I would like to do a rounded slider like the image below.
Is jQuery able to do this?
I know how a straight slider works but I would like to make a HTML5 rounded slider.
Here is what I found online
http://jsfiddle.net/XdvNg/1/ - but I dont know how to get the slider value one the user lets go
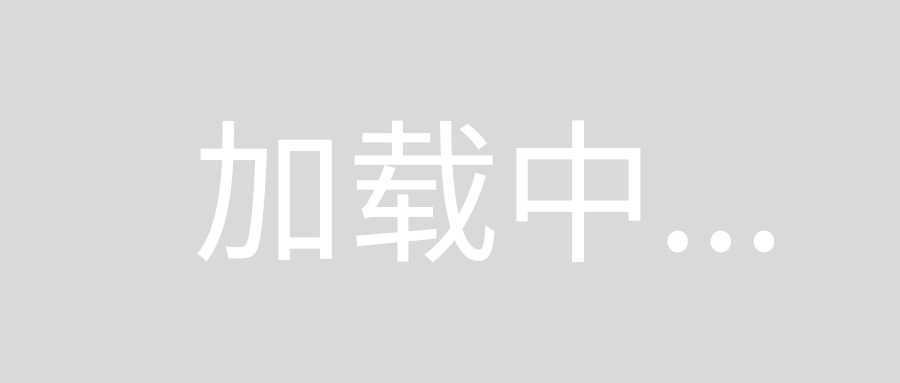
Here is what I came up with:
jsBin demo
$(function () {
var $circle = $('#circle'),
$handler = $('#handler'),
$p = $('#test'),
handlerW2 = $handler.width()/2,
rad = $circle.width()/2,
offs = $circle.offset(),
elPos = {x:offs.left, y:offs.top},
mHold = 0,
PI2 = Math.PI/180;
$handler.mousedown(function() { mHold = 1; });
$(document).mousemove(function(e) {
if (mHold) {
var mPos = {x:e.pageX-elPos.x, y:e.pageY-elPos.y},
atan = Math.atan2(mPos.x-rad, mPos.y-rad),
deg = -atan/PI2+180,
perc = (deg*100/360)|0,
X = Math.round(rad* Math.sin(deg*PI2)),
Y = Math.round(rad* -Math.cos(deg*PI2));
$handler.css({left:X+rad-handlerW2, top:Y+rad-handlerW2, transform:'rotate('+deg+'deg)'});
}
}).mouseup(function() { mHold = 0; });
});
Here is little improved version of Roko's script:
jsFiddle demo
To calculate mouse position I use event offset(little fix for firefox), corrected if event target isn't #rotationSliderContainer. I add also attraction to multiples of 90 degrees.
$(function(){
var $container = $('#rotationSliderContainer');
var $slider = $('#rotationSlider');
var $degrees = $('#rotationSliderDegrees');
var sliderWidth = $slider.width();
var sliderHeight = $slider.height();
var radius = $container.width()/2;
var deg = 0;
X = Math.round(radius* Math.sin(deg*Math.PI/180));
Y = Math.round(radius* -Math.cos(deg*Math.PI/180));
$slider.css({ left: X+radius-sliderWidth/2, top: Y+radius-sliderHeight/2 });
var mdown = false;
$container
.mousedown(function (e) { mdown = true; e.originalEvent.preventDefault(); })
.mouseup(function (e) { mdown = false; })
.mousemove(function (e) {
if(mdown)
{
// firefox compatibility
if(typeof e.offsetX === "undefined" || typeof e.offsetY === "undefined") {
var targetOffset = $(e.target).offset();
e.offsetX = e.pageX - targetOffset.left;
e.offsetY = e.pageY - targetOffset.top;
}
if($(e.target).is('#rotationSliderContainer'))
var mPos = {x: e.offsetX, y: e.offsetY};
else
var mPos = {x: e.target.offsetLeft + e.offsetX, y: e.target.offsetTop + e.offsetY};
var atan = Math.atan2(mPos.x-radius, mPos.y-radius);
deg = -atan/(Math.PI/180) + 180; // final (0-360 positive) degrees from mouse position
// for attraction to multiples of 90 degrees
var distance = Math.abs( deg - ( Math.round(deg / 90) * 90 ) );
if( distance <= 5 )
deg = Math.round(deg / 90) * 90;
if(deg == 360)
deg = 0;
X = Math.round(radius* Math.sin(deg*Math.PI/180));
Y = Math.round(radius* -Math.cos(deg*Math.PI/180));
$slider.css({ left: X+radius-sliderWidth/2, top: Y+radius-sliderHeight/2 });
var roundDeg = Math.round(deg);
$degrees.html(roundDeg + '°');
$('#imageRotateDegrees').val(roundDeg);
}
});
});
Here is the jQuery roundSlider plugin for your requirement, it works fine in mobile devices and touch devices also.
This roundslider having the similar options like the jQuery ui slider. It support default, min-range and range slider type. Not only the round slider it also supports various circle shapes such as quarter, half and pie circle shapes.
For more details check the demos and documentation page.
Please check the demo from jsFiddle
.
Screenshot of the Output:
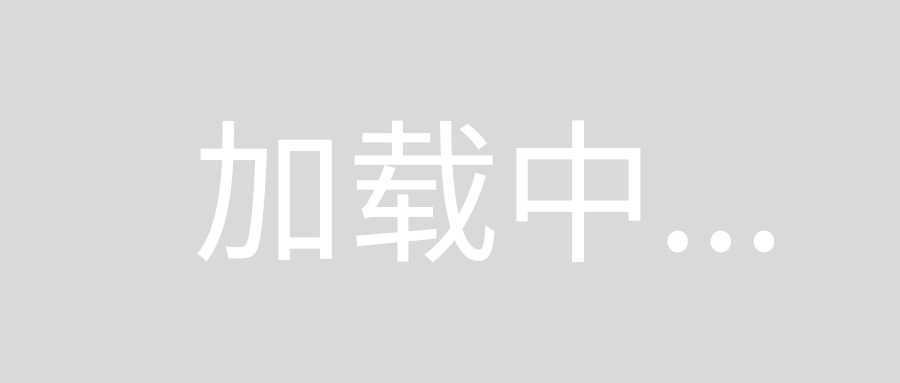
You can customize the appearance as per your requirement. Please check here for some custom appearances.