I don't suppose someone could guide me on the proper way to dynamically size a UITextView while still using Auto-Layout? Using Swift, that is.
I've tried programmatically adjusting the bottom constraints in attempt to get the UITextView to hug the content. But I'm not sure how to get the height of the content of the UITextView?
I'm attempting to load data into UITextView fields, then, dynamically adjust them to look neat.
@IBOutlet weak var serviceDescriptionTextViewBottomGuide: NSLayoutConstraint!
override func viewDidLoad() {
self.activityName.text = serviceCodes[selectionValue]
self.serviceDescriptionTextView.text = serviceDescription[selectionValue]
self.whenToUseDescriptionTextView.text = whenToUseCode[selectionValue]
self.serviceDescriptionTextViewBottomGuide?.constant += x
}
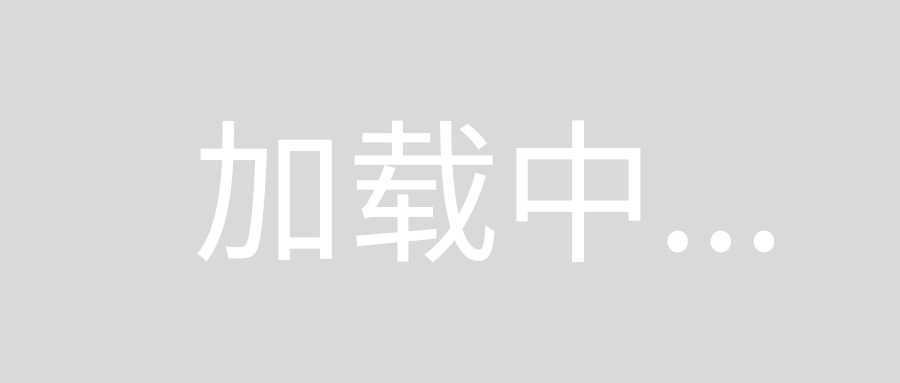
Disable scrolling and do not put any other constraint that can dictate the height of UITextView.
This is right but I also had to write:
override func viewDidLoad() {
super.viewDidLoad()
tableView.estimatedRowHeight = 44.0 // Replace with your actual estimation
// Automatic dimensions to tell the table view to use dynamic height
tableView.rowHeight = UITableViewAutomaticDimension
}
See more:
https://pontifex.azurewebsites.net/self-sizing-uitableviewcell-with-uitextview-in-ios-8/
You can create an IBOutlet
for any constraint you want to vary programmatically. Then vary the constraint via its NSLayoutConstraint's constant
property in the code via its outletted reference. That works pretty well. You can vary width, hight and position that way. You cannot modify NSLayoutConstraint's multiplier
property though (it's readonly). But that doesn't matter, because what you could accomplish via definining a constraint's multiplier property in Interface Builder (to get a proportional value), you can alternatively accomplish by performing the math in your code and modifying the constant
property.
An alternative approach is, you can disable the resizing mask translation (e.g.the UIView
translatesAutoresizingMaskIntoConstraints
property set to nil) and create your constraints from scratch programmatically, for which the recommended approach (easier to use) is called visual format. But creating constraints totally programmatically is more work than using Interface Builder Autolayout to create a rough draft of your views, and then tweaking select constraints programmatically after the fact, at least in typical simple cases.