How can I get the border width of a stand Android button programmatically? I simply need to resize the text to fit to the gray area and need to position other objects inline with the buttons, but I cannot do that without knowing the size of the border. I need this to work in all API's 7+. The red arrows in the image below show what I am trying to get:
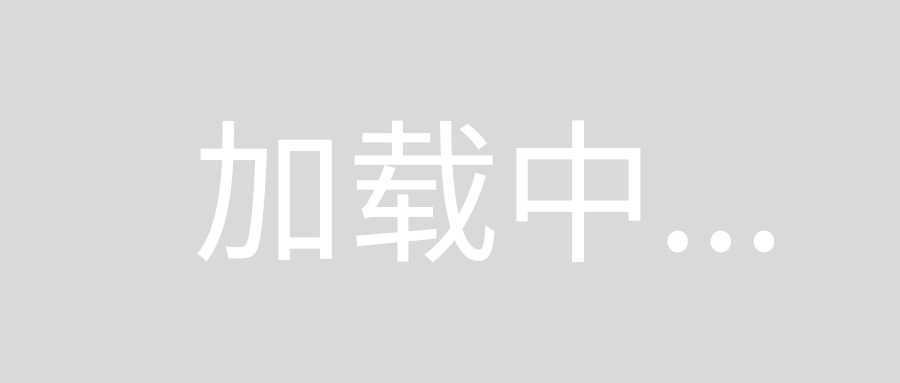
Here is the code I use for creating my button:
cmdView = new Button(this);
params = new RelativeLayout.LayoutParams(widthLblViewVerbs , (int) fieldHeight);
params.leftMargin = (int) (screenWidth - params.width);
params.topMargin = (int) yPos;
cmdView.setSingleLine();
cmdView.setText("View");
cmdView.setPadding(0, 0, 0, 0);
cmdView.setTextSize(TypedValue.COMPLEX_UNIT_PX, SetTextSize(cmdView.getText().toString(), params.width, params.height));
layout.addView(cmdView, params);
NB. I've had to ask this question again because someone downvoted my question last time and I am desperate for a solution. I have made absolutely no progress with my program in weeks because I have been stuck with this and another problem. If there is something unclear about my question, please let me know and I will edit it. Thank you
My initial code recommendation from the comments can be found here. The implementation done by Dan Bray is:
final Button button = new Button(this);
params = new RelativeLayout.LayoutParams(50, 50);
layout.addView(button, params);
button.post(new Runnable()
{
@Override
public void run()
{
button.buildDrawingCache();
Bitmap viewCopy = button.getDrawingCache();
boolean stillBorder = true;
PaddingLeft = 0;
PaddingTop = 0;
while (stillBorder)
{
int color = viewCopy.getPixel(PaddingLeft, button.getHeight() / 2);
if (color != Color.TRANSPARENT)
stillBorder = false;
else
PaddingLeft++;
}
stillBorder = true;
while (stillBorder)
{
int color = viewCopy.getPixel(button.getWidth() / 2, PaddingTop);
if (color != Color.TRANSPARENT)
stillBorder = false;
else
PaddingTop++;
}
}
});
Not really clear on the question - you want the margins of the underlying view and then the set the size of the button to match ? Then Have you tried
ViewGroup.MarginLayoutParams lp = (ViewGroup.MarginLayoutParams) view.getLayoutParams();
margins are accessible via
lp.leftMargin;
lp.rightMargin;
lp.topMargin;
lp.bottomMargin;
Details here:http://developer.android.com/reference/android/view/ViewGroup.MarginLayoutParams.html
This gets the width and height of the button's border:
final Button button = new Button(this);
params = new RelativeLayout.LayoutParams(50, 50);
layout.addView(button, params);
button.post(new Runnable()
{
@Override
public void run()
{
button.buildDrawingCache();
Bitmap viewCopy = button.getDrawingCache();
boolean stillBorder = true;
PaddingLeft = 0;
PaddingTop = 0;
while (stillBorder)
{
int color = viewCopy.getPixel(PaddingLeft, button.getHeight() / 2);
if (color != Color.TRANSPARENT)
stillBorder = false;
else
PaddingLeft++;
}
stillBorder = true;
while (stillBorder)
{
int color = viewCopy.getPixel(button.getWidth() / 2, PaddingTop);
if (color != Color.TRANSPARENT)
stillBorder = false;
else
PaddingTop++;
}
}
});
Assuming you are using a nine patch background image, there is a much easier way.
In the nine patch image, you can define a padding box. This padding box will automatically be applied to the contents you set. No need to recalculate the paddings manually in that case.
Note that you should not set a padding on views using a nine patch as a background as the padding from the nine patch will be ignored.