I'm new to QT and am making a widget that interfaces with a pre-existing gui. I'd like to have my widget continuously output one signal while the user has a pushbutton pressed and then continuously output another when it is released.
By enabling autorepeat I can have the widget output my signal while the user is pressing the pushbutton, however, the output signal switches between pressed() and released(). E.G.
<>
Outputs:
* pressed signal
* released signal
* pressed signal
* released signal
I've seen this question been asked about keyPressEvents but I'm not sure how to access isAutoRepeat() for PushButtons. Can someone give me advice on this?
One way is you can use timer object to achieve this. Below is the example, that will run the 2 slot's when button pressed and released. The code comment will explain in detail. when button pressed & released a text box will show the continuous time in Milli-seconds. Timer is an object that will emit the timeout() signal in a given interval. We need to stop and start the alternate timers in button pressed / released signal. This application created using the QT Creator "QT Widgets Application" wizard.
Hope this help.
//Header File
class MainWindow : public QMainWindow{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private slots:
//Button slots
void on_pushButton_pressed(); //Continuous press
void on_pushButton_released(); //Continuous release
void on_pushButton_2_clicked(); //stop both the timer
//QTimer timeout actions
void timer1_action();
void timer2_action();
private:
Ui::MainWindow *ui;
//Timer object
QTimer *t1, *t2;
//Date time object for testing
QDateTime dt1,dt2;
};
//CPP file
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow){
ui->setupUi(this);
//Parent object will take care of the deallocation of the 2 timer objects
t1 = new QTimer(this);
t2 = new QTimer(this);
//Interval to the timer object
t1->setInterval(10);
t2->setInterval(10);
//Signal slot for the timer
this->connect(t1,SIGNAL(timeout()),this,SLOT(timer1_action()));
this->connect(t2,SIGNAL(timeout()),this,SLOT(timer2_action()));
}
MainWindow::~MainWindow(){
delete ui;
}
void MainWindow::on_pushButton_pressed(){
//starting and stoping the timer
t2->stop();
t1->start();
//date time when pressed
dt1 = QDateTime::currentDateTime();
}
void MainWindow::on_pushButton_released(){
//starting and stoping the timer
t1->stop();
t2->start();
//date time when pressed
dt2 = QDateTime::currentDateTime();
}
void MainWindow::timer1_action(){
ui->txtTimer1->setPlainText("Button Pressed for " + QString::number(dt1.msecsTo(QDateTime::currentDateTime())) + " Milli Seconds");
}
void MainWindow::timer2_action(){
ui->txtTimer2->setPlainText("Button Released for " + QString::number(dt2.msecsTo(QDateTime::currentDateTime())) + " Milli Seconds");
}
void MainWindow::on_pushButton_2_clicked(){
//stoping both the timer
t1->stop();
t2->stop();
}
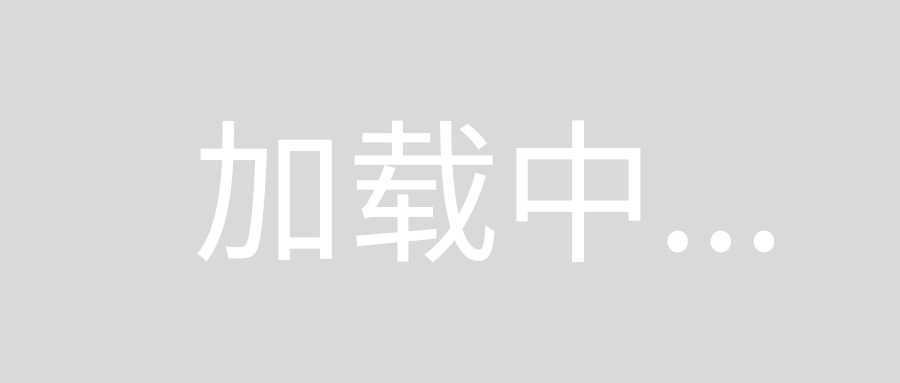