I'm using IE 11 to emulate older versions of IE. The following code does not work as expected in IE 9 and below:
var search_input_val = $.trim($("#search_input").val()).replace(/\s{2,}/g, ' ');
console.log(search_input_val);
var recBox_val_arr = search_input_val.split(/\s+/); // HERE
console.log(recBox_val_arr);
recBox_val_arr
is logged to the console as undefined
.
The above code returns an Array
on IE 10 and 11, Firefox, Chrome, Opera and Safari. Why is it not working in IE 9 and below?
More details
Given this situation:
$("#search_input").val() === "ab abc";
search_input_val === "ab abc";
recBox_val_arr
is logged as undefined
by IE ≤ 9, [object Array]
by Firefox and ["ab", "abc"]
by other browsers.
I'm linking to jQuery 1.10.2 via Google's CDN:
//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js
I also tried recBox_val_arr = search_input_val.split(' ')
, but recBox_val_arr
is still logged as undefined
.
IE 11's implementation of IE 9's console.log
is busted
There are indeed bugs with older IE's implementation of split
, but that's not the problem here. In fact, split
is working just fine—the real issue is IE 11's busted implementation of console.log
when emulating IE 9:
console.log("test test".split(/\s+/)) // logs "undefined"
"test test".split(/\s+/) // logs "[object Array]"
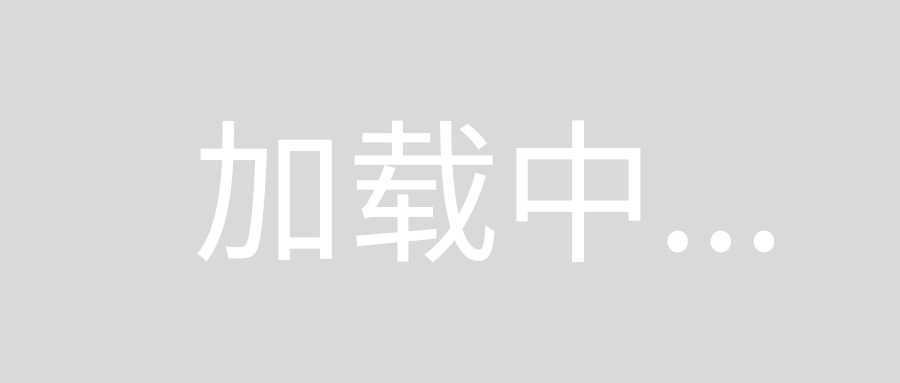
Like really, really busted
More generally, console.log
in IE 11 emulating IE 9 doesn't support logging of objects or arrays:
console.log("foo"); // logs "foo"
console.log({ foo: "bar" }) // logs "undefined"
console.log(["foo"]) // logs "undefined"
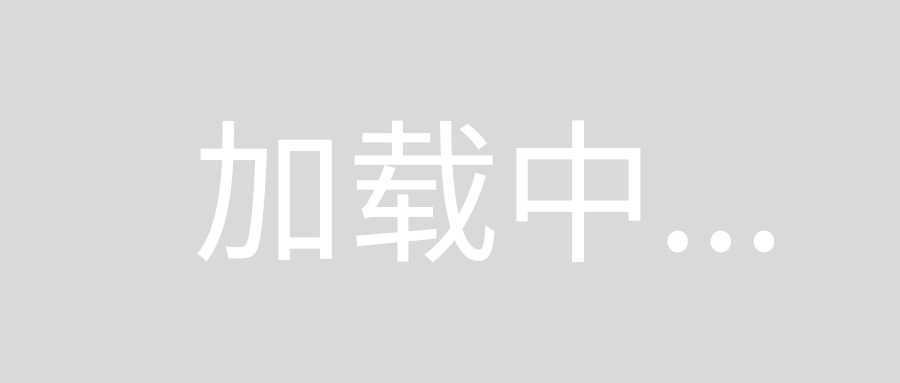
Even IE 9 wasn't this bad
Worst of all, this isn't even comparable with how IE 9 actually behaves. Here's what you get if you run IE 9 directly on a VM:
console.log("test test".split(/\s+/)) // logs "test,test"
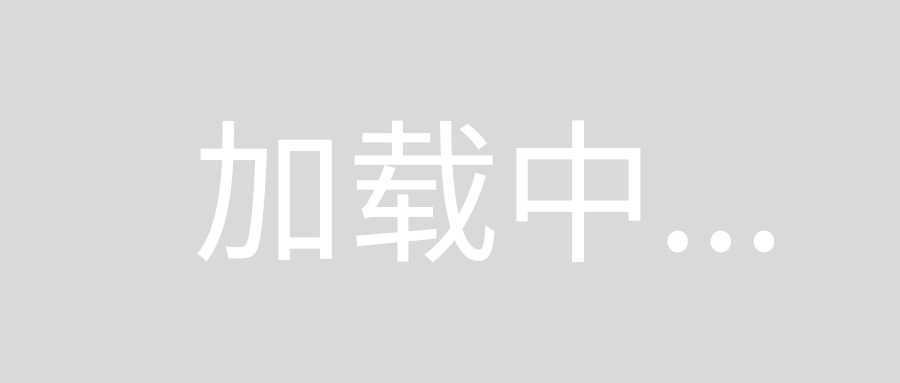
Summary
- IE 11's emulation of IE 9 isn't perfect.
console.log
is totally borked when called on IE 11 emulating IE 9.
- Always use a VM (freely available to download) for reliable cross-browser testing.
$(document).ready(function(){
$("button").click(function(){
var search_input_val = $.trim($("#search_input").val()).replace(/\s{2,}/g, ' ');
console.log(search_input_val);
var recBox_val_arr = search_input_val.split(/\s+/); //--Here--
console.log(recBox_val_arr);
console.log(recBox_val_arr[0]);
console.log(recBox_val_arr[1]);
console.log(recBox_val_arr[2]);
});
});
Create body with
< body>
< input type="text" id="search_input" />
< button>Submit < /button>
< /body>
console.log(recBox_val_arr);
Input => hello 123 hi
Output => ["hello", "123", "hi"]
Split function in PHP is used to break string into an array.
console.log(recBox_val_arr[0]); == hello
console.log(recBox_val_arr[1]); == 123
console.log(recBox_val_arr[2]); == hi
If you put console.log() outside $("button").click(function(){}); then the value will be initially shown as "undefined".
Because it was not able to get value from text box.