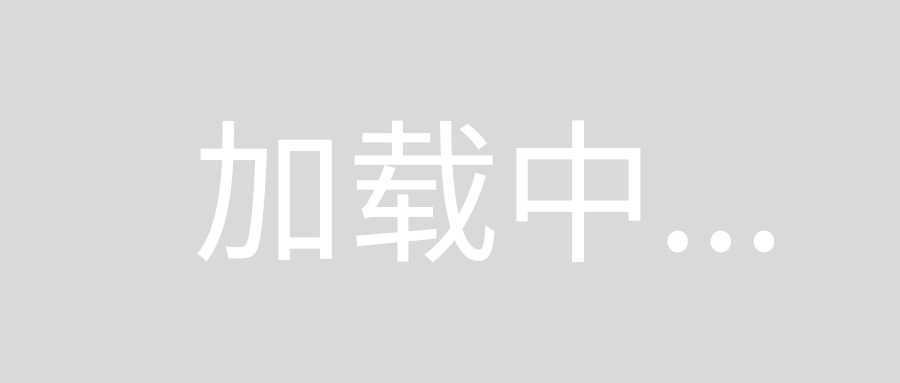
I have an app that basically looks like the design in the attached picture.
As you can see, there are 4 activities, and some of the activities have fragments.
I want to get the answer of a test back to the user's profile.
Up to now, I've been uploading the result to the server and having the app update the user's profile every time they go back to the ProfileActivity, but that seems like a waste of resources.
Is there a pattern or way to do this in the app?
I looked at this: which seems doable if I can somehow link two startActivityForResult()
s.
I looked into using the delegate pattern described in this question but I can't get the instance of the UserFragment to the TestActivity.
I hope someone can point me in the direction of the correct way of doing this.
One approach is use startActivityForResult()
don't finish any Activity
start all activities by startActivityForResult()
then depending on your condition you can finish activity
pass result back to the previous activity using onActivityResult()
Then for fragment you can store fragment object in ProfileActivity .
In Fragment write method for updating UI
So you can access that method using fragment object
class ProfileActivity extends ......
{
@override
public void onActivityResult(....)
{
.....
frgamnetObject.updateUI();
....
}
}
Assuming that you are getting one int value from last page:
create class :
public class GenericUtility {
public static int getIntFromSharedPrefsForKey(String key, Context context)
{
int selectedValue = 0;
SharedPreferences prefs = context.getSharedPreferences("com.your.packagename", Context.MODE_PRIVATE);
selectedValue = prefs.getInt(key, 0);
return selectedValue;
}
public static boolean setIntToSharedPrefsForKey(String key, int value, Context context)
{
boolean savedSuccessfully = false;
SharedPreferences prefs = context.getSharedPreferences("com.your.packagename", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = prefs.edit();
try
{
editor.putInt(key, value);
editor.apply();
savedSuccessfully = true;
}
catch (Exception e)
{
savedSuccessfully = false;
}
return savedSuccessfully;
}
public static String getStringFromSharedPrefsForKey(String key, Context context)
{
String selectedValue = "";
SharedPreferences prefs = context.getSharedPreferences("com.your.packagename", Context.MODE_PRIVATE);
selectedValue = prefs.getString(key, "");
return selectedValue;
}
public static boolean setStringToSharedPrefsForKey(String key, String value, Context context)
{
boolean savedSuccessfully = false;
SharedPreferences prefs = context.getSharedPreferences("com.your.packagename", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = prefs.edit();
try
{
editor.putString(key, value);
editor.apply();
savedSuccessfully = true;
}
catch (Exception e)
{
savedSuccessfully = false;
}
return savedSuccessfully;
}
}
than to set int value to it:
GenericUtility.setIntToSharedPrefsForKey("selected_theme", 1, getApplicationContext());
or
GenericUtility.setIntToSharedPrefsForKey("selected_theme", 1, MyActivity.this))
and in your very first activity where you want result:
int selectedValue = GenericUtility.getIntFromSharedPrefsForKey("selected_theme", getApplicationContext());
or
int selectedValue = GenericUtility.getIntFromSharedPrefsForKey("selected_theme", MyActivity.this);
selectedValue will return default value if there is no value in it.
PS change int to String in class to get and set result accordingly.