I'm trying to populate a select input/ form dropdown with the data from the table.
HTML
<select id="room_type" name="inputInfo" >
<option value="<?php echo set_value('room_type', '$data_room_type'); ?>"></option>
</select>
Model:
function get_room_details($data_room_type) { // line 19
$data_room_type = array();
$this->db->select('room_type', 'default_price');
$this->db->from('room_type');
$query = $this->db->get();
if ($query->num_rows() > 0) {
foreach ($query->result_array() as $row) {
$data_room_type[] = $row;
}
}
return $data_room_type;
}
Controller:
function index() {
$this->load->view('/main/new_reservation');
$this->load->model('reservations_model');
$this->reservations_model->get_room_details($data_room_type);
}
But I received this error:
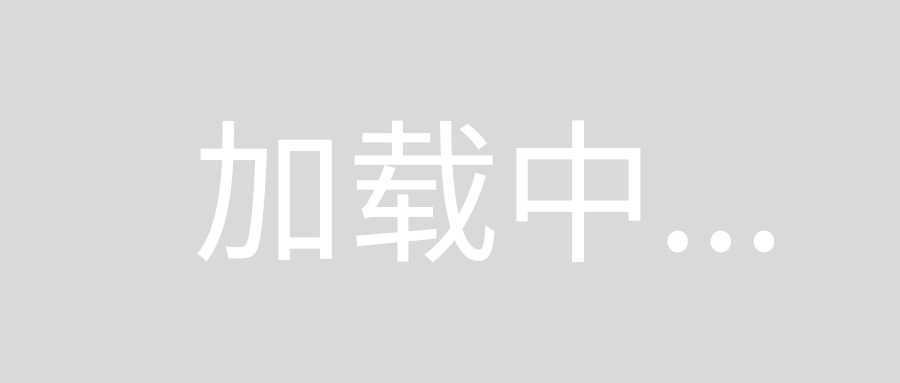
A PHP Error was encountered
Severity: Notice
Message: Undefined variable: data_room_type
Filename: controllers/reservations.php
Line Number: 8
Line number 8 is::
$this->reservations_model->get_room_details($data_room_type);
return $query->result_array(); line will populate more than one row for you.(if you have more than one record in room_type table)
so you need to run a foreach loop in view.for normal html
<select name="">
<?php
foreach($room_type as $each)
{
?>
<option value="<?=$each['rt_name']?>"><?=$each['rt_name']?></option>
<?php
}
?>
</select>
use normal html otherwise you need to change the return data what your model returning.
May be it will help you.Please let me know if you face any problem.
You have the syntax error means you have make something wrong. and as I can see that you have write the spelling of function wrong in model.
It is fucntion
you have write and it should be function
so change there and enjoy.
fucntion get_room_details($data_room_type) {
typo function
it should be
function get_room_details($data_room_type) {
$data_room_type[] = $row
. Missing semicolon
It should be $data_room_type[] = $row;
You can do like this
In Model : function get_room_details($data_room_type = array()) {
In Controller : $this->reservations_model->get_room_details();
This almost worked:
Model:
function pop_room_type() {
$this->db->select('rt_name')->from('room_type');
$query=$this->db->get();
return $query->result_array();
}
Controller:
function index() {
$this->load->model('reservations_model');
$data['room_type'] = $this->reservations_model->pop_room_type();
//echo "<pre>";print_r($data['room_type']);echo"</pre>";
$this->load->view('/main/new_reservation', $data);
}
View:
<?php
//$js = 'name='$room_type'';
echo form_dropdown('room_type', $room_type);
?>
However, there's a one BIG issue whereas the "name" of all select
options are the same (rt_name: as in the table's column).