I am using an app which displays a drop down Spinner
with an EditText
as a child of TextInputLayout
next to it.
When an underlined error is shown under the TextInputLayout
, then the EditText
will go up, and alignment will miss !!
See my screenshot please:
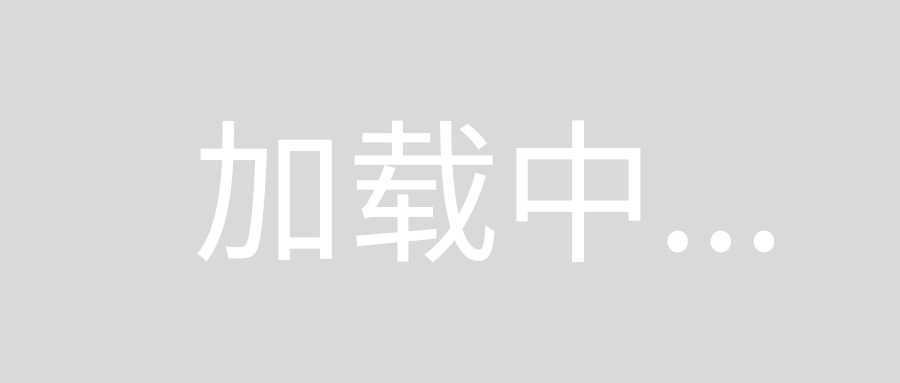
What I am trying to do is:
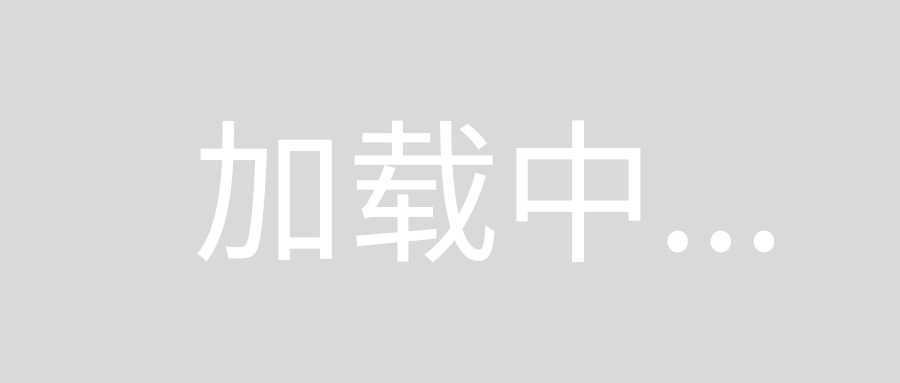
Here is my xml code:
<LinearLayout
android:layout_width="@dimen/edittext_width"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="bottom">
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinner_countries"
style="@style/Widget.AppCompat.Spinner.Underlined"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="30"
android:layout_gravity="start"
android:spinnerMode="dialog" />
<android.support.design.widget.TextInputLayout
android:id="@+id/input_layout_mobile"
style="@style/TextInputLayoutTheme"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="70">
<com.cashu.app.ui.widgets.CustomEditText
android:id="@+id/et_fragment_register_step_one_mobile"
android:layout_width="@dimen/edittext_mobile_num_width"
android:layout_height="wrap_content"
android:ems="12"
android:gravity="center_vertical"
android:layout_gravity="start"
android:hint="@string/mobile_hint"
android:inputType="number"
android:textSize="@dimen/font_size_normal" />
</android.support.design.widget.TextInputLayout>
</LinearLayout>
Here is my TextInputLayout
theme:
<!-- TextInputLayout Style -->
<style name="TextInputLayoutTheme" parent="Widget.Design.TextInputLayout">
<item name="android:gravity">center</item>
<item name="android:textColor">@color/white</item>
<item name="android:textColorHint">@color/colorControlActivated</item>
<item name="errorTextAppearance">@style/AppTheme.TextErrorAppearance</item>
<item name="counterTextAppearance">@style/TextAppearance.Design.Counter</item>
<item name="counterOverflowTextAppearance">@style/TextAppearance.Design.Counter.Overflow</item>
</style>
I tried searching for many keywords, terms and methods but unfortunately with no luck.
I would appreciate if anyone has a hack for this issue.
Add app:errorEnabled="true"
in TextInputLayout.
This will leave space for error even if error is not displayed. This will consume more space but will help you to maintain alignment.
Make your TextInputLayout as below.
<android.support.design.widget.TextInputLayout
android:id="@+id/input_layout_mobile"
style="@style/TextInputLayoutTheme"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="70"
app:errorEnabled="true">
Later in java:
To set error text use
textInputLayout.setError("Email can not be blank");
To remove error text and set view as normal
textInputLayout.setError("");
*Note that the use of textInputLayout.setErrorEnable(false)
to remove the error will take out entire text view of error from the textInputLayout which will disturb your alignment.
I figured out my problem, I set margin to my AppCompatSpinner
and set top gravity to it with specific hight.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="bottom"
android:orientation="horizontal">
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinner_countries"
style="@style/Widget.AppCompat.Spinner.Underlined"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginBottom="26dp"
android:layout_weight="30"
android:spinnerMode="dialog" />
<android.support.design.widget.TextInputLayout
android:id="@+id/input_layout_mobile"
style="@style/TextInputLayoutTheme"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="70"
app:errorEnabled="true">
<com.cashu.app.ui.widgets.CustomEditText
android:id="@+id/et_mobile"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="start"
android:ems="12"
android:gravity="center_vertical"
android:hint="@string/mobile_hint"
android:inputType="number"
android:textSize="@dimen/font_size_normal" />
</android.support.design.widget.TextInputLayout>
</LinearLayout>
[EDIT]
When my TextInputLayout
has an error, I remove it by adding the following lines:
myTextInputLayout.setErrorEnabled(false);
myTextInputLayout.setErrorEnabled(true);
myTextInputLayout.setError(" ");
Why?
This line: myTextInputLayout.setErrorEnabled(false);
will clear the error from the TextInputLayout
This line: myTextInputLayout.setErrorEnabled(true);
will enable error for the TextInputLayout
again
This line: myTextInputLayout.setError(" ");
will prevent layout alignment disruption.
Problem now is solved.
try this,
<LinearLayout
android:layout_width="300dp"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="bottom">
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinner_countries"
style="@style/Widget.AppCompat.Spinner.Underlined"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="30"
android:spinnerMode="dialog" />
<android.support.design.widget.TextInputLayout
android:id="@+id/input_layout_mobile"
style="@style/TextInputLayoutTheme"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="70">
<EditText
android:id="@+id/et_fragment_register_step_one_mobile"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:ems="12"
android:gravity="center_vertical"
android:layout_gravity="start"
android:hint="Mobile No."
android:inputType="number"
android:textSize="20sp" />
</android.support.design.widget.TextInputLayout>