The company I work for has an iOS application that has two sliding menus: one on the left that is used for navigation, and one on the right that displays app wide contextual info and actions. The iOS application has an ActionBar like widget that slides with the main content pane when displaying either of the menus (meaning it is hidden). Due to the published guidelines for using a navigation drawer, we are looking at updating the UI in the Android version to conform (as closely as possible). With that in mind, my UI designer has asked for a couple of working demos that can be put in front of users to get feedback. She's asked for three samples:
one that has a stationary action bar across the top. Both menus would overlay the main content pane but not the action bar. This would follow the published guidelines.
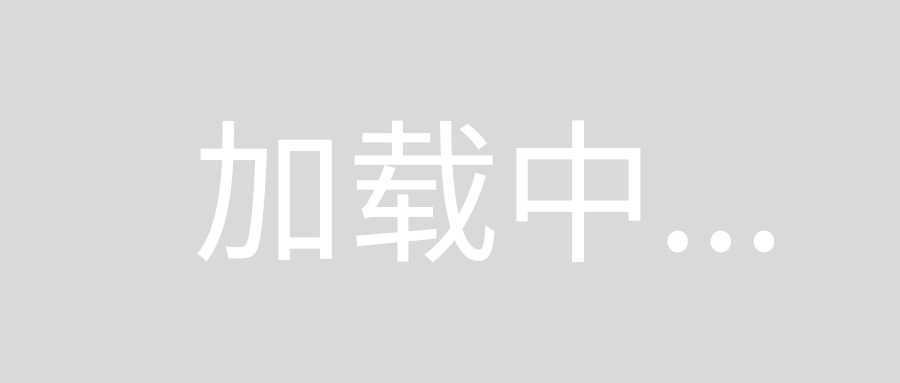
one that functions like the current Facebook app. The ActionBar (or equivalent) is considered part of the main content pane and would be covered/slide away when either drawer is opened.
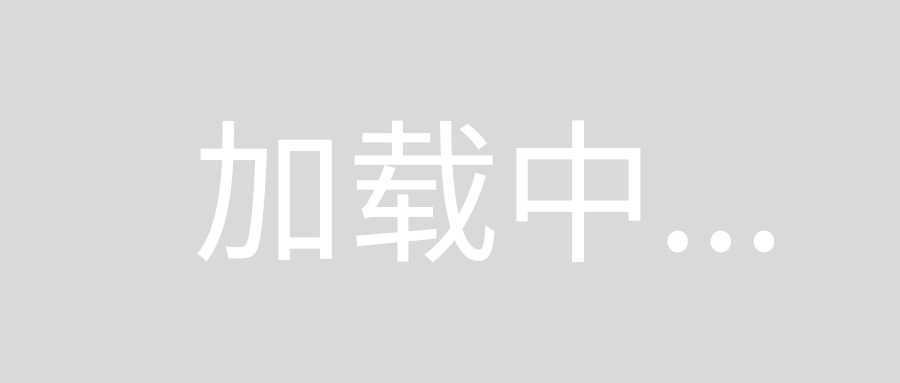
one that is a hybrid of the previous two: the left navigation menu should slide out under the action bar (following the guidelines). The right drawer would slide out over the action bar. This scenario is similar to the current Evernote app.
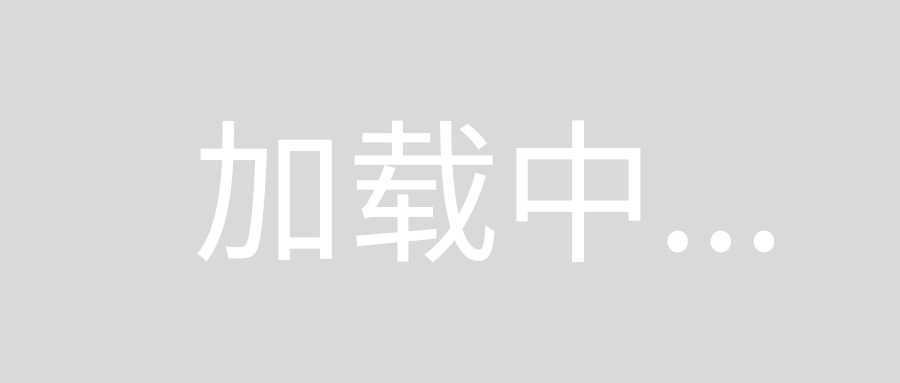
So far, I've been tinkering with SlidingMenu and have a functional demo for #1 and #2. Based on what I've read here, I should be able to accomplish #1 using the official Android navigation drawer. However, I haven't been able to get anything working for demo #3. It doesn't look like DrawerLayout can be made to work over top of the ActionBar so it's out.
Is it possible to use two drawers (using SlidingMenu): one that works with the ActionBar and one that doesn't? If not, are there any alternative solutions out there that will work or do I need to look at rolling my own solution?
Before I get into my answer I will just clarify the different types of drawers we have here
Option 1 is the standard Navigation Drawer where the content is brought on top of the main layout
option 2 is a 3rd party navigation drawer Sliding Menu which replicates exactly what the Facebook app does and has lots of options to customize
Option 3 is the SlidingPaneLayout with that looks to be a home made right navigation drawer forged from the android navigation drawer. You can tell its the slidingpane layout because you can swipe over anywhere in the content and the pane will move where as usually you have to swipe from the edge of the screen to get it to move.
it is possible to use 2 drawers to get the effect of #3, what I would do is use the SlidingPaneLayout
and then import the Sliding Menu
library and use it only got the right menu, that way you have your actionbar moving when you open the right drawer. It may not be exactly what you want where it is over the actionbar but its close.
So it turns out this is possible using SlidingMenu. Essentially I ended up creating two SliderMenu objects:
public class SlidingMenuEvernoteActivity extends SlidingFragmentActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.sliding_menu_main);
setBehindContentView(R.layout.sliding_menu_red);
setSlidingActionBarEnabled(false);
SlidingMenu leftMenu = getSlidingMenu();
leftMenu.setTouchModeAbove(SlidingMenu.TOUCHMODE_MARGIN);
leftMenu.setMode(SlidingMenu.LEFT);
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.sliding_menu_main, new ListFragment())
.commit();
SlidingMenu rightMenu = new SlidingMenu(this);
rightMenu.setTouchModeAbove(SlidingMenu.TOUCHMODE_MARGIN);
rightMenu.setMode(SlidingMenu.RIGHT);
rightMenu.setMenu(R.layout.sliding_menu_blue);
rightMenu.attachToActivity(this, SlidingMenu.SLIDING_WINDOW);
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.sliding_menu_blue, new ListFragment())
.commit();
}
}
Note: because the activity extends SlidingFragmentActivity, there is no need to create two SlidingMenu objects manually. The first is automatically created in a callback.
And here are the relevant layout files:
*sliding_menu_main.xml*
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent"
android:clickable="true"
android:id="@+id/sliding_menu_main">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:id="@+id/textView"
android:layout_gravity="center" />
</FrameLayout>
*sliding_menu_red.xml*
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/sliding_menu_red"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ff001c">
</LinearLayout>
*sliding_menu_blue.xml*
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/sliding_menu_blue"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#0028ff">
</LinearLayout>