I am trying to implement the Rotatory wheel in android, just like the image displayed below.I came across the tutorial from this link. But i want to implement just as shown in the below image.The wheel consists of individual images.Does anybody have any idea regarding this implementation?? Any help would be appreciated.
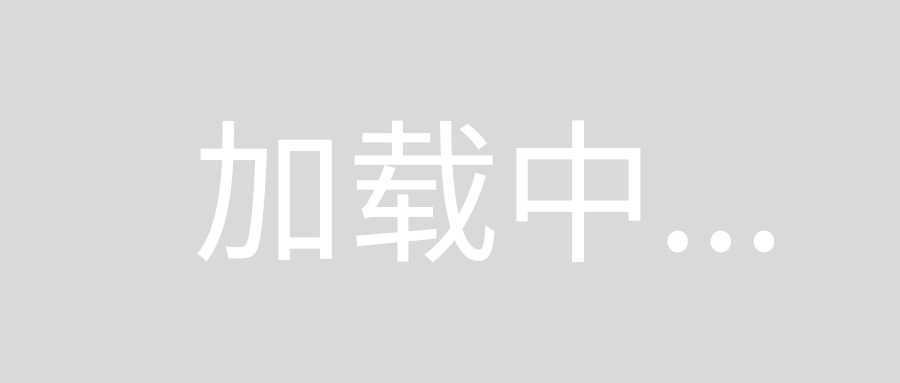
Thanks in advance.
Akash
To do this from scratch, you would need a way to transform your touch coordinates, into polar coordinates (to have the rotation angle). This can be done easily like this:
private float cartesianToPolar(float x, float y) {
return (float) -Math.toDegrees(Math.atan2(x - 0.5f, y - 0.5f));
}
To rotate the imageview, or the element you are using to display your knob, you can use a matrix like this:
Matrix matrix=new Matrix();
ivRotor.setScaleType(ScaleType.MATRIX);
matrix.postRotate((float) deg, m_nWidth/2, m_nHeight/2);//getWidth()/2, getHeight()/2);
ivRotor.setImageMatrix(matrix);
Where deg is the angle and ivRobor is the knob imageview.
A complete working sample for Android, is available on Google code at: https://code.google.com/p/android-rotaryknob-view/
Applied on OnTouchListener on the imageView through which i got three events namely:
- MotionEvent.ACTION_DOWN,
- MotionEvent.ACTION_MOVE &
- MotionEvent.ACTION_UP.
On MotionEvent.ACTION_DOWN got the angle where the users touches and on MotionEvent.ACTION_UP got the angle where user releases.
After getting difference of the two angles,rotated the image of that angle.
After rotating the image checked the quadrant through angle and maintained int variable which incremented according to the quadrant and by fulfilling the condition set the new image(the desired one).
Maintained the click event according to the value of the int variable.
Check this out. Its simple and basic wheel implementation on android. but you can develop on top of that.
https://github.com/lib4/RotateWheelAndroid_lib4
Here is the complete code for this:
import android.animation.ObjectAnimator;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Color;
import android.graphics.drawable.ColorDrawable;
import android.os.Bundle;
import android.graphics.Matrix;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.LinearInterpolator;
import android.view.animation.RotateAnimation;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.Toast;
import java.util.Random;
public class MainActivity extends Activity{
// Button rotate;
ImageView i;
ImageView ii;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
i= (ImageView) findViewById(R.id.i);
i.setImageResource(R.drawable.gg);
ii= (ImageView) findViewById(R.id.ii);
ii.setImageResource(R.drawable.gg);
// i.setBackgroundColor(Color.rgb(255, 255, 255));
}
public void ii(View v)
{
RotateAnimation rotate =
//new RotateAnimation(0f,generateRandomNumber(),55f,55f);
new RotateAnimation(0, generateRandomNumber(),
Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
rotate.setDuration(1500);
rotate.setInterpolator(new LinearInterpolator());
i.startAnimation(rotate);
i.setRotation(generateRandomNumber());
RotateAnimation rotate1 =
//new RotateAnimation(0f,generateRandomNumber(),55f,55f);
new RotateAnimation(0, 999999999, Animation.RELATIVE_TO_SELF,
0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
rotate1.setDuration(99999);
rotate1.setInterpolator(new LinearInterpolator());
// i= (ImageView) findViewById(R.id.i);
// i.setImageResource(R.drawable.g);
ii.startAnimation(rotate1);
/*i= (ImageView) findViewById(R.id.i);
i.setImageResource(R.drawable.g);
ObjectAnimator animator = ObjectAnimator.ofFloat(i,"rotationY", 360f);
animator.setDuration(1000);
animator.start();
*/
/* Matrix matrix = new Matrix();
i.setScaleType(ImageView.ScaleType.CENTER_INSIDE); //required
matrix.postRotate(generateRandomNumber());
i.setImageMatrix(matrix);
*/
/* Matrix matrix = new Matrix();
Bitmap bMap = BitmapFactory.decodeResource(getResources(),
R.drawable.g);
matrix.postRotate(generateRandomNumber());
Bitmap bMapRotate = Bitmap.createBitmap(bMap, 0,
0,bMap.getWidth(),bMap.getHeight(), matrix, true);
i.setImageBitmap(bMapRotate);*/
}
public float generateRandomNumber() {
Random rand = new Random();
int randomNum = rand.nextInt((10000000 - 125000) + 1);
return (float)randomNum;
}
int backpressed=0;
@Override
public void onBackPressed() {
backpressed++;
if(backpressed>1)
{
super.onBackPressed();
finish();
}
else
{
Toast.makeText(this, "Press back again to exit",
Toast.LENGTH_LONG).show();
new Thread(new Runnable() {
@Override
public void run() {
try{
Thread.sleep(2000);}
catch (Exception e)
{
e.printStackTrace();
}
backpressed=0;
}
});
}
}
}
Here is the XML:
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=".............."
android:textColor="#00ff10"
android:textStyle="bold"
android:textSize="25dp"
android:layout_gravity="center"/>
<ImageView
android:layout_width="200dp"
android:layout_height="80dp"
android:layout_gravity="center"
android:src="@drawable/a"
/>
<ImageView
android:layout_width="300dp"
android:layout_height="300dp"
android:layout_gravity="center"
android:id="@+id/i"
/>
<DigitalClock
android:layout_width="100dp"
android:layout_height="50dp"
android:layout_gravity="center"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play"
android:backgroundTint="#ff0044"
android:id="@+id/rotate"
android:layout_gravity="center"
android:onClick="ii"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="powered by ashwani"
android:textColor="#00ff10"
android:textStyle="bold"
android:layout_gravity="center"/>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center"
android:id="@+id/ii"
/>