I'll have more than one of these small boxes on my site, and each will start counting down at different times.
How can I decrease the numerical value of the timer per second, giving the simulation of a countdown timer?
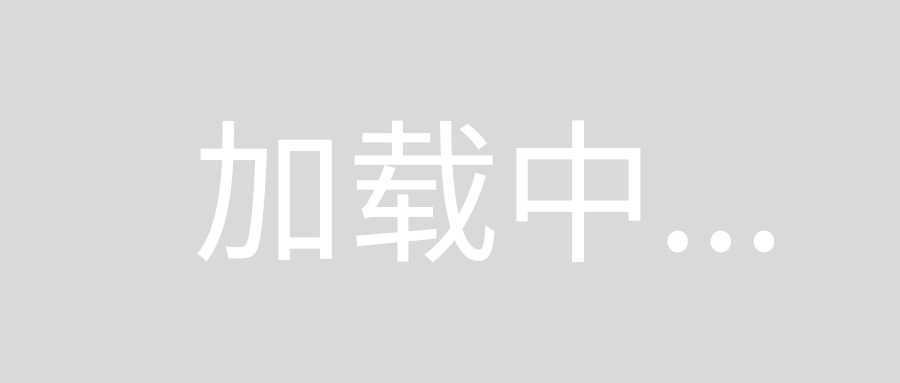
<p class="countdown">15</p>
Using this javascript it correctly countsdown, but every single auctionbox is affected. How would you suggest I isolate the timer to act on only one item?
<script>
var sec = 15
var timer = setInterval(function() {
$('.auctiondiv .countdown').text(sec--);
if (sec == -1) {
$('.auctiondiv .countdown').fadeOut('slow');
clearInterval(timer);
}
}, 1000);
</script>
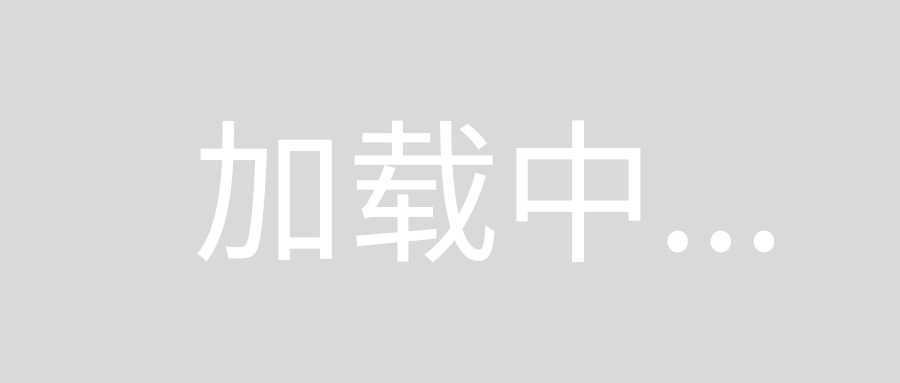
Try the following which will properly issue the count down for the selected values.
$(document).ready(function() {
// Function to update counters on all elements with class counter
var doUpdate = function() {
$('.countdown').each(function() {
var count = parseInt($(this).html());
if (count !== 0) {
$(this).html(count - 1);
}
});
};
// Schedule the update to happen once every second
setInterval(doUpdate, 1000);
});
JSFiddle Example
- http://jsfiddle.net/n24BP/
Note: This will run the count down sequence on every element which has the countdown
class. If you'd like to make it more restrictive to a single element you'll need to alter the selector from .countdown
to something more restrictive. The easiest way is to add an id and reference the item directly.
<p id='theTarget'>15</p>
The JavaScript is a little more complex here because you'll want the timer to eventually shut off since there's not much chance, or use, of element with a duplicate id being added
$(document).ready(function() {
var timer = setInterval(function() {
var count = parseInt($('#theTarget').html());
if (count !== 0) {
$('#theTarget').html(count - 1);
} else {
clearInterval(timer);
}
}, 1000);
});
JSFiddle Example
- http://jsfiddle.net/bSe9E/
HTML:
<p id="countdown">15</p>
JS:
var count = document.getElementById('countdown');
timeoutfn = function(){
count.innerHTML = parseInt(count.innerHTML) - 1;
setTimeout(timeoutfn, 1000);
};
setTimeout(timeoutfn, 1000);
Fiddle: http://jsfiddle.net/wwvEn/
You don't need jQuery for this (though it will help in setting the text).
setInterval
is what you want.
$.each(
$('.countdown'), function(el) {
setInterval( function() {
$(this).text($(this).text()*1 - 1);
}, 1000);
}
);
CountdownTimer is a reverse count down jQuery plugin for displaying countdown as per need with its different configuration options.
A minimal jQuery countdown clock plugin which allows you to count down to a target date time with the support of custom UTC Timezone offset.
