I'm looking to generate a binomial-esque distribution. I want a binomial distribution but I want it centred around zero (I know this doesn't make much sense with respect to the definition of binomial distributions but still, this is my goal.)
The only way I have found of doing this in python is:
def zeroed_binomial(n,p,size=None):
return numpy.random.binomial(n,p,size) - n*p
Is there a real name for this distribution? Does this code actually give me what I want (and how can I tell)? Is there a cleaner / nicer / canonical / already implemented way of doing this?
What you're doing is fine if you want a "discretized" normal distribution centered around 0. If you want integer values, you should round n*p
before subtracting.
But the limit of the binomial distribution is just the normal distribution when n
becomes large and with p
bounded away from 0 or 1. since n*p
is not going to be an integer except for certain values, why not just use the normal distribution?
The probability distributions implemented in the scipy.stats
module allow you to shift distributions arbitrarily by specifying the loc
keyword in the constructor. To get a binomial distribution with mean shifted close to 0, you can call
p = stats.binom(N, p, loc=-round(N*p))
(Be sure to use an integer value for loc
with a discrete distribution.)
Here's an example:
p = stats.binom(20, 0.1, loc=-2)
x = numpy.arange(-3,5)
bar(x, p.pmf(x))
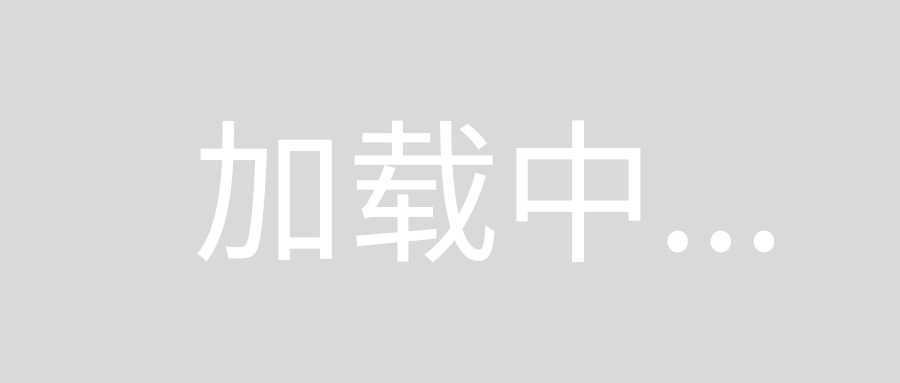
Edit:
To generate the actual random numbers, use the rvs()
method which comes with every random distribution in the scipy.stats
module. For example:
>>> stats.binom(20,0.1,loc=-2).rvs(10)
array([-2, 0, 0, 1, 1, 1, -1, 1, 2, 0])