可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
In my code, I have an AlertDialog
and a TextView
. I'd like display this TextView
in my AlertDialog
but I don't know how to do it. I don't know how to add the View
in a AlertDialog
.
I could show my code but I don't think it would be usefull.
Thank's
EDIT:
Thank's for all your answers. I just did a test and it works perfectly.
Here is my working code:
package com.example.testalertdialog;
import android.app.Activity;
import android.app.AlertDialog;
import android.os.Bundle;
import android.widget.LinearLayout;
import android.widget.TextView;
public class MainActivity extends Activity {
LinearLayout layout;
AlertDialog ad;
TextView tv1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
layout = new LinearLayout(this);
ad = new AlertDialog.Builder(this).create();
tv1 = new TextView(this);
setContentView(layout);
tv1.setText("Test");
ad.setView(tv1);
ad.show();
}
}
Edit2: But why doesn't this code work ?
package com.example.testalertdialog;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.TextView;
@SuppressLint("HandlerLeak")
public class MainActivity extends Activity implements OnClickListener{
LinearLayout layout;
AlertDialog ad;
TextView tv1;
Button b1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
layout = new LinearLayout(this);
tv1 = new TextView(this);
b1 = new Button(this);
b1.setOnClickListener(this);
layout.addView(b1);
ad = new AlertDialog.Builder(this).create();
setContentView(layout);
tv1.setText("Test");
}
@Override
public void onClick(View v) {
if (v == b1) {
ad.setMessage("Chargement");
ad.show();
ad.setView(tv1);
}
}
}
回答1:
AlertDialog.Builder alert = new AlertDialog.Builder(this);
alert.setTitle("Title");
alert.setMessage("Message");
// Create TextView
final TextView input = new TextView (this);
alert.setView(input);
alert.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
input.setText("hi");
// Do something with value!
}
});
alert.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
// Canceled.
}
});
alert.show();
回答2:
Here in the part custom layout - you can create a custom layout for your alertDialog, not only in textview, but anything.
Link to simplify: here is my code from my game
//create builder
AlertDialog.Builder builder = new AlertDialog.Builder(GWGPlay.this);
//inflate layout from xml. you must create an xml layout file in res/layout first
LayoutInflater inflater = GWGPlay.this.getLayoutInflater();
View layout = inflater.inflate(R.layout.guesskeyword /*my layout here*/, null);
builder.setView(layout);
//set 2 main buttons
builder.setPositiveButton("Answer", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
endGame(true);
}
});
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
builder.show();
回答3:
A view can be added to a AlertDialog
using the setView
method.
Example:
AlertDialog dialog = new AlertDialog.Builder(this).create();
dialog.setView(your_textview);
dialog.show();
回答4:
Another example of using the setView
method:
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
LayoutInflater inflater = getActivity().getLayoutInflater();
builder.setView(inflater.inflate(R.layout.name_dialog, null));
builder.create().show();
回答5:
Try this sample snippet code,
Dialog dialog = new Dialog(context);
dialog.setContentView(R.layout.custom);
dialog.setTitle("Title...");
// set the custom dialog components - text, image and button
TextView text = (TextView) dialog.findViewById(R.id.text);
text.setText("Android custom dialog example!");
ImageView image = (ImageView) dialog.findViewById(R.id.image);
image.setImageResource(R.drawable.ic_launcher);
Button dialogButton = (Button) dialog.findViewById(R.id.dialogButtonOK);
// if button is clicked, close the custom dialog
dialogButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
dialog.dismiss();
}
});
dialog.show();
回答6:
In drawable create a file name custom_window_dialog_frame.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<shape android:shape="rectangle"> <!-- this shape is used as shadow -->
<padding android:bottom="3dp"
android:left="3dp"
android:right="3dp"
android:top="3dp"/>
<solid android:color="#44000000"/>
<!-- <corners android:radius="5dp"/> -->
</shape>
</item>
</layer-list>
and then go to values folder open styles.xml and paste code below
<style name="CustomDialog" parent="@android:style/Theme.Dialog">
<item name="android:backgroundDimEnabled">true</item>
<item name="android:windowBackground">@drawable/custom_window_dialog_frame</item>
<item name="android:windowNoTitle">true</item>
</style>
Create a layout as you want to show. In my case i have done like in file dialog_layout_pro.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#dedede"
>
<TextView
android:id="@+id/tv_dialog"
android:layout_margin="30dip"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:gravity="center"
android:textColor="#444444"
android:text="Please wait..."
android:textSize="30sp" />
</RelativeLayout>
In MyTestClass.java Class
Dialog cusDialog;
cusDialog = new Dialog(MyTestClass.this, R.style.CustomDialog);
cusDialog.setContentView(R.layout.dialog_layout_pro);
cusDialog.setCancelable(false);
cusDialog.show();
}
Custom Dialog will look like in image below. enjoy coding :)
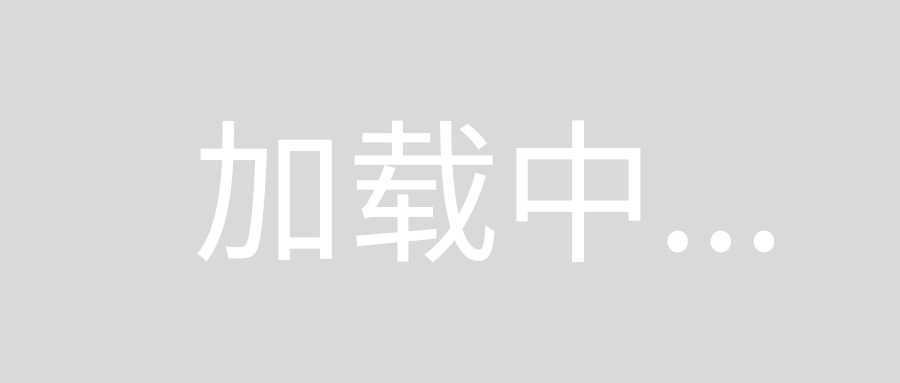
回答7:
try this
http://www.mkyong.com/android/android-custom-dialog-example/
here you need to set your own layout .
like this
final Dialog dialog = new Dialog(context);
dialog.setContentView(R.layout.custom);
it will give you textview
inside dialog.
try this
//layout for textview
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#B7B7B8"
android:text="Hello"/>
</LinearLayout>
code:-
AlertDialog dlg = new AlertDialog.Builder(context)
.setView(R.layout.textview)
.setTitle("Message")
.setMessage(msg)
.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
//retval = 0;
Toast.makeText(context,
msg, Toast.LENGTH_SHORT).show();
}
}).create();
dlg.show();