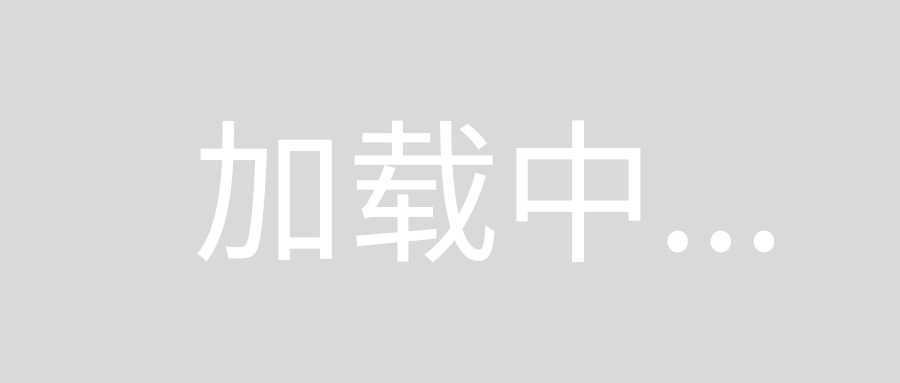
How to use it:
jQuery library and the jQuery countdown clock plugin in your web page.
<script type="text/javascript">
function SetTimer()
{
if(document.SetDefaultTimer != true){
SetDefaultTimeOut();
}
}
function SetDefaultTimeOut()
{
//document.SetDefaultTimer = true;
setTimeout(function () {
StartTimeOut();
$("#videos-container4").css("display", "block");
}, $("#HdnSetDefaultTime").val());
}
function SetTimerByContinue()
{
document.SetDefaultTimer = true;
setTimeout(function () {
StartTimeOut();
$("#videos-container4").css("display", "block");
}, $("#HdnSMDIdleNotificationRepeatTime").val());
//}, 600000);
}
function ResetTimer()
{
$("#videos-container4").css("display", "none");
clearInterval(cleanTimeOutInterval);
//SetDefaultTimeOut();
SetTimerByContinue();
}
$('#ClickContinue').click(function () {
ResetTimer();
});
var cleanTimeOutInterval;
function StartTimeOut() {
debugger
var timer2 = $("#HdnTimerCountDownStart").val() + ":00";
$('.countdown').html($("#HdnTimerCountDownStart").val() + "mins" + ":00" + "secs");
cleanTimeOutInterval = setInterval(function () {
var timer = timer2.split(':');
//by parsing integer, I avoid all extra string processing
var minutes = parseInt(timer[0], 10);
var seconds = parseInt(timer[1], 10);
--seconds;
minutes = (seconds < 0) ? --minutes : minutes;
if (minutes < 0) clearInterval(interval);
seconds = (seconds < 0) ? 59 : seconds;
seconds = (seconds < 10) ? '0' + seconds : seconds;
//minutes = (minutes < 10) ? minutes : minutes;
//$('.countdown').html(minutes + ':' + seconds);
if(minutes == "0"){
$('.countdown').html(seconds + 'secs');
} else {
$('.countdown').html(minutes + 'mins ' + seconds + 'secs');
}
timer2 = minutes + ':' + seconds;
if (timer2 == "0:00") {
//Your action below
}
}, 1000);
}
</script>
Create the Html for a countdown clock using unordered list.
<div id="videos-container4" class="container-fluid main-timer">
<a href="javascript:void(0);" id="btnAnchor" onclick="SetTimer()" runat="server">Timer</a>
<input type="hidden" id="HdnSetDefaultTime" value="0" />
<input type="hidden" id="HdnSMDIdleNotificationRepeatTime" value="0" />
<input type="hidden" id="HdnTimerCountDownStart" value="0" />
<span>Your Time will be end in </span>
<br />
<span style="color: red;">
<span class="countdown"></span>
</span>
<!-- <span>minutes</span> -->
<div style="margin-top: 10px;">
<a id="ClickContinue" class="btn-continue">Continue</a>
</div>
</div>
Add the following CSS snippets to style the countdown clock.
<style type="text/css">
.main-timer {
margin: 10px 15px;
display: none;
float: right;
border: 1px solid #fd0000;
border-radius: 15px;
padding-top: 8px;
height: 100px;
width: 17%;
text-align: center;
background: #fff;
box-shadow: 0 4px 16px -4px #888;
position: relative;
}
.btn-continue {
cursor: pointer;
border: 1px solid #ff0707;
padding: 4px;
margin-right: 6px;
color: green;
text-decoration: none;
}
.btn-continue:hover {
border: 2px solid #ff0707;
color: green;
text-decoration: none;
}
</style>
var countDown = function() {
var ct = 15;
var $elem = $(this);
var display = function() {
$elem.text(ct--);
}
var iv = setInterval(function() {
display();
if (ct === 0) {
clearInterval(iv);
}
}, 1000);
display();
};
$("#countdown").each(countDown);
Try this in your inspector to get the idea how a countdown timer should work:
var count = 15; setInterval("if(count>0)console.log(count--)", 1000)
And here is full code for your case(no jquery)
var counter = document.getElementsByClassName('countdown')[0],
count = parseInt(counter);
setInterval("if(count>0)counter.innerHTML(count--)", 1000)