I am looking at using XCUITest for UI tests for my iOS apps. It looks like XCUITest has a wide arrange of functionality, including the ability to work with multiple apps. However, the multiple app support seems somewhat limited.
It seems that using XCUIApplication I can start another app using Bundle ID and even monitor its state. However, what I want to do is be able to run a coordinated test for two apps that have tight interaction (for example, one app does an open URL to another app, performs some UI action, and then returns to the first app).
Is this possible just using XCUITest, or do I need some higher level tool? (I think some tools exist that can do this but would prefer to stay with XCUITest if possible)
Ideally, I would have all the code in a single file that executed the UI actions in the two apps. But if that was impossible, I would be open to writing to separate test apps which effectively hand off to one other, performing actions when it is their 'turn'. But I am not sure how I would work the coordination of the two tests apps.
This is actually supported really nicely in Xcode 9. Here's how to set it up:
I've found the easiest is to create a workspace containing both of your apps. In the app project containing your UI test code to excercise both apps, make sure both apps are listed under Target Dependencies in your UI Test target:
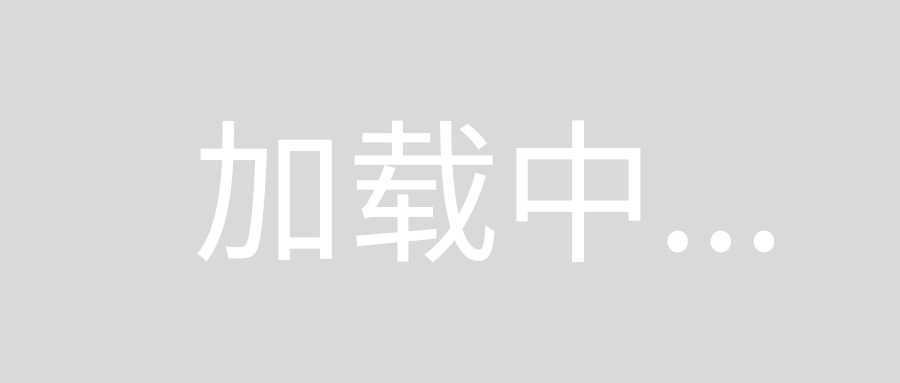
Now it's just a matter of starting both apps in your setup function of your test, using their bundle identifiers:
app1 = XCUIApplication(bundleIdentifier: "no.terje.app1.App1")
app1.launch()
app2 = XCUIApplication(bundleIdentifier: "no.terje.app2.App2")
app2.launch()
(the apps are instance members of my test class, var app1: XCUIApplication!
)
Now you're all set up. Run tests against both apps like this:
func testButtonsExist() {
app1.activate()
app1.buttons["mybutton"].exists
app2.activate()
app2.buttons["myotherbutton"].exists
}
As for the waiting or async challenges you are mentioning: I would imagine most of those exist for async code within a single app too, for example waiting for a URL to open. But do post a specific thing you are trying and failing if you have specific problems.