well I know that every imageViews have a layer (we can access it with :imageView.layer).I would like to use the layer of my imageView
and add a subLayer to it's layer:shapeLayer
(that is a CAShapeLayer) My code doesn't work, it doesn't show the shape layer!
- (void)viewDidLoad
{
imageView = [[UIImageView alloc] initWithFrame:CGRectMake(100, 100, 100, 100)];
[self.view addSubview:imageView];
}
- (void)anotherMethod
{
[imageView.layer addSublayer:shapeLayer];
}
How can I solve this please ?
Try this:
[outputImage.layer insertSublayer:shapeLayer atIndex:0];
I solved similar problem by using UIView
below UIImageView
and setting a layer to UIView
. When you add a layer to UIImageView
at index 0, your image will not be visible.
I have a UIView
called photoView
. It has a subview of class UIImageView
, that has same bounds and has a clear background color. And I add a gradientLayer
to photoView
.
- (void)viewDidLoad
{
[super viewDidLoad];
// Adding gradient background to UIImageView
CAGradientLayer *gradientLayer = [CAGradientLayer layer];
gradientLayer.frame = self.photoImageView.bounds;
gradientLayer.colors = @[(id)[UIColor customUltraLightGrayColor].CGColor, (id)[UIColor customUltraLightGrayColor].CGColor, (id)[UIColor customDarkGrayColor].CGColor];
gradientLayer.locations = @[@(0.0), @(0.6)];
[self.photoView.layer insertSublayer:gradientLayer atIndex:0];
}
As a result I get this:
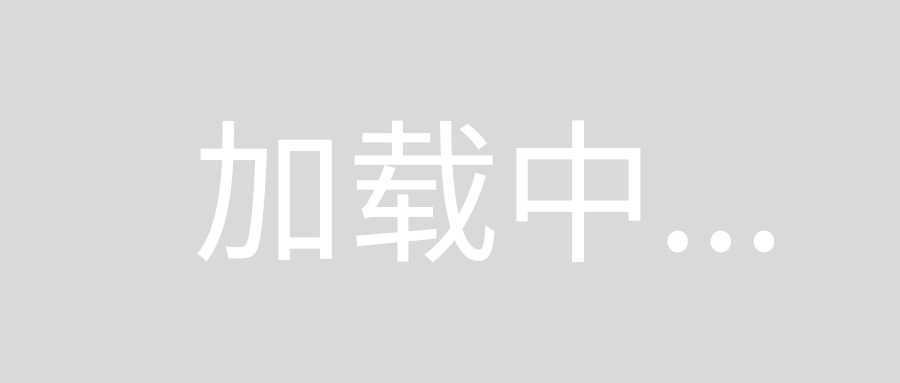
This could have several reasons, for example:
Did you #import <QuartzCore/QuartzCore.h>
(and / or CoreGraphics/CoreGraphics.h>
depending on what you are doing in the rest of your code)?
You could have set a wrong CGRectMake
when initializing the CALayer
which is not inside your visible frame (Like CGRectMake(5000,5000,...,...)
) or one of the size
properties is 0
?
Try setting the frame for layer:
Follow the three steps
CALayer layerToBeAdded = [[CALayer alloc] init];
[layerToBeAdded setFrame:imgView.frame];
[imgView.layer addSublayer:layerToBeAdded];
Instead of allocating CALayer, you can use [imgView layer]
And to add image to layer do it like this
layerToBeAdded.contents = (id)imgXYZ.CGImage;
Assuming that you are talking about just one UIImageView (imageView == outputImage ???)
//in another method:
{
[imageView.layer addSublayer:shapeLayer]; //Where is outputImage initialized?
}
Here is a solution:
CALayer *pulseLayer_ = [[CALayer layer] retain];
pulseLayer_.backgroundColor = [[UIColor whiteColor] CGColor];
pulseLayer_.bounds = CGRectMake(0., 0., 80., 80.);
pulseLayer_.cornerRadius = 12.;
pulseLayer_.position = self.view.center;
[self.view.layer addSublayer:pulseLayer_];
CALayer *imageLayer = [CALayer layer];
imageLayer.frame = pulseLayer_.bounds;
imageLayer.cornerRadius = 10.0;
imageLayer.contents = (id) [UIImage imageNamed:@"jacklogo.png"].CGImage;
imageLayer.masksToBounds = YES;
[pulseLayer_ addSublayer:imageLayer];
[pulseLayer_ setNeedsDisplay];