I've got this markup
<div contentEditable="true">
Some other editable content
<div class="field" contentEditable="false">
<span class="label">This is the label</span>
<span class="value" contentEditable="true">This is where the caret is</span>
</div>
<!-- This is where I want the Caret -->
</div>
The caret is currently in the .field
span.
I need to move it back out after the .field
in the parent contentEditable
.
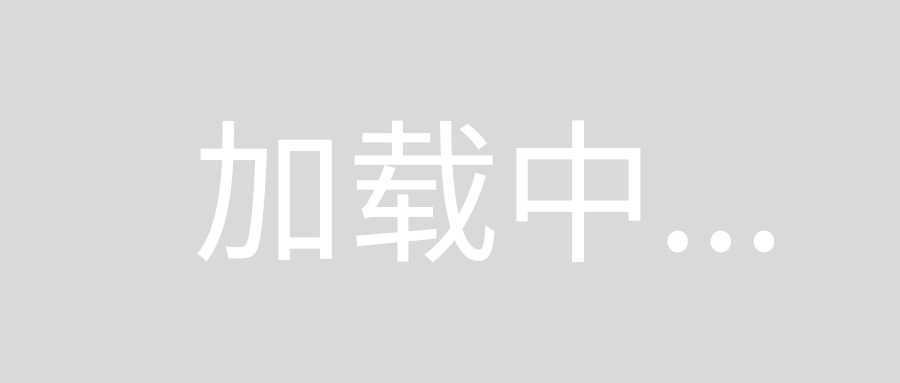
How can this be accomplished via javascript (with the use of jQuery if needed) ?
It will be trying to trigger it on a keydown event when the caret is in the .value span as shown.
An update to the previous answer.
http://jsfiddle.net/GLzKy/4/
placeCaretAtEnd($(this).parent().nextAll('.field').children('value'));
UPDATE
Updated answer with correct answer in comments
For moving directly after the current field, you might want to use range.setStartAfter and range.setEndAfter: http://jsfiddle.net/GLzKy/5
I am assuming that you want to go to this next section. To make it clear, I added a text "Here!" in a demo to show you that it does goes to the end.
In the below demo, press Enter key from the .field .value
to go to the end.
DEMO: http://jsfiddle.net/GLzKy/1/
Below is the function from https://stackoverflow.com/a/4238971/297641 which actually does all the work.
$('.field .value').keydown(function (e) {
if (e.which == 13) { //Enter Key
e.preventDefault();
placeCaretAtEnd($(this).closest('.parent')[0]);
}
});
/**
This below function is from https://stackoverflow.com/a/4238971/297641
All credits goes to the original author.
*/
function placeCaretAtEnd(el) {
el.focus();
if (typeof window.getSelection != "undefined"
&& typeof document.createRange != "undefined") {
var range = document.createRange();
range.selectNodeContents(el);
range.collapse(false);
var sel = window.getSelection();
sel.removeAllRanges();
sel.addRange(range);
} else if (typeof document.body.createTextRange != "undefined") {
var textRange = document.body.createTextRange();
textRange.moveToElementText(el);
textRange.collapse(false);
textRange.select();
}
}
Tested in FF, IE8 and Chrome
Reference: https://stackoverflow.com/a/4238971/297641