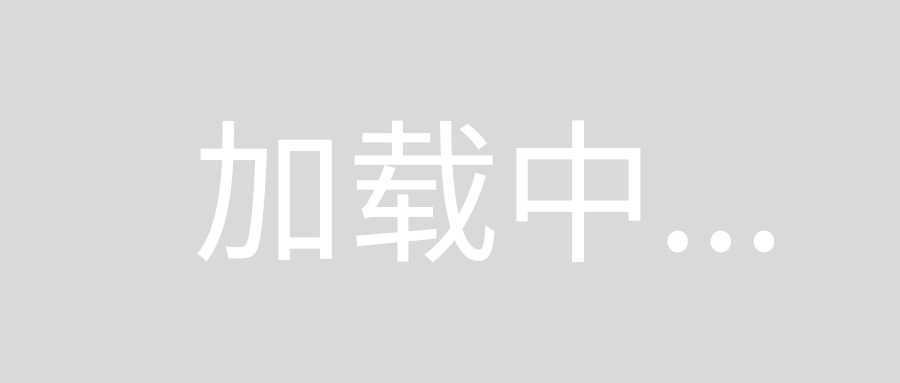
I need the search bar under the navigation title how to do that programmatically or via story board.
UISearchBar *searchBar = [[UISearchBar alloc]initWithFrame:CGRectMake(0,30, 250,44)];
searchBar.placeholder = @"Search";
UIBarButtonItem *searchBarItem = [[UIBarButtonItem alloc]initWithCustomView:searchBar];
searchBarItem.tag = 123;
searchBarItem.customView.hidden = YES;
searchBarItem.customView.alpha = 0.0f;
self.navigationItem.leftBarButtonItem = searchBarItem;
self.navigationItem.title = @"locations";
You have added the search bar as UIBarButtonItem
, thats why it is not visible.
If you want to display the search bar in place of the title view of navigation bar the use this code
Code to show navigation bar in place of Title of Navigation :
UISearchBar *searchBar = [[UISearchBar alloc]init];
searchBar.tintColor = [UIColor grayColor];
searchBar.backgroundColor = [UIColor redColor];
searchBar.placeholder = @"Search";
self.navigationItem.titleView = searchBar;
Output :
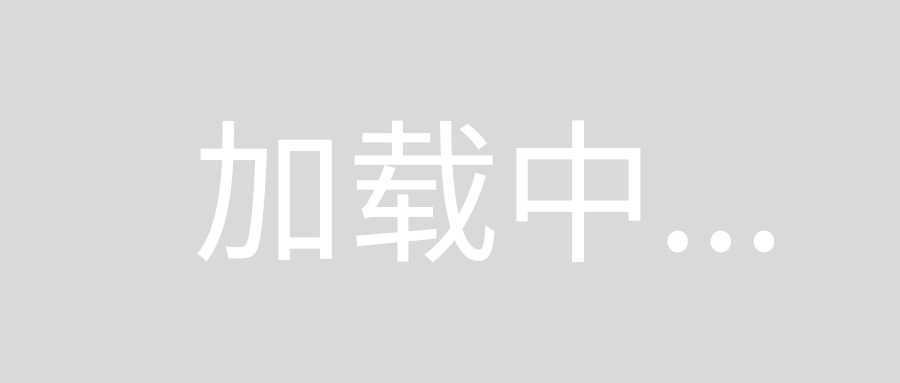
Code to show navigation bar below the Navigation bar:
UISearchBar *searchBar1 = [[UISearchBar alloc]initWithFrame:CGRectMake(0, 64, [UIScreen mainScreen].bounds.size.width, 64)];
searchBar1.tintColor = [UIColor grayColor];
searchBar1.backgroundColor = [UIColor redColor];
searchBar1.placeholder = @"Search";
[self.view addSubview:searchBar1];
UPDATE :
self.navigationController.navigationBar.barTintColor = [UIColor redColor];
searchBar1.searchBarStyle = UISearchBarStyleMinimal;
UISearchBar *searchBar1 = [[UISearchBar alloc]initWithFrame:CGRectMake(0, 64, [UIScreen mainScreen].bounds.size.width, 64)];
searchBar1.tintColor = [UIColor grayColor];
searchBar1.searchBarStyle = UISearchBarStyleMinimal;
searchBar1.backgroundColor = [UIColor redColor];
searchBar1.placeholder = @"Search";
