Has there ever been a performance difference in any of the java versions released to date between
foo != null
and
null != foo
?
I personally do not think there is any change in behavior of the above two forms of null check of an object, but not sure about which one performs better. I know the difference will be very low, if any, but was wondering why someone had written all the code in that fashion.
This came from old C times. And it started with foo == null (null == foo).
In c syntax was valid (compiler did not complain):
if (foo = null) {
}
To prevent this subtle error syntax was started to use:
if (null == foo) {
}
Because null is Constant this
if (null = foo) {
}
becomes syntax error.
In java there is only one case where this matters:
boolean someBoolean;
if (someBoolean = true) {
}
is runtime error, but compiles
This
boolean someBoolean;
if (true = someBoolean) {
}
is compile error.
There is no performance issues, only error prevention in some cases.
There should be no performance difference but form readability perspective foo != null is better. Because generally on the left side we put what we want to compare against the value on the right side. Generally we will be comparing our object like foo against null.
Equality is symmetric. So the order won't matter semantically.
Conventional style says you put the thing you're checking first and the constant last. This follows (at least many European) linguistic forms, e.g. "is x null?" not "is null x?".
At one point in history, people used to write if (NULL == x)
in C. This was so that the compiler would catch an accidental syntax error if (NULL = x)
by saying "you can't assign to a constant". Standard code quality tools can now pick up these types of errors, so this practise is now obsolete.
The fashion is about yoda style. This is only matter of personal preferences.
"Yoda Conditions"— using if (constant == variable) instead of if
(variable == constant), like if (4 == foo). Because it's like saying
"if blue is the sky" or "if tall is the man".
More on SO
No there is no difference between the two forms.
No matter what, there are the following instructions - load the value of foo, compare with 0 (null) and read the comparison result.
Now, if it was the equals() method, that would be different :)
public class Stack
{
public static void main(String[] args)
{
Object obj0 = null;
Object obj1 = new Object();
long start;
long end;
double difference;
double differenceAvg = 0;
for (int j = 0; j < 100; j++)
{
start = System.nanoTime();
for (int i = 0; i < 1000000000; i++)
if (obj0 == null);
end = System.nanoTime();
difference = end - start;
differenceAvg +=difference;
}
System.out.println(differenceAvg/100);
differenceAvg = 0;
for (int j = 0; j < 100; j++)
{
start = System.nanoTime();
for (int i = 0; i < 1000000000; i++)
if (null == obj0);
end = System.nanoTime();
difference = end - start;
differenceAvg +=difference;
}
System.out.println(differenceAvg/100);
differenceAvg = 0;
for (int j = 0; j < 100; j++)
{
start = System.nanoTime();
for (int i = 0; i < 1000000000; i++)
if (obj1 == null);
end = System.nanoTime();
difference = end - start;
differenceAvg +=difference;
}
System.out.println(differenceAvg/100);
differenceAvg = 0;
for (int j = 0; j < 100; j++)
{
start = System.nanoTime();
for (int i = 0; i < 1000000000; i++)
if (null == obj1);
end = System.nanoTime();
difference = end - start;
differenceAvg +=difference;
}
System.out.println(differenceAvg/100);
}
}
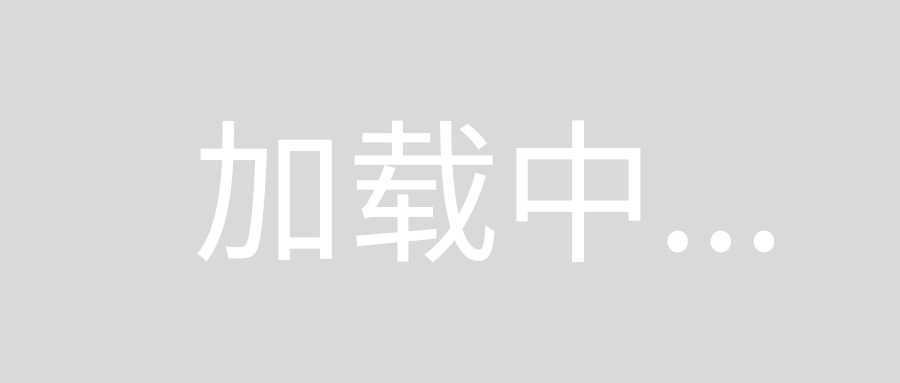
In the case when the Object
was initialized, nothing is gleaned; however, when the Object
is null
, in almost every average case run, obj0 == null
was faster than null == obj0
. I ran 21 additional executions, and in 20 of them this was the case.