可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
One of my old apps is not working with iOS8. When I start the app up, and try to tap on the screen anywhere, I get this message in my console:
unexpected nil window in _UIApplicationHandleEventFromQueueEvent,
_windowServerHitTestWindow: <UIWindow: 0x7fe4d3e52660; frame = (0 0; 320 568);
opaque = NO; autoresize = RM+BM; gestureRecognizers = <NSArray: 0x7fe4d3e2c450>;
layer = <UIWindowLayer: 0x7fe4d3e86a10>>
I'm using an old style MainWindow.xib. In the MainWindow.xib is my Window object, as well as a UINavigationController which has its first View Controller defined within as well. The image below is showing the Outlets connected to the App Delegate.
The white "view" in the screenshot below is the UIWindow. The view on the right is the UINavigationController (nav bar hidden) with the first ViewController defined inside it.
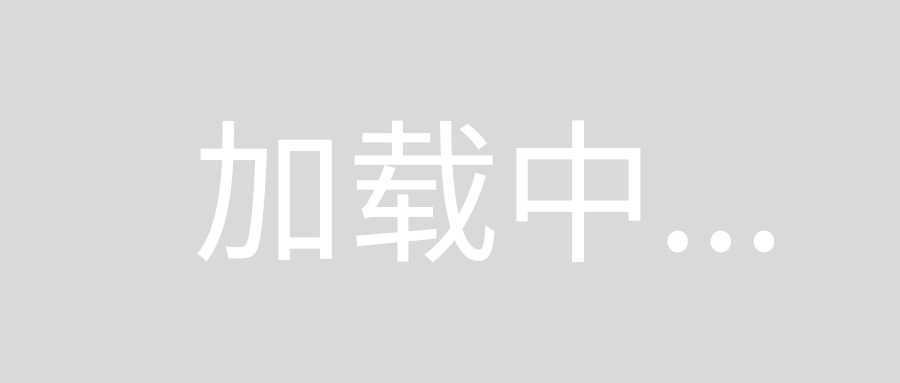
How do I fix this without recreating the entire app from scratch with a new project?
EDIT: I just discovered there is a TINY strip wherein my buttons will actually receive their taps/clicks.
I've also noticed that my self.view.window is nil. How do I make sure that is set?
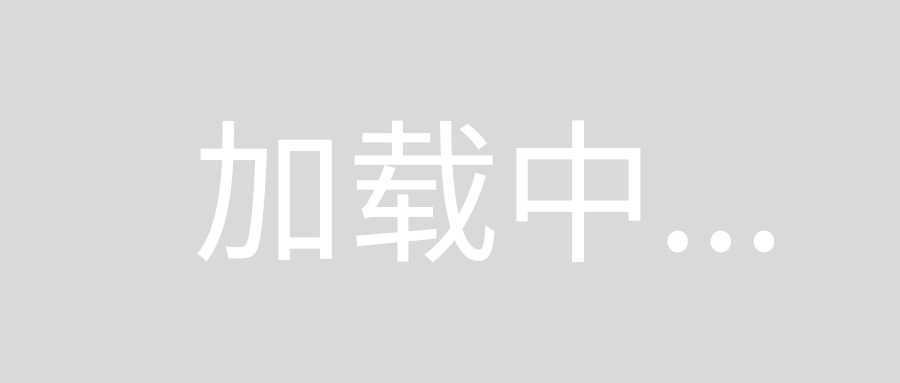
回答1:
Additional to B H answer. Also look this answer.
Got this issue when launching my landscape only app from portrait orientation (also the app shouldn't be presented in recently opened apps list, which can be seen by pressing Home button twice. Perhaps, iOS somehow caches the orientation and window size).
My code was
self.window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
[self.window makeKeyAndVisible];
I set W+H
autoresizing mask for window, it didn't help me, because the window don't being resized on rotation, but the transform
matrix is changed.
The solution is simple
self.window = [UIWindow new];
[self.window makeKeyAndVisible];
self.window.frame = [[UIScreen mainScreen] bounds];
So the frame is set AFTER the window get its orientation from root view controller.
