I am supplying the following regex : ^((?:\+27|27)|0)(\d{9})$
for number of South Africa and want to only return true for numbers that start with +27 or 27 or 0. eg; +27832227765, 27838776654 or 0612323434.
I tried using:
regexplanet.com
and regex test
But both return false no matter what I enter (even simple regex).
Anybody know what I am doing wrong?
In Java code, you need to double the backslashes, and in a online tester, the backslashes must be single.
Also, it is not necessary to use start and end of string markers if used with String#matches
as this method requires a full string match.
Here is how your Java code can look like:
String rex = "(\\+?27|0)(\\d{9})";
System.out.println("+27832227765".matches(rex)); // => true
System.out.println("27838776654".matches(rex)); // => true
System.out.println("0612323434".matches(rex)); // => true
See IDEONE demo
If you use String#find
, you will need the ^
and $
anchors you have in the original regex.
Note I shortened ((?:\+27|27)|0)
to (\+?27|0)
as ?
quantifier means 1 or 0 occurrences. The extra grouping is really redundant here. Also, If you are not using captured texts, I'd suggest turning the first group into a non-capturing (i.e. (?:\+?27|0)
) and remove the round brackets from \d{9}
:
String rex = "(?:\\+?27|0)\\d{9}";
This is an expression tested on http://tools.netshiftmedia.com/regexlibrary/# for numbers that start with +27 or 27 or 0 and after with length 9.
^((0)|(27)|(\+27))(\d{9})$
It's ok with your numbers :
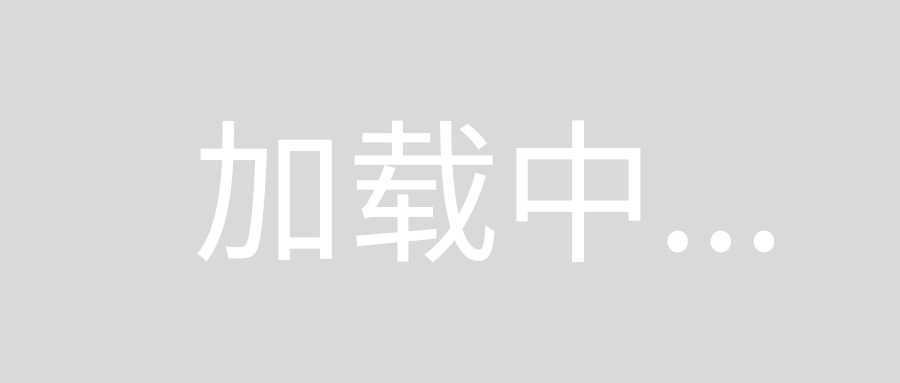
This should work, here is a variant. (I removed ^ and $ for this example):
((?:\+27|27|0)(?:\d{9}))