I'm trying to stitch some images together using java. I have a bunch of images I'd like to stitch together and they are all the same dimensions so it's really just a question of lining them up next to each other I suppose. I have it working but it's very slow and probably very memory intensive. I'm wondering if there's an easier way:
public static void main(String[] args) throws IOException
{
int dim = 256;
BufferedImage merged = null;
for(int y = 0; y<10;y++)
{
for(int x = 0; x<10;x++)
{
URL url = new URL(someURL);
BufferedImage nextImage = ImageIO.read(url);
if(merged==null)
merged=nextImage;
else
{
BufferedImage tempMerged;
tempMerged = new BufferedImage(10*dim,10*dim,merged.getType());
//Write first image
for(int xx=0;xx<merged.getWidth();xx++)
for(int yy=0;yy<merged.getHeight();yy++)
tempMerged.setRGB(xx,yy,merged.getRGB(xx,yy));
//Write img2
for(int xx=0;xx<dim;xx++)
{
for(int yy=0;yy<dim;yy++)
{
int destX = (x*dim)+xx;
int destY = (y*dim)+yy;
tempMerged.setRGB(destX,destY,nextImage.getRGB(xx,yy));
}
}
merged=tempMerged;
}
System.out.println("Stitched image at "+x+","+y);
}
}
ImageIO.write(merged, "png", new File("merged.png"));
}
AFAIK what you're doing here is to write layers to a image. However, the png format doesn't support this.
You'd have to create a new image of twice the size of the source images (e.g. for 2x 512x512 the new image should be 512x1024 or 1024x512). Then you'd render the source images to the respective area/rectangle of the target image.
@Thomas: You'd have to create a new image of twice the size of the source images (e.g. for 2x 512x512 the new image should be 512x1024 or 1024x512). Then you'd render the source images to the respective area/rectangle of the target image
E.G. TiledImageWrite.java
import java.awt.image.BufferedImage;
import java.awt.*;
import javax.swing.*;
import java.net.URL;
import java.io.File;
import javax.imageio.ImageIO;
class TiledImageWrite {
public static void main(String[] args) throws Exception {
URL dayStromloUrl = new URL("http://pscode.org/media/stromlo1.jpg");
URL nightStromloUrl = new URL("http://pscode.org/media/stromlo2.jpg");
final BufferedImage dayStromloImage = ImageIO.read(dayStromloUrl);
final BufferedImage nightStromloImage = ImageIO.read(nightStromloUrl);
final int width = dayStromloImage.getWidth();
final int height = dayStromloImage.getHeight();;
final BufferedImage columnImage =
new BufferedImage(width,2*height,BufferedImage.TYPE_INT_RGB);
final BufferedImage rowImage =
new BufferedImage(2*width,height,BufferedImage.TYPE_INT_RGB);
SwingUtilities.invokeLater( new Runnable() {
public void run() {
JPanel gui = new JPanel(new BorderLayout(3,3));
Graphics2D g2dColumn = columnImage.createGraphics();
g2dColumn.drawImage(dayStromloImage,0,0, null);
// start this one at 'height' down the final image
g2dColumn.drawImage(nightStromloImage,0,height, null);
Graphics2D g2dRow = rowImage.createGraphics();
g2dRow.drawImage(dayStromloImage,0,0, null);
// start this one at 'width' across the final image
g2dRow.drawImage(nightStromloImage,width,0, null);
gui.add(new JLabel(new ImageIcon(columnImage)),BorderLayout.CENTER);
gui.add(new JLabel(new ImageIcon(rowImage)),BorderLayout.SOUTH);
JOptionPane.showMessageDialog(null, gui);
}
} );
ImageIO.write(columnImage, "png", new File("column.png"));
ImageIO.write(rowImage, "png", new File("row.png"));
}
}
column.png
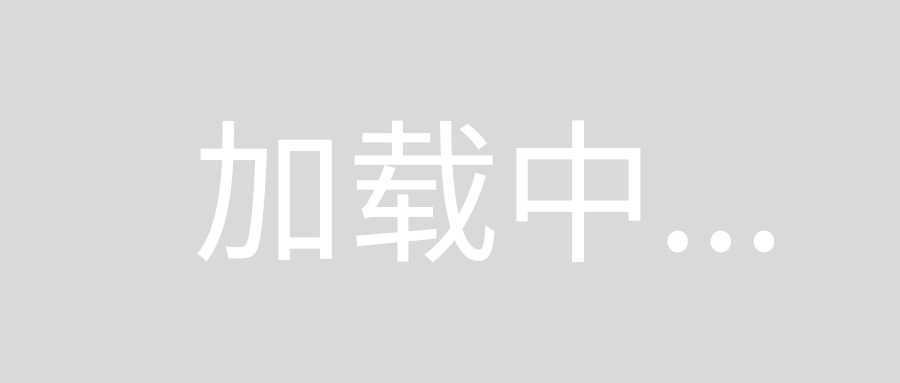
I figured out why it was going slow. In reality, I didn't want to merge images together, but rather stitch together a bunch of images. What I was doing was rewriting the original image everything when all I really want to do is add to it. Much faster now!