I trying to place an AdMob ad at the top of the screen (above) the Android Actionbar.
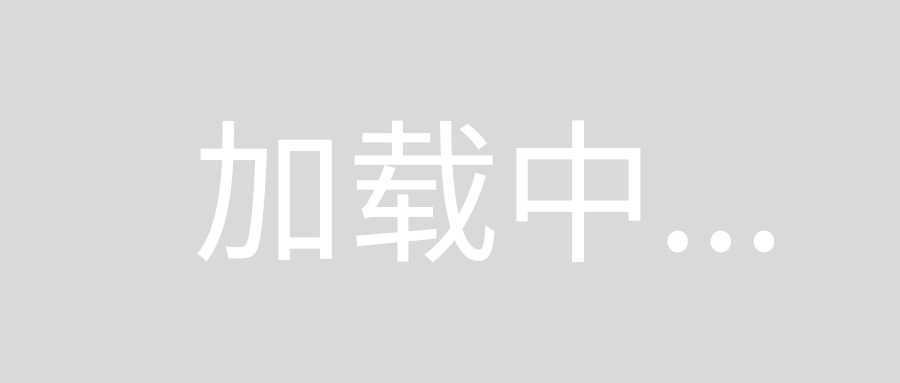
- yellow = ActionBar
- red = content
- green = AdMob
A similar question I found is to place an ad at the bottom of a screen with Actionbar showing up. But so far I couldn't figure out how to place it at the top of the screen.
I tried it this way:
private AdView adView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// main view setup
setContentView(R.layout.main);
this.getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
// actionbar setup
ActionBar actionBar = getActionBar();
actionBar.setTitle("Search");
actionBar.setDisplayHomeAsUpEnabled(true);
final LinearLayout content = (LinearLayout) findViewById(android.R.id.content).getParent();
// Create the adView
adView = new AdView(this, AdSize.BANNER, "ID");
// Lookup your LinearLayout assuming it’s been given
// the attribute android:id="@+id/mainLayout"
// Add the adView to it
FrameLayout.LayoutParams parm = (FrameLayout.LayoutParams) content.getLayoutParams();
parm.gravity = Gravity.TOP;
content.addView(adView);
// Initiate a generic request to load it with an ad
AdRequest adRequest = new AdRequest();
adRequest.addTestDevice("TEST_DEVICE_ID");
adView.loadAd(adRequest);
}
Below you can see a part of the hierarchy viewer of my app. The view "id/main" is my main layout.
As you can see on the picture the ActionBar has a id called "id/action_bar_container". In my code above I managed to access the FrameLayout "content" but I wasn't able to access the action_bar_container view. Because they are on the same hierarchy level (I think) it should be possible to access that ActionBar view somehow.
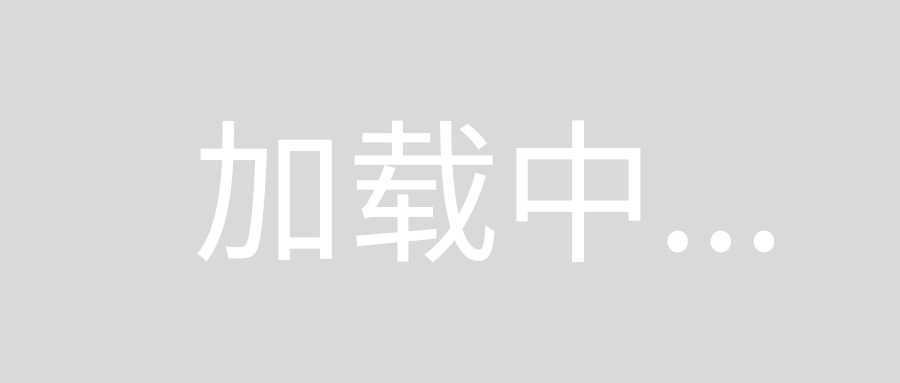
But it didn't work:
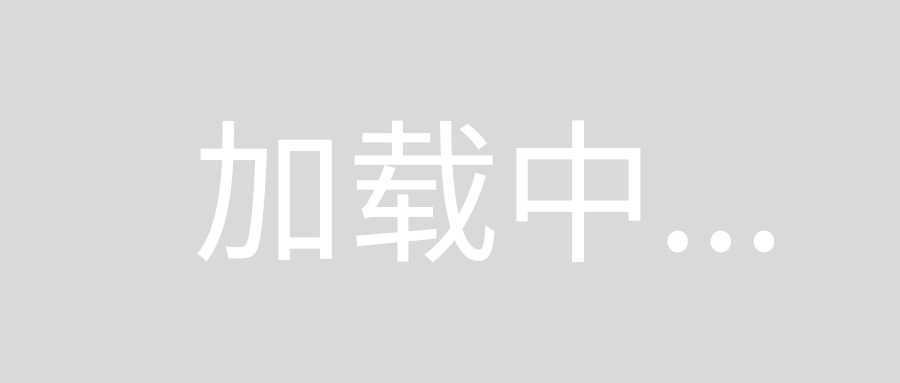
Any idea how to achieve that or a link how it could work or an official statement that it doesn't work.
You can usegetRootView()
on any view orgetWindow().getDecorView()
to get thePhoneDecorView
. It has one child - a verticalLinearLayout
.
Add your layout there as the first child.
Note that this is a bad, bad idea. From a user experience point of view, this is a horrific strike against all Design Guidelines. The action bar must be at the top for a multitude of design reasons.
Second, this might break on OEM-themed devices.
Third, what about tablets in landscape, will you really have such a huge bar at the top?
In conclusion, yes, it's possible but it's a horrible idea. Don't do it.
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import com.actionbarsherlock.app.ActionBar;
import com.actionbarsherlock.app.SherlockActivity;
public class MainActivity extends SherlockActivity {
private ActionBar bar;
@Override
protected void onCreate(Bundle savedInstanceState) {
setTheme(R.style.Theme_Sherlock);
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bar = getSupportActionBar();
LayoutInflater inflater = (LayoutInflater)getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View customView = (View)inflater.inflate(R.layout.custom_view, null); //<-- put your ad instead of this
bar.setDisplayOptions(
ActionBar.DISPLAY_SHOW_CUSTOM,
ActionBar.DISPLAY_SHOW_CUSTOM | ActionBar.DISPLAY_SHOW_HOME
| ActionBar.DISPLAY_SHOW_TITLE);
bar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
bar.setCustomView(customView, new ActionBar.LayoutParams(
ViewGroup.LayoutParams.MATCH_PARENT,
ViewGroup.LayoutParams.MATCH_PARENT));
}
@Override
public boolean onCreateOptionsMenu(com.actionbarsherlock.view.Menu menu) {
getSupportMenuInflater().inflate(R.menu.test_menu, menu);
return super.onCreateOptionsMenu(menu);
}
}
As far as I know, once you choose to work with an ActionBar, it is added in a higher level (of the UI hierarchy) than your layout.
Meaning you have no control as to where it is placed, and what is placed above it.
Your layout starts below the action bar and ends at the bottom of the screen.
In my opinion, if you do find a way to do what you want, it will be very patchy.
You say you have the content frame layout reference. can you get its parent view? cast it to LinearLayout then add your view on the first position.
http://developer.android.com/reference/android/view/View.html#getParent%28%29
This hardly seems worth the bounty, but a previous question answers this:
Getting admob above actionbarsherlock
The trick is to switch over from using the google actionbar to actionbarsherlock library. This is likely a good idea for your app anyway, as it allows you to support the actionbar on older builds of android.
The user claims to have gotten that to work.