I was recently given with the task of completely revising one of my company's software Gui appearance.
Up until now I only done basic look-n-feel changes. Mostly upgrading the Gui from the default Metal L&F to System L&F with some GridBagConstraints handling.
But this task is something different:
We have a graphic designer who drew the desired design:
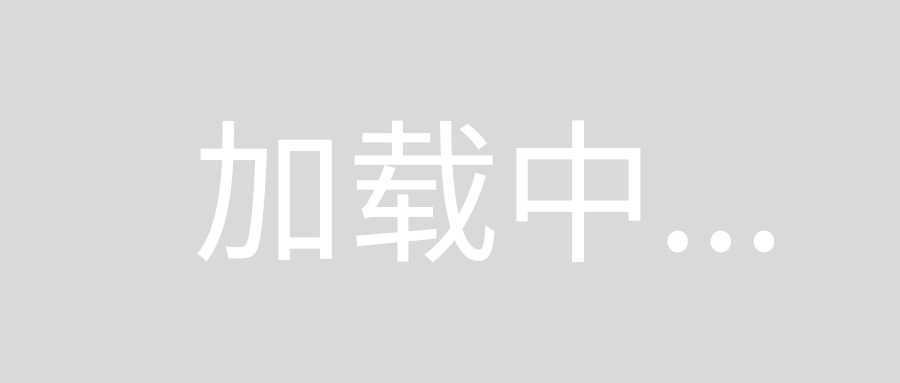
I know how to create this kind of functionality. I might use a double JTabbedPanes or just add Jpanels all around.
My problem is how to incorporate the graphics with gui objects.
What should I ask the designer to give me in order to make a Java Swing application that looks like this - with the rounded tabs and the merging of tab-panel colors?
How can I make JPanels or a JTabbedPanes wear this fancy costume when all I am given right now is this drawing above?
Most custom Swing GUIs are made using JLabels with ImageIcons and custom handling code. For example:
public class CustomGUI extends JFrame {
private JLabel frame, border, grip, exit, minimize;
private Point initialClick;
public CustomGUI() {
initComponents();
}
private void initComponents() {
setTitle("CustomGUI");
setMinimumSize(new Dimension(640, 480));
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setUndecorated(true);
setBackground(new Color(0, 0, 0, 0));
setLayout(null);
pack();
frame = new JLabel();
frame.setBounds(0, 0, 640, 480);
frame.setIcon(new ImageIcon(getClass().getResource("frame.png"));
border = new JLabel();
border.setBounds(0, 0, 640, 480);
border.setIcon(new ImageIcon(getClass().getResource("border.png"));
grip = new JLabel();
grip.setBounds(215, 0, 376, 25);
grip.setIcon(new ImageIcon(getClass().getResource("grip.png"));
grip.addMouseListener(new MouseAdapter() {
@Override
public void mousePressed(MouseEvent evt) {
initialClick = evt.getPoint();
}
});
grip.addMouseMotionListener(new MouseMotionAdapter() {
@Override
public void mouseDragged(MouseEvent evt) {
int thisX = getLocation().x;
int thisY = getLocation().y;
int xMoved = (thisX + evt.getX()) - (thisX + initialClick.x);
int yMoved = (thisY + evt.getY()) - (thisY + initialClick.y);
int posX = thisX + xMoved;
int posY = thisY + yMoved;
setLocation(posX, posY);
}
});
exit = new JLabel();
exit.setBounds(620, 4, 32, 16);
exit.setIcon(new ImageIcon(getClass().getResource("exit.png"));
exit.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent evt) {
dispatchEvent(new WindowEvent(new UI(), WindowEvent.WINDOW_CLOSING));
}
@Override
public void mouseEntered(MouseEvent evt) {
exit.setIcon(new ImageIcon(getClass().getResource("exitGlow.png"));
}
@Override
public void mouseExited(MouseEvent evt) {
exit.setIcon(new ImageIcon(getClass().getResource("exit.png"));
}
});
minimize = new JLabel();
minimize.setBounds(600, 4, 16, 16);
minmize.setIcon(new ImageIcon(getClass().getResource("minimize.png"));
minimize.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent evt) {
setState(Frame.ICONIFIED);
}
@Override
public void mouseEntered(MouseEvent evt) {
minimize.setIcon(new ImageIcon(getClass().getResource("minimizeGlow.png"));
}
@Override
public void mouseExited(MouseEvent evt) {
minimize.setIcon(new ImageIcon(getClass().getResource("minimize.png"));
}
});
add(minimize);
add(exit);
add(grip);
add(border);
add(frame);
}
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
new CustomGUI().setVisible(true);
});
}
}
This code creates a custom GUI with a custom frame, border, and a grip for dragging the window, including exit and minimize buttons that glow when your mouse enters them, making them look proffesional. (The images for each component need to be in the root of the project for this to work.) If you want to create custom tabbed panes, create JLabels
with ImageIcons
that look like rounded tabs or anything that you want them to look like, each with its corresponding text on it, and add handling that will make it look selected by changing its color or adding a border around it and bring its corresponding panel to the front, hiding all other panels.
Alternatively, you can create a custom Look and Feel, but this is harder to do and does not give you the freedom that using JLabels
with ImageIcons
does.
You can also use JavaFX, a rich GUI platform that was designed to replace Swing, but as far as Swing goes, the above code is your best bet for a Swing application.