回答2:
You don't have to add any code to fix this.
Open the main xib file and find "Window" from the left side and enable Visible at Launch
and Full Screen at launch
.
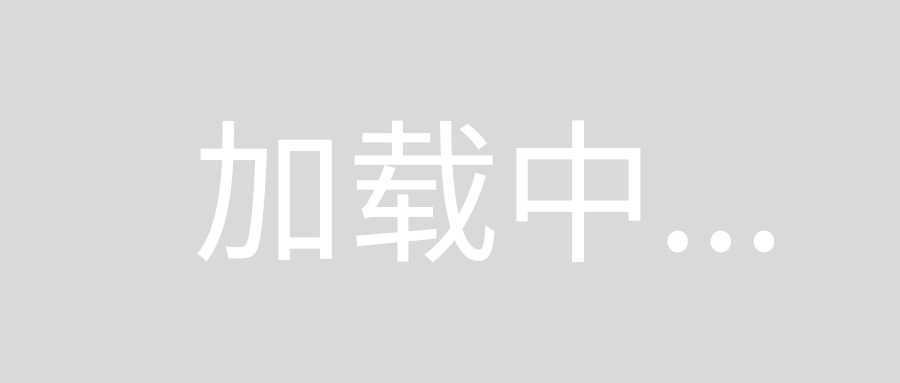
回答3:
Check out your Window nib file and make sure it is taking up the full screen. I had a similar issue on my app where touch events weren't being registered on a strip on the right side. Once I set my MainWindow.xib to take up the Full Screen, I had no more errors and touch events were working again. My app was also being displayed in Landscape but my MainWindow.xib had portrait dimensions.
回答4:
Sometimes it's just simple setting that's missing some value: Click on Project(whatever you named your project) name item then make sure General tab is selected and scroll to the bottom. Look for App icons and Launch Images make sure there is some value in the Launch Screen File field, this should be either Main or LaunchScreen
回答5:
In case somebody finds this useful:
In my case, I had used
self.window = [[UIWindow alloc] init];
instead of
self.window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
and that was the reason for the problem.
回答6:
I was getting this error on an old app that did not have the Default-568h@2x.png launch screen. When the taller iPhones were first introduced, this was the signal to iOS that the app could handle the new geometry. Otherwise, it was displayed in a 320x480 window. Funny, I did not even notice the app was not using the full screen.
回答7:
I wasn't able to test efpies
solution, but the way I fixed it in my app was to replace the MainWindow.xib with a Storyboard.
回答8:
We had the same issue, and we tried the proposed solutions in this thread without success.
What ended up working for us, was to re-write the logic to be pure programmatically instead of using xib's. After this we don't see the 'unexpected nil window' error and the view is getting the hit all over the screen.
回答9:
In case this helps anyone else that stumbles here, I also got this error when I had the UIScreen.mainScreen().bounds
size flipped when setting the window's frame.
回答10:
I am also upgrading my old project to iOS 7 and iOS 8.
Actually, I don't use MainWindow.xib, but creating window
manually in application:didFinishLaunchingWithOptions:
.
But i have same unexpected nil window in _UIApplicationHandleEventFromQueueEvent
error after launch.
So, in my case, problem was solved by changing deployment target to 6.0
and replacing code in main.m
:
Old main.m:
int main(int argc, char *argv[])
{
NSAutoreleasePool *pool = [[NSAutoreleasePool alloc] init];
int retVal = UIApplicationMain(argc, argv, nil, nil);
[pool release];
return retVal;
}
New main.m:
int main(int argc, char *argv[])
{
@autoreleasepool {
return UIApplicationMain(argc, argv, nil, NSStringFromClass([YourAppDelegate class]));
}
}
回答11:
Using the new ios 8 'nativeBounds' UIScreen property instead of 'bounds' when creating the window fixed the issue for me. No other changes required.
self.window = [[[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] nativeBounds]] autorelease];
To support previous version too, I do a runtime check with the version:
if([[[UIDevice currentDevice] systemVersion] floatValue] >= 8.0)