<?php
require_once('facebook.php');
$config = array(
'appId' => 'xxxxx',
'secret' => 'xxxxx',
);
$facebook = new Facebook($config);
$user_id = $facebook->getUser();
?>
<html>
<head></head>
<body>
<?php
if($user_id) {
try {
$user_profile = $facebook->api('/me','GET');
echo "Name: " . $user_profile['name'];
} catch(FacebookApiException $e) {
$login_url = $facebook->getLoginUrl();
echo 'Please <a href="' . $login_url . '">login.</a>';
echo "1";
error_log($e->getType());
error_log($e->getMessage());
}
} else {
$login_url = $facebook->getLoginUrl();
echo 'Please <a href="' . $login_url . '">login.</a>';
echo "2";
}
?>
</body>
</html>
When I go to the mysite.com/fbconect.php, it is a white page with a link LOGIN. When i click login, it is going to the facebook page login. After I Logged In, i go back to mysite.com/fbconect.php then It displays, Login link again with Echoed "1". The URL of mysite.com became mysite.com/fbconect.php?xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx. What is wrong in my code?
There is nothing wrong in your code.
Be sure:
- The facebook user used for the test has authorized the app
- This code just run into a PHP page deployed on the website specified in both:
Facebook Developers
site -> App > YourApp > Basic
-> App Domains
Facebook Developers
site -> App > YourApp > Basic
-> Website with Facebook Login
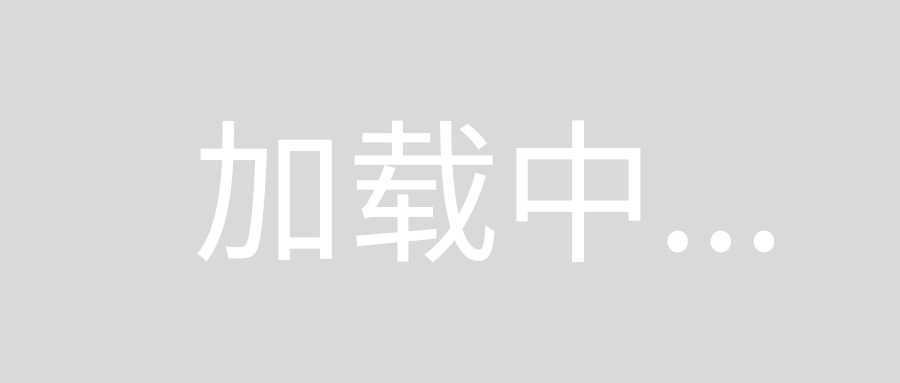
Maybe you are logged in with a user that has not already accepted your fb app.
In this case you will see "Please login.1"
If you'll see "Please login.2", sure you're code have caught and exception.
For example if your read this:
OAuthException
Error validating access token: User YYYYYYYY has not authorized application XXXXXXXXXXXXXXXX.
very likely your fb user have removed your app from his/her list.
Or if you see
Couldn't connect to host
This means the system you're working on most likely blocks outgoing connections.
If you want a test user that has already accepted your fb app, you can create one from Facebook Developers
site go into "Apps > YourApp > Developer Roles
"
In case you want get authorization from the user you can change your code:
<?php
require_once('facebook.php');
$config = array(
'appId' => 'xxxxx',
'secret' => 'yyyyyy',
);
$facebook = new Facebook($config);
$user_id = $facebook->getUser();
?>
<html>
<head></head>
<body>
<?php
function render_login($facebook) {
$canvas_page = 'http://www.yoursite.com/';
// HERE YOU ASK THE USER TO ACCEPT YOUR APP AND SPECIFY THE PERMISSIONS NEEDED BY
$login_url = $facebook->getLoginUrl(array('scope'=>'email,user_photos,friends_photos', 'redirect_uri'=>$canvas_page));
echo 'Please <a href="' . $login_url . '">login.</a>';
}
if($user_id) {
try {
$user_profile = $facebook->api('/me','GET');
echo "Name: " . $user_profile['name'];
} catch(FacebookApiException $e) {
render_login($facebook);
echo "1";
error_log($e->getType());
error_log($e->getMessage());
}
} else {
render_login($facebook);
echo "2";
}
?>
</body>
</html>
Hope this helps.