I have created a list of Items using the following code:
<html ng-app="ionicApp">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no">
<title> Creators </title>
<link href="http://code.ionicframework.com/nightly/css/ionic.css" rel="stylesheet">
<script src="http://code.ionicframework.com/nightly/js/ionic.bundle.js"></script>
<script>
angular.module('ionicApp', ['ionic'])
.controller('MyCtrl', function($scope) {
$scope.items = [
{
"id": "1",
"name": "Steve Jobs"
},
{
"id": "2",
"name": "Bill Gates"
}
];
});
</script>
</head>
<body ng-controller="MyCtrl">
<ion-header-bar class="bar-positive">
<h1 class="title">Smart List</h1>
</ion-header-bar>
<ion-content>
<ion-list>
<ion-item ng-repeat="item in items" item="item">
{{ item.name }}
</ion-item>
</ion-list>
</ion-content>
</body>
</html>
Here is what I am getting:
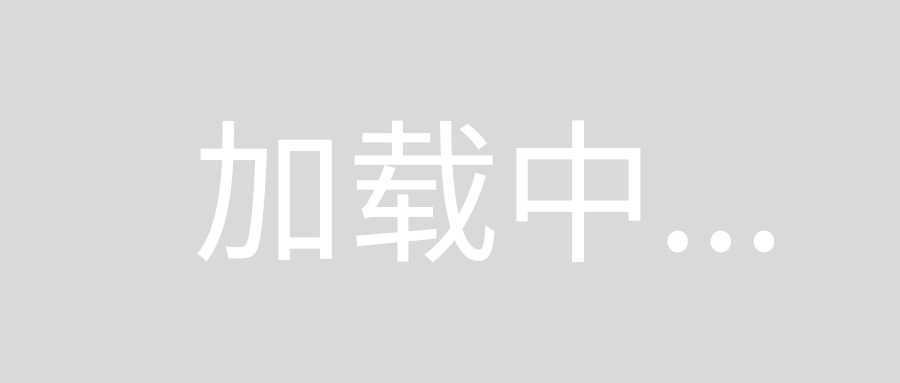
But now I would like to open another html
page where I can show detail
of tapped list item, like : Id and Name in case of above example
Here is the working plunker with some corrected changes ! Cheers to @Nimsesh Patel for creating this.
New html
<ion-modal-view>
<ion-header-bar>
<button ng-click="closeModal()">close</button>
<h1 class="title">My Modal title</h1>
</ion-header-bar>
<ion-content>
<h3>{{items[currentItem].id}}</h3>
<p>{{items[currentItem].name}}</p>
</ion-content>
</ion-modal-view>
In your controller
angular.module('ionicApp', ['ionic'])
.controller('MyCtrl', function($scope, $ionicModal) {
$scope.currentItem = 1;
$scope.items = [
{
"id": "1",
"name": "Steve Jobs"
},
{
"id": "2",
"name": "Bill Gates"
}
];
$scope.getdetails = function(id){
$scope.currentItem = id;
$scope.modal.show();
};
$ionicModal.fromTemplateUrl('detail.html', {
scope: $scope,
animation: 'slide-in-up'
}).then(function(modal) {
$scope.modal = modal;
});
$scope.closeModal = function() {
$scope.modal.hide();
};
});
In main html where ng-repeat is there
<body ng-controller="MyCtrl">
<ion-header-bar class="bar-positive">
<h1 class="title">Smart List</h1>
</ion-header-bar>
<ion-content>
<ion-list>
<ion-item ng-repeat="item in items track by $index" item="item" ng-click="getdetails($index)">
{{ item.name }}
</ion-item>
</ion-list>
</ion-content>
</body>
you can achieve this using stateProvider. Here is the example of chats list and chat detail In which when you click on particular chat, chat details will be shown in chat details page.
Here is app.js file which includes two controllers.
angular.module('app', ['ionic'])
.controller('ChatDetailsCtrl', function($scope, $stateParams, ChatService) {
$scope.chatId = $stateParams.chatId;
$scope.chat = ChatService.getChat($scope.chatId);
})
.controller('ChatsCtrl', function($scope, ChatService) {
$scope.chats = ChatService.getChats();
})
.service('ChatService', function() {
return {
chats: [
{
id: "1",
message: "Chat Message 1"
},
{
id: "2",
message: "Chat Message 2"
},
],
getChats: function() {
return this.chats;
},
getChat: function(chatId) {
for(i=0;i<this.chats.length;i++){
if(this.chats[i].id == chatId){
return this.chats[i];
}
}
}
}
})
.config(function($stateProvider, $urlRouterProvider) {
$stateProvider
.state('chats', {
url: "/chats",
templateUrl: "templates/chats.html",
controller: "ChatsCtrl"
})
.state('chatDetails', {
url: "/chats/:chatId",
templateUrl: "templates/chatDetails.html",
controller: "ChatDetailsCtrl"
});
$urlRouterProvider.otherwise("/chats");
})
Here is Html page's body code.
<body>
<ion-pane class="pane">
<ion-nav-bar class="bar-positive">
<ion-nav-back-button>
</ion-nav-back-button>
</ion-nav-bar>
<ion-nav-view></ion-nav-view>
<script id="templates/chats.html" type="text/ng-template">
<ion-view view-title="Chats">
<ion-content>
<ion-list>
<ion-item ng-repeat="chat in chats" href="#/chats/{{chat.id}}">
Chat {{ chat.id }}
</ion-item>
</ion-list>
</ion-content>
</ion-view>
</script>
<script id="templates/chatDetails.html" type="text/ng-template">
<ion-view view-title="Chat Details">
<ion-content>
<ion-item><b>Chat:</b> {{ chat.id }}</ion-item>
<ion-item><b>Message:</b> {{ chat.message }}</ion-item>
</ion-content>
</ion-view>
</script>
</ion-pane>
</body>
You can call a function on click of item like following
<ion-list>
<ion-item ng-repeat="item in items" ng-click="functionName()">
{{ item.name }}
</ion-item>
</ion-list>
In controller:
$scope.functionName = function(){
// open new html modal to show new screen using following
$ionicModal.fromTemplateUrl('templates/views/details.html',{
scope: $scope,
animation: 'none',
backdropClickToClose: false
}).then(function(modal){
$scope.modal= modal;
$scope.modal.show();
});
}
Using this you can show details of clicked item on new screen.