I got this alertDialog with a multiple choice item selection:
builder.setTitle(R.string.layer_options_title)
.setMultiChoiceItems(availableOptions, selectedLayerOptions, new DialogInterface.OnMultiChoiceClickListener() {
...
});
where
availableOptions = getApplicationContext().getResources().getStringArray(R.array.layer_options);
comes from
<string-array name="layer_options">
<item>Should have green background</item>
<item>Should have yellow background</item>
<item>Should have orange background</item>
<item>Should have blue background</item>
</string-array>
Is there any way to make these multiple choice items have a background color?
Yes, there is a way. Put your checkbox in a layout and give the layout backgrond color. This is how I could do it:
AlertDialog.Builder alert = new AlertDialog.Builder(this);
LinearLayout mainLayout = new LinearLayout(this);
mainLayout.setOrientation(LinearLayout.VERTICAL);
LinearLayout layout1 = new LinearLayout(this);
layout1.setOrientation(LinearLayout.HORIZONTAL);
CheckBox cb1 = new CheckBox(getApplicationContext());
cb1.setText("Easy");
layout1.addView(cb1);
layout1.setBackgroundColor(Color.BLUE);
layout1.setMinimumHeight(50);
LinearLayout layout2 = new LinearLayout(this);
layout2.setOrientation(LinearLayout.HORIZONTAL);
layout2.addView(new TextView(this));
CheckBox cb2 = new CheckBox(getApplicationContext());
cb2.setText("Normal");
layout2.addView(cb2);
layout2.setBackgroundColor(Color.CYAN);
layout2.setMinimumHeight(50);
LinearLayout layout3 = new LinearLayout(this);
layout3.setOrientation(LinearLayout.HORIZONTAL);
CheckBox cb3 = new CheckBox(getApplicationContext());
cb3.setText("Hard");
layout3.addView(cb3);
layout3.setBackgroundColor(Color.GREEN);
layout3.setMinimumHeight(50);
mainLayout.addView(layout1);
mainLayout.addView(layout2);
mainLayout.addView(layout3);
alert.setTitle("Custom alert demo");
alert.setView(mainLayout);
alert.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.cancel();
}
});
alert.setPositiveButton("Done", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(getBaseContext(), "done", Toast.LENGTH_SHORT).show();
}
});
alert.show();
The result:
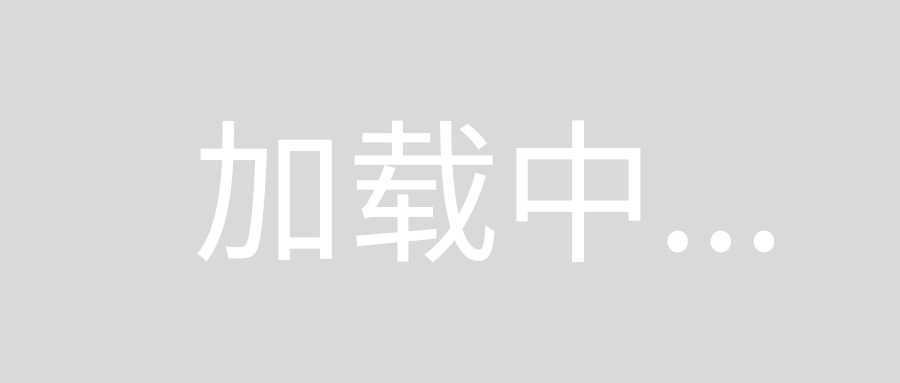
Firstly, I created a main layout (vertical) as you see in the code. Then, for each one of the checkboxes I created a horizontal layout. In this case you can play with the colors and fonts of the elements (checkboxes, items, and etc.).
Try this:
AlertDialog.Builder builder1 = new AlertDialog.Builder(MainActivity.this);
builder1.setTitle("Some Titel?");
builder1.setCancelable(true);
List<Spanned> listItems = new ArrayList<Spanned>();
listItems.add(Html.fromHtml("ipohne"));
listItems.add(Html.fromHtml("windows"));
listItems.add(Html.fromHtml("<span style='background-color: #FFFF00'>samsung</span>"));
final CharSequence[] charSequenceItems = listItems.toArray(new CharSequence[listItems.size()]);
builder1.setMultiChoiceItems(charSequenceItems, null, null);
builder1.setPositiveButton("Weiter",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
dialog.cancel();
}
});
builder1.setNegativeButton("Abbrechen",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
dialog.cancel();
}
});
AlertDialog alert11 = builder1.create();
alert11.show();
screenshot