Imagine a view bag called
ViewBag.Modes
this contains the following:
Simple
Advanced
Manual
Complete
How can I access the viewbag by index like you would in an array?
e.g Simple is at index 0 then it would look like this
ViewBag.Modes[0]
I tried the above but it doesn't work so...How can I replicate this with viewbag or is there a workaround I can use?
This does the trick for me:
Controller:
public ActionResult Index()
{
var stringArray = new string[3] { "Manual", "Semi", "Auto"};
ViewBag.Collection = stringArray;
return View();
}
View:
@foreach(var str in ViewBag.Collection)
{
@Html.Raw(str); <br/>
}
@for (int i = 0; i <= 2; i++ )
{
@Html.Raw(ViewBag.Collection[i]) <br/>
}
Output:
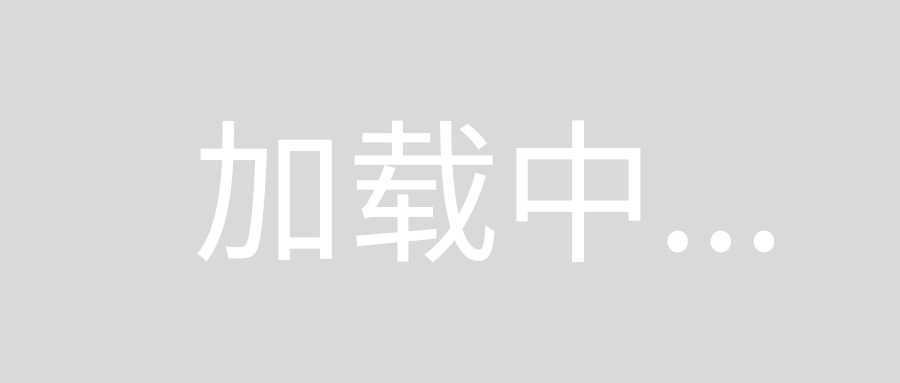
Sorry for not using your terms. I was scrachting this together from the top of my head.
ViewBag is a dynamic property, which complicates things a bit.
For the behavior you're looking for, you might want to use ViewData instead. ViewData is a dictionary, which means you can access the values of each index using Linq:
this.ViewData.Keys.ElementAt(0);
Using the ViewBag:
Controller
public ActionResult Index()
{
List<string> modes = new List<string>();
modes.Add("Simple");
modes.Add("Advanced");
modes.Add("Manual");
modes.Add("Complete");
ViewBag["Modes"] = modes;
return View();
}
View
<h1>List of Modes</h1>
@{foreach (var mode in ViewBag.Modes) {
<li>
@hobby
</li>
} }
----------------------------------------------------------------
Using the ViewData:
Controller
public ActionResult Index()
{
string[] Modes = {"Simple", "Advanced", "Manual", "Complete" };
ViewData["Modes"] = Modes;
return View();
}
**View
<h1>List of Modes</h1>
@foreach (string mode in ViewData["Modes"] as string[]) {
<li>
@mode
</li>
}
Thanks for the posts but shortly after writing this I came up with this solution using a model & list as below this could also be done using viewbag instead of model.
List<string> list = new List<string>();
foreach (Models.AppModeInfo blah in Model.theAppModes)
{
list.Add(blah.Name);
}
var AppModeText = "";
switch (item.AppModeId)
{
case 1:
AppModeText = list[0];
break;
case 2:
AppModeText = list[1];
break;
case 3:
AppModeText = list[2];
break;
case 4:
AppModeText = list[3];
break;
case 5:
AppModeText = list[4];
break;
}
In your controller:
string[] Modes = {"Simple", "Advanced", "Manual", "Complete" };
ViewData["Modes"] = Modes;
In your View:
<div>
@(((string[])ViewData["Modes"])[0])
</div>
<div>
@(((string[])ViewData["Modes"])[1])
</div>
<div>
@(((string[])ViewData["Modes"])[2])
</div>