I have data that looks like this:
Name Odds_Ratio_(log2) p-value
Ann -1.80494 0.05
Lucy 2.51017 0.1
Sally -1.97779 0.01
...
And I want to make graph that would look something like Figure B or C.
To have p-values on y-axis, odds ratio on x axis (divided by such vertical line) and Names next to plotted symbol. I don't have fractional size so it's impossible to change symbol size here.
How can I do it?
I really hope that someone will help me with this, as my R plotting knowledge lets me to make plots only like this:
plot(data$V2,data$V3, main="Ratio", xlab="p-value",ylab="Odds ratio (Log2)")
.
You should refer to ?plot
, ?abline
, and ?text
. That said, here's one way to do it with base functions.
d <- data.frame(Name=LETTERS, Odds_Ratio_log2=runif(26, -8, 8),
p_value=runif(26))
plot(d$Odds_Ratio_log2, d$p_value, pch=20, xlim=c(-8, 8), ylim=c(0, 1),
axes=F, xlab='', ylab='', yaxs='i')
abline(v=0, lwd=3)
axis(3, lwd=3, at=seq(-8, 8, 1), cex.axis=0.8, lwd.ticks=1)
mtext('Odds (Log2)', 3, line=2.5)
text(d$Odds_Ratio_log2, d$p_value, d$Name, pos=4, cex=0.7)
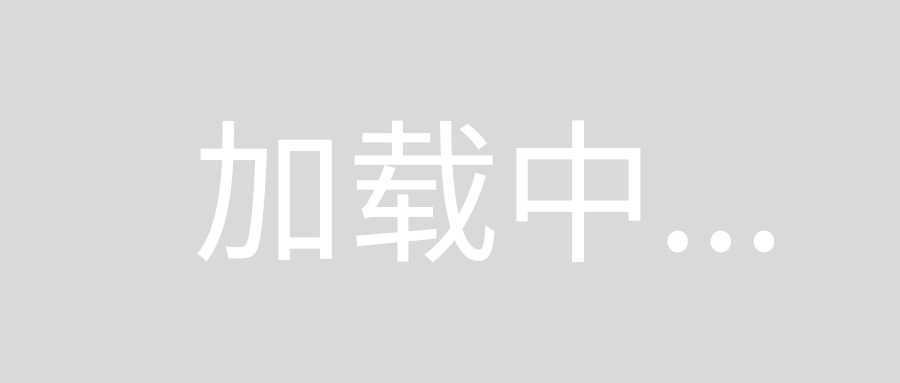
UPDATE:
I've added yaxs='i'
to the plot
call above, because unless we do this, the top axis intercepts slightly higher than y=1, perhaps giving an impression of lower p-values.
Note that the plot is not "rotated", per se. Rather, we suppress axis 1 and 2 (the default x and y axes), with axes=F
, and instead we plot axis 3 (the top axis; see ?axis
). We then use mtext
to plot the label for axis 3. To plot a label at the left edge of the plot, you can either use the ylab
argument (which I've set equal to ''
) in the plot
function, or use mtext('p-value', 2)
.
Alternatively, if you want the central vertical line to have ticks and labels corresponding to y-values, . Then, use segments
to add tick marks, and text
to add tick labels, e.g.:
segments(-0.1, seq(0, 1, 0.1), 0, seq(0, 1, 0.1), lwd=2)
text(rep(0, 10), seq(0, 1, 0.1), seq(0, 1, 0.1), cex=0.7, pos=2)
The end result will look something like this:
opar <- par(no.readonly = TRUE)
d <- data.frame(Name=LETTERS, Odds_Ratio_log2=runif(26, -8, 8),
p_value=runif(26))
plot(d$Odds_Ratio_log2, d$p_value, pch=20, xlim=c(-8, 8), ylim=c(0, 1),
axes=F, xlab='', ylab='', yaxs='i', col='gray20')
abline(v=0, lwd=3)
axis(3, lwd=3, at=seq(-8, 8, 1), cex.axis=0.8, lwd.ticks=1)
mtext('Odds (Log2)', 3, line=2.5)
text(d$Odds_Ratio_log2, d$p_value, d$Name, pos=4, offset=0.3, cex=0.7)
par(xpd=NA)
segments(-0.1, seq(0.1, 0.9, 0.1), 0, seq(0.1, 0.9, 0.1), lwd=2)
text(rep(0, 10), seq(0.1, 0.9, 0.1), seq(0.1, 0.9, 0.1),
cex=0.7, pos=2, offset=0.3)
par(opar)
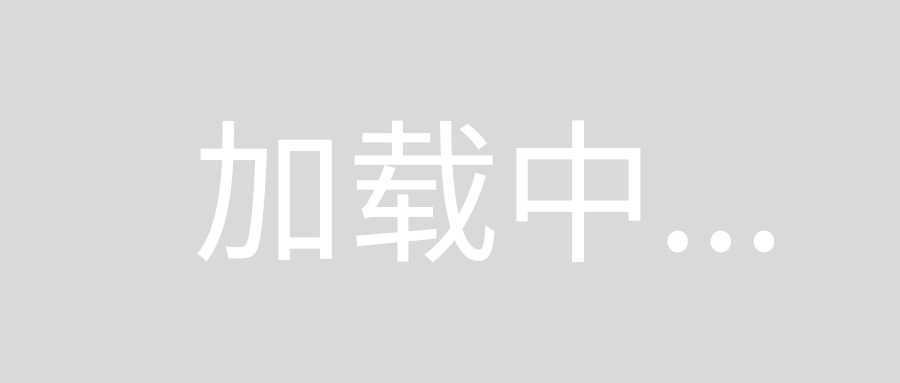