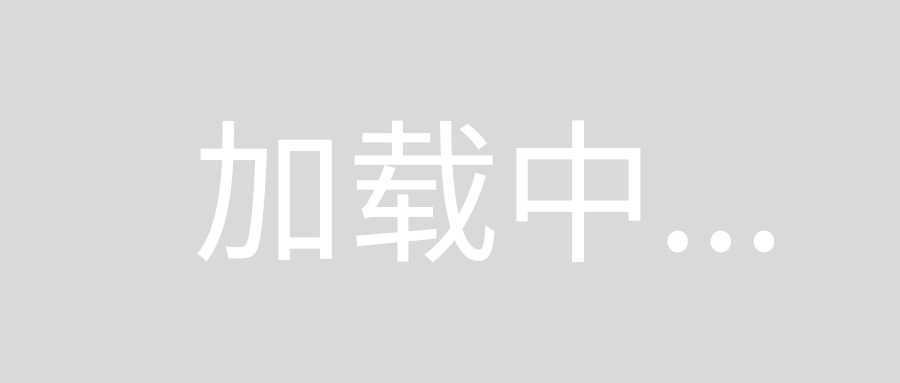
As shown here, the compiler didn't stop for input for the second time. What is the reason behind this. Thanks in advance !
Just put a space in the scanf like this:
scanf(" %c",&ch);
And the reason is you enter: Y\n
(with the enter)
scanf only reads Y
! So in the next scanf \n
is still in the buffer and get's read in!
So if you now have a space before you read something new it makes a cut (and it looks like this: '\n ')!
'\n'
automatically becomes the input for the next iteration.
To avoid this use getchar()
. It will take care of the unwanted '\n'
.
#include<stdio.h>
int main()
{
char ch='Y';
while(ch=='Y')
{
printf("\nEnter new value for Y ");
scanf("%c",&ch);
getchar(); //takes care of '\n'
if(ch=='Y' || ch=='y')
// do some work
printf("Y was selected\n");
}
printf("exiting main");
return 0;
}
the code works fine now. Below is the snippet from the command window.
Enter new value for Y Y
Y was selected
Enter new value for Y Y
Y was selected
Enter new value for Y _