I'm fairly new to iOS development but have reached the point where I want to create my own composite UIView as a custom UIButton. I would like to layout a UILabel and 2x UIImageViews as follows;
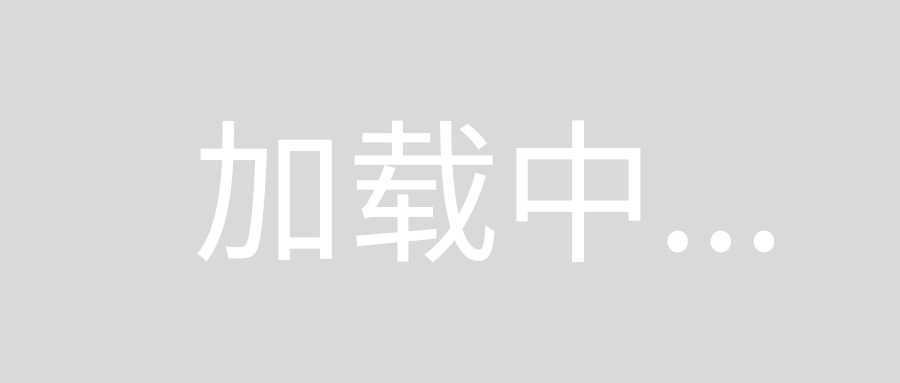
But I would also like to anchor the controls (subviews) in such a way that as the label expands, due to translations say, the view automatically handles the extra real estate. For example;
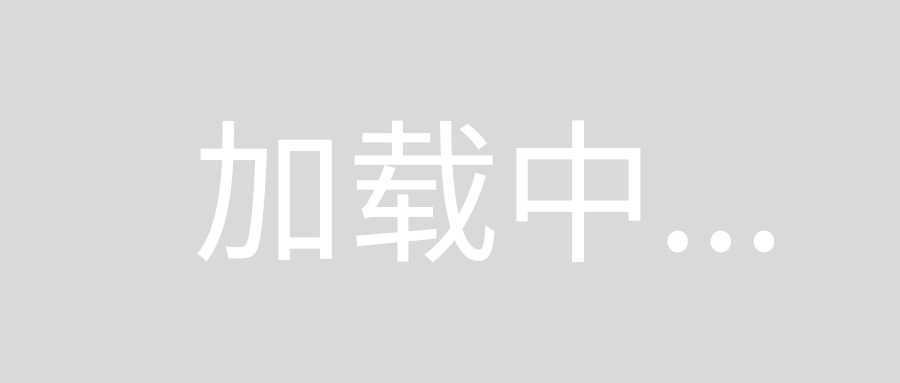
Ideally -
- the right hand side UIView is anchored to the right; and remains a fixed width/hight (right aligned).
- the label and bottom image divide the remaining left hand space
- the label is vertically centered in the top half of the upper remaining space
- the bottom image remains centered (both vertically and horizontally) in the lower remaining space
- if the label is wider than the bottom image then the view should expand
I'm happy to construct this in pure code if required. I used a XIB in the above images to play with attributes and to visualize my question.
I'm from a C#/XAML background so I would typically use grid layouts with fixed/auto/* columns and rows but I'm guessing I need to use something like NSLayoutConstraints here - unfortunately I don't know where to start or how to search for the solution. Any help appreciated!
