I'm using Swashbuckle to enable the use of swagger and swagger-ui in my WebApi project.
In the following image you can see two of my controllers shown in the swagger-ui page. These are named as they are in the C# code, however I was wondering if there was a way to change what is shown here?
This is mainly because as you can see ManagementDashboardWidget
is not a user friendly name, so I want to change it to be user friendly.
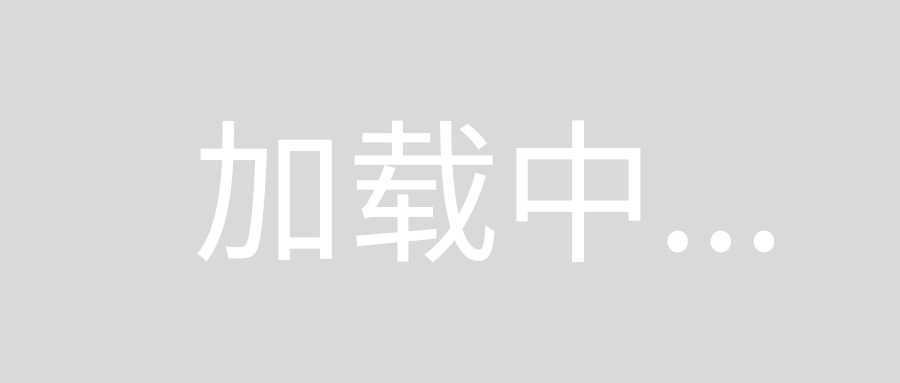
You can use tags for that. By default Swashbuckle adds a tag with the name of the controller to every operation. You can override that with the SwaggerOperationAttribute
. For instance, the next line replaces the default tag, Values, with the tag Test:
public class ValuesController : ApiController
{
[SwaggerOperation(Tags = new[] { "Test" })]
public IHttpActionResult Get()
{
// ...
}
}
The Get
operation will now be put in the group Test
.
If you want the operation to appear in multiple groups you can add more tags. For instance:
[SwaggerOperation(Tags = new[] { "Test", "Release1" })]
will put the Get
operation in the groups Test
and Release1
.
I tried using venerik's answer, but it still kept the original controller name in the UI along with the new tag that you specify. I also didn't like that you had to add an attribute to every function, so I came up with a solution where you only have to add an attribute to the controller. There are two steps:
Add DisplayNameAttribute
on the controller:
[DisplayName("Your New Tag")]
public class YourController : ApiController
{
// ...
}
Then in the Swagger configuration, you can override the base functionality using the GroupActionsBy
function to pull the name that you specified in that attribute:
GlobalConfiguration.Configuration
.EnableSwagger(c => {
c.GroupActionsBy(apiDesc => {
var attr = apiDesc
.GetControllerAndActionAttributes<DisplayNameAttribute>()
.FirstOrDefault();
// use controller name if the attribute isn't specified
return attr?.DisplayName ?? apiDesc.ControllerName();
});
})
.EnableSwaggerUi(c => {
// your UI config here
});