[self.view addSubview:searchBar1];
[[UITextField appearanceWhenContainedIn:[UISearchBar class], nil] setDefaultTextAttributes:@{NSForegroundColorAttributeName:[UIColor whiteColor]}];
Output :
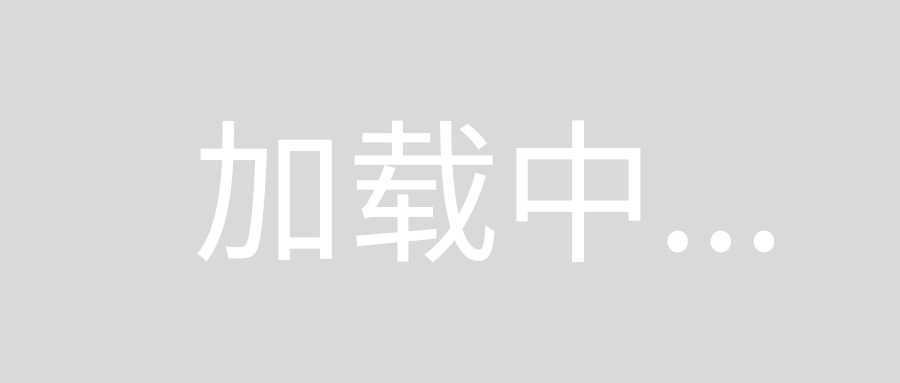
Updated output
To customize the textField :
UISearchBar *searchBar1 = [[UISearchBar alloc]initWithFrame:CGRectMake(0, 64, [UIScreen mainScreen].bounds.size.width, 64)];
searchBar1.tintColor = [UIColor grayColor];
searchBar1.searchBarStyle = UISearchBarStyleMinimal;
searchBar1.backgroundColor = [UIColor redColor];
searchBar1.placeholder = @"Search";
[self.view addSubview:searchBar1];
[searchBar1 setSearchFieldBackgroundImage:[UIImage imageNamed:@"text-input.png"] forState:UIControlStateNormal];
The image I have used is of height 30 pixel like the search bar's text field.
here is the image :
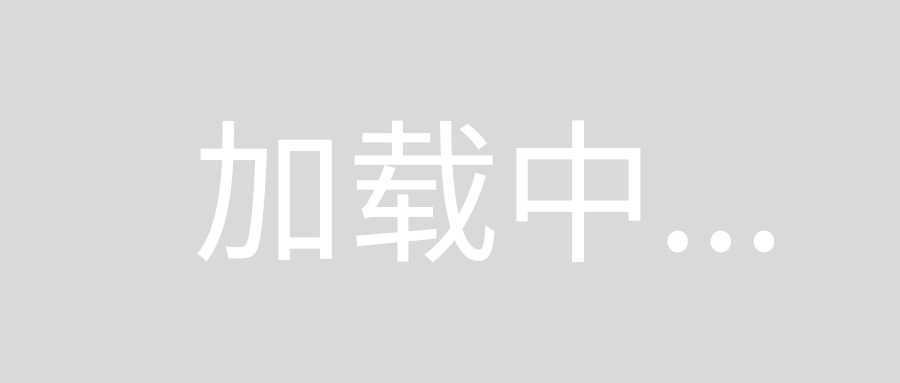
And output :
Hope it helps ...
Happy coding ...
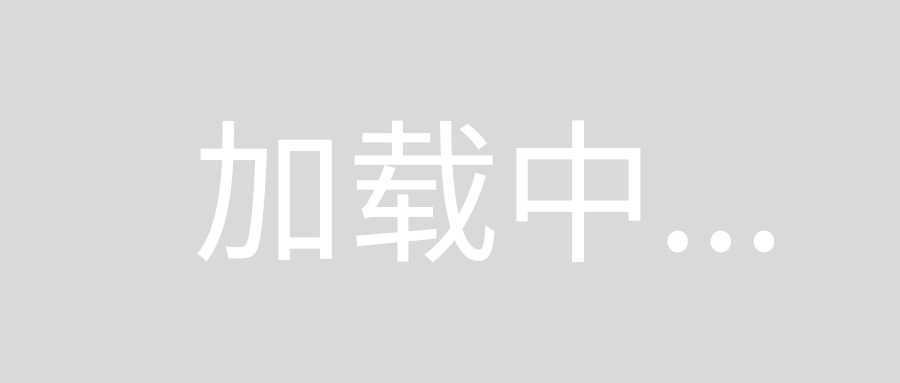
We can also use textbox as search bar, it is working fine in my app,
Go to StoryBoard
and do the following changes
From Object Library--> type view
and drag to storyboard
and paste below the Navigation bar.
Add the textbox to the view and assign the outlets and delegates.
now go to viewcontroller.m
and paste the following code
-(void)textFieldDidChange:(UITextField *)textField
{
searchTextString=textField.text;
[self updateSearchArray:searchTextString];
}
-(void)updateSearchArray:(NSString *)searchText
{
if(searchText.length==0)
{
isFilter=NO;
}
else
{
isFilter=YES;
searchArray=[[NSMutableArray alloc]init];
for(NSString *string in responceArray)
{
NSRange stringRange = [[NSString stringWithFormat:@"%@",string]
rangeOfString:searchtext.text options:NSCaseInsensitiveSearch];
if (stringRange.location != NSNotFound)
{
[searchArray addObject:string];
}
}
[jobsTable reloadData];
}
}
Now it will work fine..