in your UIButton
(code not tested)
//put the code below in your button's init method
UILabel *label = [[UILabel alloc] init];
UIImageView *bottomImageView = [[UIImageView alloc] init];
UIImageView *rightImageView = [[UIImageView alloc] init];
// we define our own contraints,
// we don't want the system to fall-back on UIView's autoresizingMask property (pre-iOS 6)
self.translatesAutoresizingMaskIntoConstraints = NO;
label.translatesAutoresizingMaskIntoConstraints = NO;
bottomImageView.translatesAutoresizingMaskIntoConstraints = NO;
rightImageView.translatesAutoresizingMaskIntoConstraints = NO;
//fixed sizes => SET THESE as you want
CGFloat labelHeight, bottomWidth, rightImageWidth;
// 1 - Label constraints :
// labelWidth = 1 *self.width - rightImageWidth
NSLayoutConstraint *labelWidth = [NSLayoutConstraint constraintWithItem:label
attribute:NSLayoutAttributeWidth
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeWidth
multiplier:1
constant:(- rightImageWidth)];
// label must be at the top :
NSLayoutConstraint *labelTop = [NSLayoutConstraint constraintWithItem:label
attribute:NSLayoutAttributeTop
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeTop
multiplier:1
constant:0];
//label must be on the left border
NSLayoutConstraint *labelLeft = [NSLayoutConstraint constraintWithItem:label
attribute:NSLayoutAttributeLeft
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeLeft
multiplier:1
constant:0];
//label must be of 1/2 height
NSLayoutConstraint *labelHeight = [NSLayoutConstraint constraintWithItem:label
attribute:NSLayoutAttributeHeight
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeHeight
multiplier:0.5
constant:0];
[self addSubview:label];
[self addConstraints:@[labelWidth, labelTop, labelLeft, labelHeight]];
//2 - botom view
// width constant
NSLayoutContraint *bottomWidth = [NSLayoutConstraint constraintWithItem:bottomImageView
attribute:NSLayoutAttributeWidth
relatedBy:NSLayoutRelationEqual
toItem:nil
attribute:NSLayoutAttributeNotAnAttribute
multiplier:0
constant:bottomWidth];
// same height constraint as label
NSLayoutConstraint *bottomHeight = [NSLayoutConstraint constraintWithItem:bottomImageView
attribute:NSLayoutAttributeHeight
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeHeight
multiplier:0.5
constant:0];
// sticks at container's bottom (pun intended)
NSLayoutConstraint *bottomBottom = [NSLayoutConstraint constraintWithItem:bottomImageView
attribute:NSLayoutAttributeBottom
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeBottom
multiplier:1
constant:0];
//we have height, width, y contraints, just x remains
// NOTE : this one is between bottom view and label
NSLayoutConstraint *bottomCenteredXAsLabel = [NSLayoutConstraint constraintWithItem:bottomImageView
attribute:NSLayoutAttributeCenterX
relatedBy:NSLayoutRelationEqual
toItem:label
attribute:NSLayoutAttributeCenterX
multiplier:1
constant:0];
[self addSubview:bottomImageView];
[self addConstraints:@[bottomWidth, bottomHeight, bottomBottom, bottomCenteredXAsLabel]];
// 3 - last one !
NSLayoutConstraint *rightAligned = [NSLayoutConstraint constraintWithItem:rightImageView
attribute:NSLayoutAttributeRight
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeRight
multiplier:1
constant:0];
//right height
NSLayoutConstraint *rightHeight = [NSLayoutConstraint constraintWithItem:rightImageView
attribute:NSLayoutAttributeHeight
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeHeight
multiplier:1
constant:0];
//constant width...
NSLayoutConstraint *rightWidth = [NSLayoutConstraint constraintWithItem:rightImageView
attribute:NSLayoutAttributeWidth
relatedBy:NSLayoutRelationEqual
toItem:nil
attribute:NSLayoutAttributeNotAnAttribute
multiplier:0
constant:rightImageWidth];
//width, height, x constraints...
//we still need one on y, let's say it sticks at the top
NSLayoutConstraint *rightTop = [NSLayoutConstraint constraintWithItem:rightImageView
attribute:NSLayoutAttributeTop
relatedBy:NSLayoutRelationEqual
toItem:self
attribute:NSLayoutAttributeTop
multiplier:1
constant:0];
[self addSubview:rightImageView];
[self addConstraints:@[rightAligned, rightHeight, rightWidth, rightTop]];
Et voilà !
The method is always the same : you need at least 4 constraints on each view, setting width, height, x, and y (CGRect 4 dimensions).
Think of a constraint as a relation :
item1.layoutAttribute1 >= a*item2.layoutAttribute2 + b
to translate in this form
[NSLayoutConstraint constraintWithItem:item1
attribute:layoutAttribute1
relatedBy:NSLayoutRelationGreaterThanOrEqual
toItem:item2
attribute:layoutAttribute2
multiplier:a
constant:b];
Note that using visual format, you might express all that with less code. (but I've not played with it yet).