I'm working with HTML5 Canvas now. I have one image file and and a mug image file. I want the image file to be drawn on that cylinder surface. Something like the images below.
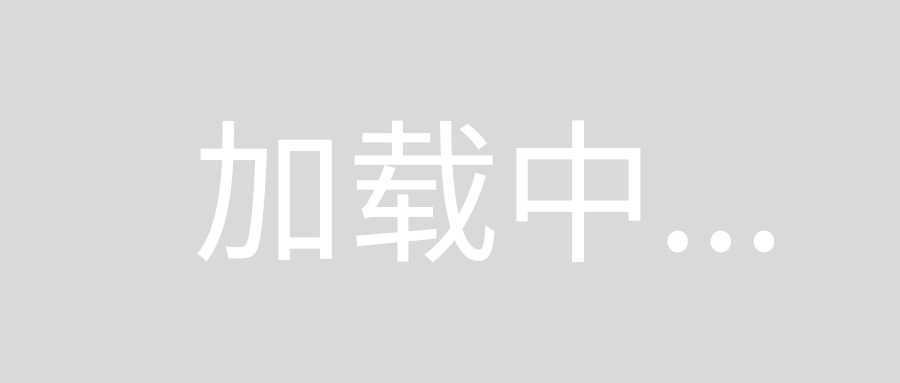
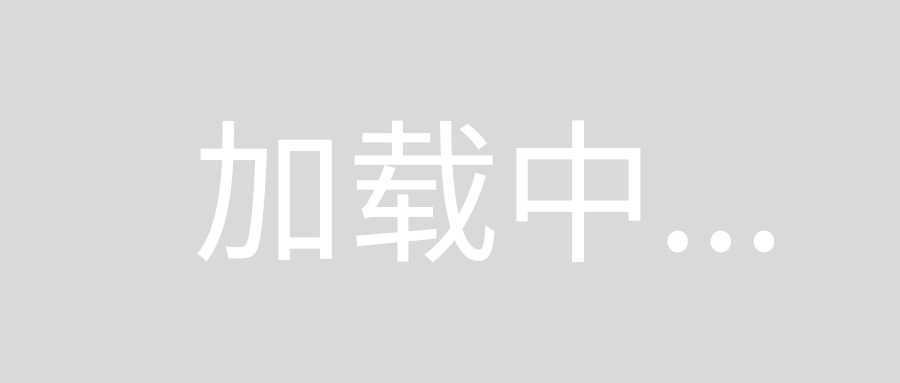
Are there any Javascript canvas (or maybe SVG) libraries that can help me archive that?
Thank you very much
You can achieve your "wrap" effect by slicing your image into 1px wide vertical slices and changing the "Y" coordinate of each slice to fit the curve of your cup.
Here's example code that uses this technique to "stretch" an image.
Feel free to modify this code to fit along the curve of your cup.
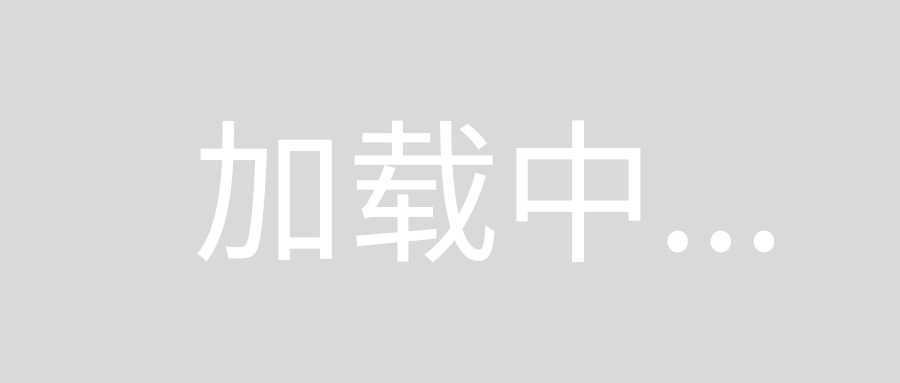
Example code and a Demo: http://jsfiddle.net/m1erickson/krhQW/
<!doctype html>
<html>
<head>
<link rel="stylesheet" type="text/css" media="all" href="css/reset.css" /> <!-- reset css -->
<script type="text/javascript" src="http://code.jquery.com/jquery.min.js"></script>
<style>
body{ background-color: ivory; }
canvas{border:1px solid red;}
</style>
<script>
$(function(){
var canvas=document.getElementById("canvas");
var ctx=canvas.getContext("2d");
var img=new Image();
img.onload=start;
img.src="https://dl.dropboxusercontent.com/u/139992952/warp.png";
function start(){
var iw=img.width;
var ih=img.height;
canvas.width=iw+20;
canvas.height=ih+20;
var x1=0;
var y1=50;
var x2=iw;
var y2=0
var x3=0;
var y3=ih-50;
var x4=iw;
var y4=ih;
// calc line equations slope & b (m,b)
var m1=Math.tan( Math.atan2((y2-y1),(x2-x1)) );
var b1=y2-m1*x2;
var m2=Math.tan( Math.atan2((y4-y3),(x4-x3)) );
var b2=y4-m2*x4;
// draw vertical slices
for(var X=0;X<iw;X++){
var yTop=m1*X+b1;
var yBottom=m2*X+b2;
ctx.drawImage( img,X,0,1,ih, X,yTop,1,yBottom-yTop );
}
// outline
ctx.beginPath();
ctx.moveTo(x1,y1);
ctx.lineTo(x2,y2);
ctx.lineTo(x4,y4);
ctx.lineTo(x3,y3);
ctx.closePath();
ctx.stroke();
}
}); // end $(function(){});
</script>
</head>
<body>
<canvas id="canvas" width=300 height=300></canvas>
</body>
</html>