Is there a way to know in SQL Server 2008R2 if a point is at the south,east,etc...of another point?
For example, I have an origin point(lat1,lng1)
and I want to know where point(lat2,lng2)
is located from that origin: north, west,etc...
I'm trying to construct a wind rose graph and this might be useful to me.
In order to calculate the bearing between two coordinates while using the Geography type in SQL Server 2008 R2, you can use this function:
CREATE FUNCTION [dbo].[CalculateBearing]
(
@pointA as geography
,@pointB as geography
)
RETURNS decimal(18,12)
AS
BEGIN
-- Declare the return variable
DECLARE @bearing decimal(18,12)
-- Declare the local variables
DECLARE @x decimal(18,12)
DECLARE @y decimal(18,12)
DECLARE @dLat decimal(18,12)
DECLARE @dLong decimal(18,12)
DECLARE @rLat1 decimal(18,12)
DECLARE @rLat2 decimal(18,12)
IF(@pointA.STIsEmpty() = 1 OR @pointB.STIsEmpty() = 1)
set @bearing = null
ELSE
BEGIN
-- Calculate delta between coordinates
SET @dLat = RADIANS(@pointB.Lat - @pointA.Lat)
SET @dLong = RADIANS(@pointB.Long - @pointA.Long)
-- Calculate latitude as radians
SET @rLat1 = RADIANS(@pointA.Lat)
SET @rLat2 = RADIANS(@pointB.Lat)
SET @y = SIN(@dLong)*COS(@rLat2)
SET @x = COS(@rLat1)*SIN(@rLat2)-SIN(@rLat1)*COS(@rlat2)*COS(@dLong)
IF (@x = 0 and @y = 0)
SET @bearing = null
ELSE
BEGIN
SET @bearing = CAST((DEGREES(ATN2(@y,@x)) + 360) as decimal(18,12)) % 360
END
END
-- Return the result of the function
RETURN @bearing
END
GO
And after this, you can use this function like this:
DECLARE @pointA as geography
DECLARE @pointB as geography
SET @pointA = geography::STGeomFromText('POINT(3 45)', 4326)
SET @pointB = geography::STGeomFromText('POINT(4 47)', 4326)
SELECT [dbo].[CalculateBearing](@pointA, @pointB)
UPDATE: Adding a schema
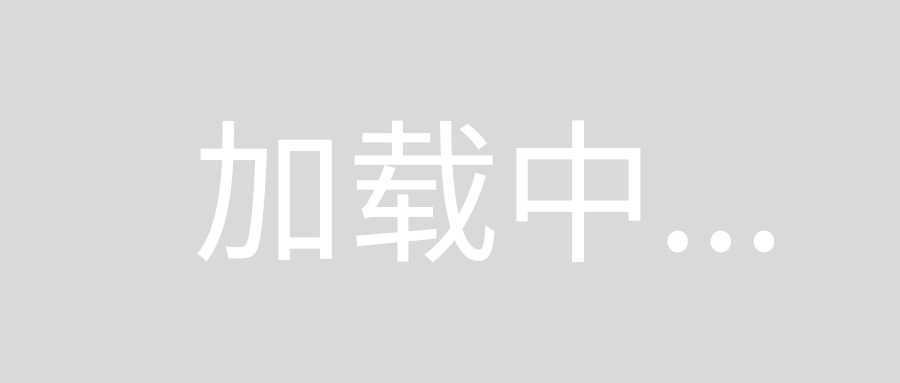
I came up with a way of calculating the bearing using a fairly straightforward use of standard SQL functions. The ATAN function does most of the real work; the two CASE statements are just special case corrections. 1 is the source and 2 is the destination.
atan(([Longitude2]-[Longitude1])/(10e-10+[Latitude2]-[Latitude1]))*360/pi()/2
+case when [Latitude2]<[Latitude1] then 180 else 0 end
+case when [Longitude2]<[Longitude1] and [Latitude2]>[Latitude1] then 360 else 0 end
This morning I had need for this functionality to provide the range and cardinal direction to users when searching for nearby orders in our system. I came to Nicolas's answer here and it got me most of the way there. I created a second function that uses Nicolas's to get myself an abbreviated cardinal direction (N, NE, E, etc) for my UI.
Using Nicolas's bearing calculation provided here combined with values from https://en.wikipedia.org/wiki/Points_of_the_compass to determine ranges for each cardinal direction,
CREATE FUNCTION [dbo].[CalculateCardinalDirection]
(
@pointA as geography
,@pointB as geography
)
RETURNS varchar(2)
AS
BEGIN
DECLARE @bearing decimal(18,12)
-- Bearing calculation provided by http://stackoverflow.com/a/14781032/4142441
SELECT @bearing = dbo.CalculateBearing(@pointA, @pointB)
RETURN CASE WHEN @bearing BETWEEN 0 AND 22.5 THEN 'N'
WHEN @bearing BETWEEN 22.5 AND 67.5 THEN 'NE'
WHEN @bearing BETWEEN 67.5 AND 112.5 THEN 'E'
WHEN @bearing BETWEEN 112.5 AND 157.5 THEN 'SE'
WHEN @bearing BETWEEN 157.5 AND 202.5 THEN 'S'
WHEN @bearing BETWEEN 202.5 AND 247.5 THEN 'SW'
WHEN @bearing BETWEEN 247.5 AND 292.5 THEN 'W'
WHEN @bearing BETWEEN 292.5 AND 337.5 THEN 'NW'
ELSE 'N' -- Catches NULL bearings and the 337.5 to 360.0 range
END
END
GO
If the points data type are "Geometry" (Like UTM Coordinate System), you may use the following formula:
DEGREES(ATAN((X2-X1)/(Y2-Y1)))
+case when Y2<Y1 then 180 else 0 end
+case when Y2>Y1 and X2<X1 then 360 else 0 end
Here is the schema for more clarification:
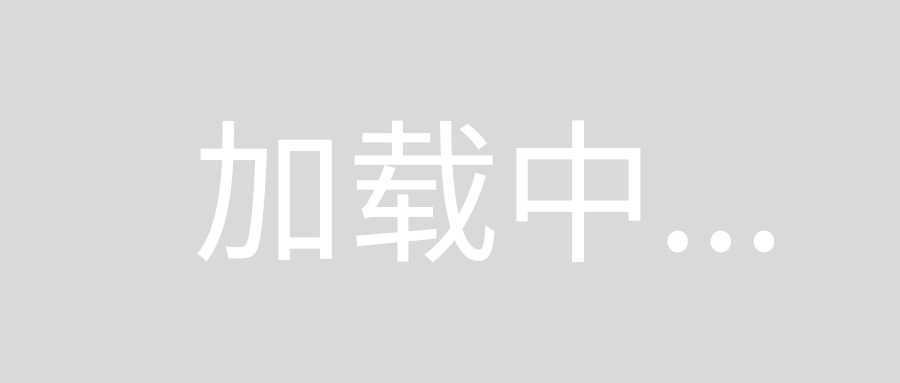
X=X2-X1
and Y=Y2-Y1.
A formula that gives the bearing clockwise from 0 (positive Y axis) to 360 degrees.
f(X,Y)=180-90*(1+SIGN(Y))*(1-SIGN(X^2))-45*(2+SIGN(Y))*SIGN(X)-180/PI()*SIGN(Y*X)*ATAN((ABS(Y)-ABS(X))/(ABS(Y)+ABS(X)))