I'm creating calendar for few days events and the events can not overlap. But event can start/end when another event starts/ends.
In Laravel, in my event model table I am storing start and end date of events.
How can I check before storing new event do database, if it is valid (is not overlapping with existing events, excluding margin/boundary days) ?
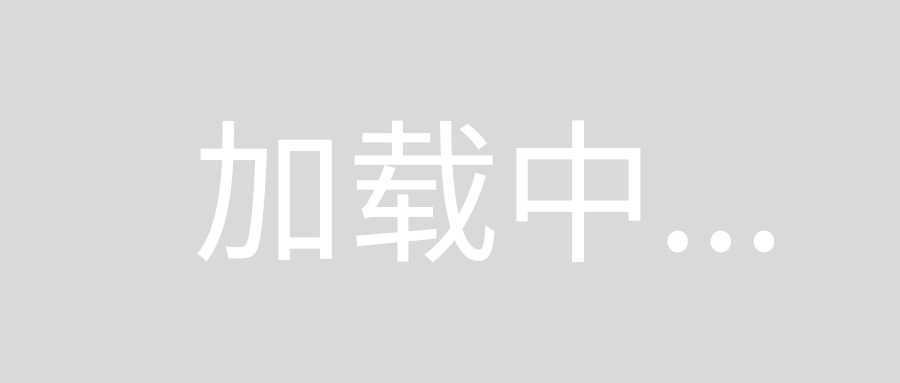
In advance thank You very much for Your help.
You can use following code to get the correct counts for events booked between certain times:
$eventsCount = Events::where(function ($query) use ($startTime, $endTime) {
$query->where(function ($query) use ($startTime, $endTime) {
$query->where('start', '>=', $startTime)
->where('end', '<', $startTime);
})
->orWhere(function ($query) use ($startTime, $endTime) {
$query->where('start', '<', $endTime)
->where('end', '>=', $endTime);
});
})->count();
This is just some pseudocode since you don't provide any code or your model, but depending on how your table is set up, you should be able to do it like this. This will return any events where the new event's start time or end time falls between an existing event's start and end times. This will work if your times are timestamps, or DateTime columns.
Events::where(DB::raw("? between start_time and end_time",$start))
->orWhere(DB::raw("? between start_time and end_time",$end))
->get();
Use it for all variants intersections date ranges. It help for me
$rangeCount = TransferSchedule::where(function ($query) use ($from, $to) {
$query->where(function ($query) use ($from, $to) {
$query->where('date_from', '<=', $from)
->where('date_to', '>=', $from);
})->orWhere(function ($query) use ($from, $to) {
$query->where('date_from', '<=', $to)
->where('date_to', '>=', $to);
})->orWhere(function ($query) use ($from, $to) {
$query->where('date_from', '>=', $from)
->where('date_to', '<=', $to);
});
})->count();